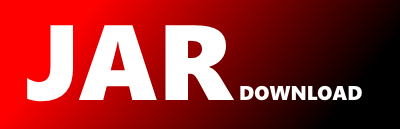
com.paypal.base.rest.AccessToken Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.base.rest;
public class AccessToken {
private String accessToken;
private long expires = 0;
/**
* Specifies how long this token can be used for placing API calls. The
* remaining lifetime is given in seconds.
*
* @return remaining lifetime of this access token in seconds
*/
public long expiresIn() {
return expires - new java.util.Date().getTime() / 1000;
}
@java.lang.SuppressWarnings("all")
public String getAccessToken() {
return this.accessToken;
}
@java.lang.SuppressWarnings("all")
public long getExpires() {
return this.expires;
}
@java.beans.ConstructorProperties({"accessToken", "expires"})
@java.lang.SuppressWarnings("all")
public AccessToken(final String accessToken, final long expires) {
this.accessToken = accessToken;
this.expires = expires;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof AccessToken)) return false;
final AccessToken other = (AccessToken) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$accessToken = this.getAccessToken();
final java.lang.Object other$accessToken = other.getAccessToken();
if (this$accessToken == null ? other$accessToken != null : !this$accessToken.equals(other$accessToken)) return false;
if (this.getExpires() != other.getExpires()) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof AccessToken;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $accessToken = this.getAccessToken();
result = result * PRIME + ($accessToken == null ? 43 : $accessToken.hashCode());
final long $expires = this.getExpires();
result = result * PRIME + (int) ($expires >>> 32 ^ $expires);
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy