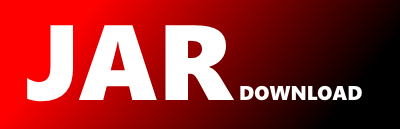
com.paypal.base.ReflectionUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
package com.paypal.base;
import java.lang.reflect.AccessibleObject;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Map.Entry;
public final class ReflectionUtil {
private ReflectionUtil() {}
public static Map decodeResponseObject(Object responseType,
String prefix) {
Map returnMap = new HashMap();
Map rMap = ReflectionUtil.generateMapFromResponse(
responseType, "");
if (rMap != null && rMap.size() > 0) {
for (Entry entry : rMap.entrySet()) {
returnMap.put(entry.getKey(), entry.toString());
}
}
return returnMap;
}
public static Map generateMapFromResponse(
Object responseType, String prefix) {
if (responseType == null) {
return null;
}
Map responseMap = new HashMap();
// To check return types
Map returnMap;
Object returnObject;
try {
Class klazz = responseType.getClass();
Method[] methods = klazz.getMethods();
Package packageName;
String propertyName;
AccessibleObject.setAccessible(methods, true);
for (Method m : methods) {
if (m.getName().startsWith("get")
&& !m.getName().equalsIgnoreCase("getClass")) {
packageName = m.getReturnType().getPackage();
try {
if (prefix != null && prefix.length() != 0) {
propertyName = prefix + "."
+ m.getName().substring(3, 4).toLowerCase(Locale.US)
+ m.getName().substring(4);
} else {
propertyName = m.getName().substring(3, 4)
.toLowerCase(Locale.US)
+ m.getName().substring(4);
}
if (packageName != null) {
if (!packageName.getName().contains("com.paypal")) {
returnObject = m.invoke(responseType, null);
if (returnObject != null
&& List.class.isAssignableFrom(m
.getReturnType())) {
List listObj = (List) returnObject;
int i = 0;
for (Object o : listObj) {
if (o.getClass().getPackage().getName()
.contains("com.paypal")) {
responseMap
.putAll(generateMapFromResponse(
o, propertyName
+ "(" + i
+ ")"));
} else {
responseMap.put(propertyName + "("
+ i + ")", o);
}
i++;
}
} else if (returnObject != null) {
if (responseType.getClass().getSimpleName()
.equalsIgnoreCase("ErrorParameter")
&& propertyName.endsWith("value")) {
propertyName = propertyName.substring(
0,
propertyName.lastIndexOf('.'));
}
responseMap.put(propertyName, returnObject);
}
} else {
returnObject = m.invoke(responseType, null);
if (returnObject != null
&& m.getReturnType().isEnum()) {
responseMap
.put(propertyName,
returnObject
.getClass()
.getMethod(
"getValue",
null)
.invoke(returnObject,
null));
} else if (returnObject != null) {
returnMap = generateMapFromResponse(
returnObject, propertyName);
if (returnMap != null
&& returnMap.size() > 0) {
responseMap.putAll(returnMap);
}
}
}
} else {
responseMap.put(propertyName,
m.invoke(klazz.newInstance(), null));
}
} catch (IllegalAccessException e) {
} catch (IllegalArgumentException e) {
} catch (InvocationTargetException e) {
} catch (InstantiationException e) {
}
}
}
} catch (Exception e) {
return null;
}
return responseMap;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy