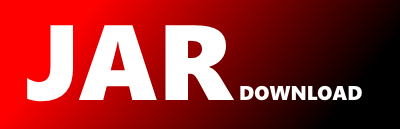
com.paypal.api.payments.Agreement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Tue Jan 31 13:36:37 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.*;
import java.io.UnsupportedEncodingException;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLDecoder;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class Agreement extends PayPalResource {
/**
* Identifier of the agreement.
*/
private String id;
/**
* State of the agreement
*/
private String state;
/**
* Name of the agreement.
*/
private String name;
/**
* Description of the agreement.
*/
private String description;
/**
* Start date of the agreement. Date format yyyy-MM-dd z, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
private String startDate;
/**
* Details of the agreement.
*/
private AgreementDetails agreementDetails;
/**
* Details of the buyer who is enrolling in this agreement. This information is gathered from execution of the approval URL.
*/
private Payer payer;
/**
* Shipping address object of the agreement, which should be provided if it is different from the default address.
*/
private Address shippingAddress;
/**
* Default merchant preferences from the billing plan are used, unless override preferences are provided here.
*/
private MerchantPreferences overrideMerchantPreferences;
/**
* Array of override_charge_model for this agreement if needed to change the default models from the billing plan.
*/
private List overrideChargeModels;
/**
* Plan details for this agreement.
*/
private Plan plan;
/**
* Date and time that this resource was created. Date format yyyy-MM-dd z, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
private String createTime;
/**
* Date and time that this resource was updated. Date format yyyy-MM-dd z, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
private String updateTime;
/**
* Payment token
*/
private String token;
/**
*/
private List links;
/**
* Default Constructor
*/
public Agreement() {
}
/**
* Parameterized Constructor
*/
public Agreement(String name, String description, String startDate, Payer payer, Plan plan) {
this.name = name;
this.description = description;
this.startDate = startDate;
this.payer = payer;
this.plan = plan;
}
/**
* Create a new billing agreement by passing the details for the agreement, including the name, description, start date, payer, and billing plan in the request JSON.
* @deprecated Please use {@link #create(APIContext)} instead.
*
* @param accessToken
* Access Token used for the API call.
* @return Agreement
* @throws PayPalRESTException
* @throws UnsupportedEncodingException
* @throws MalformedURLException
*/
public Agreement create(String accessToken) throws PayPalRESTException, MalformedURLException, UnsupportedEncodingException {
APIContext apiContext = new APIContext(accessToken);
return create(apiContext);
}
/**
* Create a new billing agreement by passing the details for the agreement, including the name, description, start date, payer, and billing plan in the request JSON.
* @param apiContext
* {@link APIContext} used for the API call.
* @return Agreement
* @throws PayPalRESTException
* @throws MalformedURLException
* @throws UnsupportedEncodingException
*/
public Agreement create(APIContext apiContext) throws PayPalRESTException, MalformedURLException, UnsupportedEncodingException {
String resourcePath = "v1/payments/billing-agreements";
String payLoad = this.toJSON();
Agreement agreement = configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, Agreement.class);
for (Links links : agreement.getLinks()) {
if ("approval_url".equals(links.getRel())) {
URL url = new URL(links.getHref());
agreement.setToken(splitQuery(url).get("token"));
break;
}
}
return agreement;
}
/**
* Helper class to parse Query part of a URL
* @param url
* @return Query part in the given URL in name-value pair
* @throws UnsupportedEncodingException
*/
private static Map splitQuery(URL url) throws UnsupportedEncodingException {
Map queryPairs = new HashMap();
String query = url.getQuery();
String[] pairs = query.split("&");
for (String pair : pairs) {
int idx = pair.indexOf("=");
queryPairs.put(URLDecoder.decode(pair.substring(0, idx), "UTF-8"), URLDecoder.decode(pair.substring(idx + 1), "UTF-8"));
}
return queryPairs;
}
/**
* Execute a billing agreement after buyer approval by passing the payment token to the request URI.
* @deprecated Please use {@link #execute(APIContext, String)} instead.
* @param accessToken
* Access Token used for the API call.
* @return Agreement
* @throws PayPalRESTException
*/
public Agreement execute(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return execute(apiContext, this.getToken());
}
/**
* Execute a billing agreement after buyer approval by passing the payment token to the request URI.
* @param apiContext
* {@link APIContext} used for the API call.
* @param token
* payment token (e.g., EC-0JP008296V451950C)
* @return Agreement
* @throws PayPalRESTException
*/
public static Agreement execute(APIContext apiContext, String token) throws PayPalRESTException {
Object[] parameters = new Object[] {token};
String pattern = "v1/payments/billing-agreements/{0}/agreement-execute";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, Agreement.class);
}
/**
* Retrieve details for a particular billing agreement by passing the ID of the agreement to the request URI.
* @deprecated Please use {@link #get(APIContext, String)} instead.
*
* @param accessToken
* Access Token used for the API call.
* @param agreementId
* String
* @return Agreement
* @throws PayPalRESTException
*/
public static Agreement get(String accessToken, String agreementId) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return get(apiContext, agreementId);
}
/**
* Retrieve details for a particular billing agreement by passing the ID of the agreement to the request URI.
* @param apiContext
* {@link APIContext} used for the API call.
* @param agreementId
* String
* @return Agreement
* @throws PayPalRESTException
*/
public static Agreement get(APIContext apiContext, String agreementId) throws PayPalRESTException {
if (agreementId == null) {
throw new IllegalArgumentException("agreementId cannot be null");
}
Object[] parameters = new Object[] {agreementId};
String pattern = "v1/payments/billing-agreements/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, Agreement.class);
}
/**
* Update details of a billing agreement, such as the description, shipping address, and start date, by passing the ID of the agreement to the request URI.
* @deprecated Please use {@link #update(APIContext, List)} instead.
* @param accessToken
* Access Token used for the API call.
* @param patchRequest
* PatchRequest
* @return Agreement
* @throws PayPalRESTException
*/
public Agreement update(String accessToken, List patchRequest) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return update(apiContext, patchRequest);
}
/**
* Update details of a billing agreement, such as the description, shipping address, and start date, by passing the ID of the agreement to the request URI.
* @param apiContext
* {@link APIContext} used for the API call.
* @param patchRequest
* PatchRequest (list of patches)
* @return Agreement
* @throws PayPalRESTException
*/
public Agreement update(APIContext apiContext, List patchRequest) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (patchRequest == null) {
throw new IllegalArgumentException("patchRequest cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payments/billing-agreements/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = JSONFormatter.toJSON(patchRequest);
return configureAndExecute(apiContext, HttpMethod.PATCH, resourcePath, payLoad, Agreement.class);
}
/**
* Suspend a particular billing agreement by passing the ID of the agreement to the request URI.
* @deprecated Please use {@link #suspend(APIContext, AgreementStateDescriptor)} instead.
* @param accessToken
* Access Token used for the API call.
* @param agreementStateDescriptor
* AgreementStateDescriptor
* @throws PayPalRESTException
*/
public void suspend(String accessToken, AgreementStateDescriptor agreementStateDescriptor) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
suspend(apiContext, agreementStateDescriptor);
return;
}
/**
* Suspend a particular billing agreement by passing the ID of the agreement to the request URI.
* @param apiContext
* {@link APIContext} used for the API call.
* @param agreementStateDescriptor
* AgreementStateDescriptor
* @throws PayPalRESTException
*/
public void suspend(APIContext apiContext, AgreementStateDescriptor agreementStateDescriptor) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (agreementStateDescriptor == null) {
throw new IllegalArgumentException("agreementStateDescriptor cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payments/billing-agreements/{0}/suspend";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = agreementStateDescriptor.toJSON();
configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, null);
return;
}
/**
* Reactivate a suspended billing agreement by passing the ID of the agreement to the appropriate URI. In addition, pass an agreement_state_descriptor object in the request JSON that includes a note about the reason for changing the state of the agreement and the amount and currency for the agreement.
* @deprecated Please use {@link #reActivate(APIContext, AgreementStateDescriptor)} instead.
* @param accessToken
* Access Token used for the API call.
* @param agreementStateDescriptor
* AgreementStateDescriptor
* @throws PayPalRESTException
*/
public void reActivate(String accessToken, AgreementStateDescriptor agreementStateDescriptor) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
reActivate(apiContext, agreementStateDescriptor);
return;
}
/**
* Reactivate a suspended billing agreement by passing the ID of the agreement to the appropriate URI. In addition, pass an agreement_state_descriptor object in the request JSON that includes a note about the reason for changing the state of the agreement and the amount and currency for the agreement.
* @param apiContext
* {@link APIContext} used for the API call.
* @param agreementStateDescriptor
* AgreementStateDescriptor
* @throws PayPalRESTException
*/
public void reActivate(APIContext apiContext, AgreementStateDescriptor agreementStateDescriptor) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (agreementStateDescriptor == null) {
throw new IllegalArgumentException("agreementStateDescriptor cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payments/billing-agreements/{0}/re-activate";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = agreementStateDescriptor.toJSON();
configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, null);
return;
}
/**
* Cancel a billing agreement by passing the ID of the agreement to the request URI. In addition, pass an agreement_state_descriptor object in the request JSON that includes a note about the reason for changing the state of the agreement and the amount and currency for the agreement.
* @deprecated Please use {@link #cancel(APIContext, AgreementStateDescriptor)} instead.
* @param accessToken
* Access Token used for the API call.
* @param agreementStateDescriptor
* AgreementStateDescriptor
* @throws PayPalRESTException
*/
public void cancel(String accessToken, AgreementStateDescriptor agreementStateDescriptor) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
cancel(apiContext, agreementStateDescriptor);
return;
}
/**
* Cancel a billing agreement by passing the ID of the agreement to the request URI. In addition, pass an agreement_state_descriptor object in the request JSON that includes a note about the reason for changing the state of the agreement and the amount and currency for the agreement.
* @param apiContext
* {@link APIContext} used for the API call.
* @param agreementStateDescriptor
* AgreementStateDescriptor
* @throws PayPalRESTException
*/
public void cancel(APIContext apiContext, AgreementStateDescriptor agreementStateDescriptor) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (agreementStateDescriptor == null) {
throw new IllegalArgumentException("agreementStateDescriptor cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payments/billing-agreements/{0}/cancel";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = agreementStateDescriptor.toJSON();
configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, null);
return;
}
/**
* Bill an outstanding amount for an agreement by passing the ID of the agreement to the request URI. In addition, pass an agreement_state_descriptor object in the request JSON that includes a note about the reason for changing the state of the agreement and the amount and currency for the agreement.
* @deprecated Please use {@link #billBalance(APIContext, AgreementStateDescriptor)} instead.
* @param accessToken
* Access Token used for the API call.
* @param agreementStateDescriptor
* AgreementStateDescriptor
* @throws PayPalRESTException
*/
public void billBalance(String accessToken, AgreementStateDescriptor agreementStateDescriptor) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
billBalance(apiContext, agreementStateDescriptor);
return;
}
/**
* Bill an outstanding amount for an agreement by passing the ID of the agreement to the request URI. In addition, pass an agreement_state_descriptor object in the request JSON that includes a note about the reason for changing the state of the agreement and the amount and currency for the agreement.
* @param apiContext
* {@link APIContext} used for the API call.
* @param agreementStateDescriptor
* AgreementStateDescriptor
* @throws PayPalRESTException
*/
public void billBalance(APIContext apiContext, AgreementStateDescriptor agreementStateDescriptor) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (agreementStateDescriptor == null) {
throw new IllegalArgumentException("agreementStateDescriptor cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payments/billing-agreements/{0}/bill-balance";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = agreementStateDescriptor.toJSON();
configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, null);
return;
}
/**
* Set the balance for an agreement by passing the ID of the agreement to the request URI. In addition, pass a common_currency object in the request JSON that specifies the currency type and value of the balance.
* @deprecated Please use {@link #setBalance(APIContext, Currency)} instead.
* @param accessToken
* Access Token used for the API call.
* @param currency
* Currency
* @throws PayPalRESTException
*/
public void setBalance(String accessToken, Currency currency) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
setBalance(apiContext, currency);
return;
}
/**
* Set the balance for an agreement by passing the ID of the agreement to the request URI. In addition, pass a common_currency object in the request JSON that specifies the currency type and value of the balance.
* @param apiContext
* {@link APIContext} used for the API call.
* @param currency
* Currency
* @throws PayPalRESTException
*/
public void setBalance(APIContext apiContext, Currency currency) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (currency == null) {
throw new IllegalArgumentException("currency cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payments/billing-agreements/{0}/set-balance";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = currency.toJSON();
configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, null);
return;
}
/**
* List transactions for a billing agreement by passing the ID of the agreement, as well as the start and end dates of the range of transactions to list, to the request URI.
* @deprecated Please use {@link #transactions(APIContext, String, Date, Date)} instead.
* @param accessToken
* Access Token used for the API call.
* @param agreementId
* String
* @return AgreementTransactions
* @throws PayPalRESTException
*/
public static AgreementTransactions transactions(String accessToken, String agreementId, Date startDate, Date endDate) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return transactions(apiContext, agreementId, startDate, endDate);
}
/**
* List transactions for a billing agreement by passing the ID of the agreement, as well as the start and end dates of the range of transactions to list, to the request URI.
* @param apiContext
* {@link APIContext} used for the API call.
* @param agreementId
* String
* @return AgreementTransactions
* @throws PayPalRESTException
*/
public static AgreementTransactions transactions(APIContext apiContext, String agreementId, Date startDate, Date endDate) throws PayPalRESTException {
if (startDate == null) {
throw new IllegalArgumentException("startDate cannot be null");
}
if (endDate == null) {
throw new IllegalArgumentException("endDate cannot be null");
}
if (agreementId == null) {
throw new IllegalArgumentException("agreementId cannot be null");
}
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
String sDate = dateFormat.format(startDate);
String eDate = dateFormat.format(endDate);
Object[] parameters = new Object[] {agreementId, sDate, eDate};
String pattern = "v1/payments/billing-agreements/{0}/transactions?start_date={1}&end_date={2}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
AgreementTransactions transactions = configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, AgreementTransactions.class);
return transactions;
}
/**
* Identifier of the agreement.
*/
@java.lang.SuppressWarnings("all")
public String getId() {
return this.id;
}
/**
* State of the agreement
*/
@java.lang.SuppressWarnings("all")
public String getState() {
return this.state;
}
/**
* Name of the agreement.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* Description of the agreement.
*/
@java.lang.SuppressWarnings("all")
public String getDescription() {
return this.description;
}
/**
* Start date of the agreement. Date format yyyy-MM-dd z, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
@java.lang.SuppressWarnings("all")
public String getStartDate() {
return this.startDate;
}
/**
* Details of the agreement.
*/
@java.lang.SuppressWarnings("all")
public AgreementDetails getAgreementDetails() {
return this.agreementDetails;
}
/**
* Details of the buyer who is enrolling in this agreement. This information is gathered from execution of the approval URL.
*/
@java.lang.SuppressWarnings("all")
public Payer getPayer() {
return this.payer;
}
/**
* Shipping address object of the agreement, which should be provided if it is different from the default address.
*/
@java.lang.SuppressWarnings("all")
public Address getShippingAddress() {
return this.shippingAddress;
}
/**
* Default merchant preferences from the billing plan are used, unless override preferences are provided here.
*/
@java.lang.SuppressWarnings("all")
public MerchantPreferences getOverrideMerchantPreferences() {
return this.overrideMerchantPreferences;
}
/**
* Array of override_charge_model for this agreement if needed to change the default models from the billing plan.
*/
@java.lang.SuppressWarnings("all")
public List getOverrideChargeModels() {
return this.overrideChargeModels;
}
/**
* Plan details for this agreement.
*/
@java.lang.SuppressWarnings("all")
public Plan getPlan() {
return this.plan;
}
/**
* Date and time that this resource was created. Date format yyyy-MM-dd z, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
@java.lang.SuppressWarnings("all")
public String getCreateTime() {
return this.createTime;
}
/**
* Date and time that this resource was updated. Date format yyyy-MM-dd z, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
@java.lang.SuppressWarnings("all")
public String getUpdateTime() {
return this.updateTime;
}
/**
* Payment token
*/
@java.lang.SuppressWarnings("all")
public String getToken() {
return this.token;
}
/**
*/
@java.lang.SuppressWarnings("all")
public List getLinks() {
return this.links;
}
/**
* Identifier of the agreement.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setId(final String id) {
this.id = id;
return this;
}
/**
* State of the agreement
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setState(final String state) {
this.state = state;
return this;
}
/**
* Name of the agreement.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setName(final String name) {
this.name = name;
return this;
}
/**
* Description of the agreement.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setDescription(final String description) {
this.description = description;
return this;
}
/**
* Start date of the agreement. Date format yyyy-MM-dd z, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setStartDate(final String startDate) {
this.startDate = startDate;
return this;
}
/**
* Details of the agreement.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setAgreementDetails(final AgreementDetails agreementDetails) {
this.agreementDetails = agreementDetails;
return this;
}
/**
* Details of the buyer who is enrolling in this agreement. This information is gathered from execution of the approval URL.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setPayer(final Payer payer) {
this.payer = payer;
return this;
}
/**
* Shipping address object of the agreement, which should be provided if it is different from the default address.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setShippingAddress(final Address shippingAddress) {
this.shippingAddress = shippingAddress;
return this;
}
/**
* Default merchant preferences from the billing plan are used, unless override preferences are provided here.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setOverrideMerchantPreferences(final MerchantPreferences overrideMerchantPreferences) {
this.overrideMerchantPreferences = overrideMerchantPreferences;
return this;
}
/**
* Array of override_charge_model for this agreement if needed to change the default models from the billing plan.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setOverrideChargeModels(final List overrideChargeModels) {
this.overrideChargeModels = overrideChargeModels;
return this;
}
/**
* Plan details for this agreement.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setPlan(final Plan plan) {
this.plan = plan;
return this;
}
/**
* Date and time that this resource was created. Date format yyyy-MM-dd z, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setCreateTime(final String createTime) {
this.createTime = createTime;
return this;
}
/**
* Date and time that this resource was updated. Date format yyyy-MM-dd z, as defined in [ISO8601](http://tools.ietf.org/html/rfc3339#section-5.6).
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setUpdateTime(final String updateTime) {
this.updateTime = updateTime;
return this;
}
/**
* Payment token
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setToken(final String token) {
this.token = token;
return this;
}
/**
*
* @return this
*/
@java.lang.SuppressWarnings("all")
public Agreement setLinks(final List links) {
this.links = links;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Agreement)) return false;
final Agreement other = (Agreement) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$state = this.getState();
final java.lang.Object other$state = other.getState();
if (this$state == null ? other$state != null : !this$state.equals(other$state)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$startDate = this.getStartDate();
final java.lang.Object other$startDate = other.getStartDate();
if (this$startDate == null ? other$startDate != null : !this$startDate.equals(other$startDate)) return false;
final java.lang.Object this$agreementDetails = this.getAgreementDetails();
final java.lang.Object other$agreementDetails = other.getAgreementDetails();
if (this$agreementDetails == null ? other$agreementDetails != null : !this$agreementDetails.equals(other$agreementDetails)) return false;
final java.lang.Object this$payer = this.getPayer();
final java.lang.Object other$payer = other.getPayer();
if (this$payer == null ? other$payer != null : !this$payer.equals(other$payer)) return false;
final java.lang.Object this$shippingAddress = this.getShippingAddress();
final java.lang.Object other$shippingAddress = other.getShippingAddress();
if (this$shippingAddress == null ? other$shippingAddress != null : !this$shippingAddress.equals(other$shippingAddress)) return false;
final java.lang.Object this$overrideMerchantPreferences = this.getOverrideMerchantPreferences();
final java.lang.Object other$overrideMerchantPreferences = other.getOverrideMerchantPreferences();
if (this$overrideMerchantPreferences == null ? other$overrideMerchantPreferences != null : !this$overrideMerchantPreferences.equals(other$overrideMerchantPreferences)) return false;
final java.lang.Object this$overrideChargeModels = this.getOverrideChargeModels();
final java.lang.Object other$overrideChargeModels = other.getOverrideChargeModels();
if (this$overrideChargeModels == null ? other$overrideChargeModels != null : !this$overrideChargeModels.equals(other$overrideChargeModels)) return false;
final java.lang.Object this$plan = this.getPlan();
final java.lang.Object other$plan = other.getPlan();
if (this$plan == null ? other$plan != null : !this$plan.equals(other$plan)) return false;
final java.lang.Object this$createTime = this.getCreateTime();
final java.lang.Object other$createTime = other.getCreateTime();
if (this$createTime == null ? other$createTime != null : !this$createTime.equals(other$createTime)) return false;
final java.lang.Object this$updateTime = this.getUpdateTime();
final java.lang.Object other$updateTime = other.getUpdateTime();
if (this$updateTime == null ? other$updateTime != null : !this$updateTime.equals(other$updateTime)) return false;
final java.lang.Object this$token = this.getToken();
final java.lang.Object other$token = other.getToken();
if (this$token == null ? other$token != null : !this$token.equals(other$token)) return false;
final java.lang.Object this$links = this.getLinks();
final java.lang.Object other$links = other.getLinks();
if (this$links == null ? other$links != null : !this$links.equals(other$links)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Agreement;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $state = this.getState();
result = result * PRIME + ($state == null ? 43 : $state.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $startDate = this.getStartDate();
result = result * PRIME + ($startDate == null ? 43 : $startDate.hashCode());
final java.lang.Object $agreementDetails = this.getAgreementDetails();
result = result * PRIME + ($agreementDetails == null ? 43 : $agreementDetails.hashCode());
final java.lang.Object $payer = this.getPayer();
result = result * PRIME + ($payer == null ? 43 : $payer.hashCode());
final java.lang.Object $shippingAddress = this.getShippingAddress();
result = result * PRIME + ($shippingAddress == null ? 43 : $shippingAddress.hashCode());
final java.lang.Object $overrideMerchantPreferences = this.getOverrideMerchantPreferences();
result = result * PRIME + ($overrideMerchantPreferences == null ? 43 : $overrideMerchantPreferences.hashCode());
final java.lang.Object $overrideChargeModels = this.getOverrideChargeModels();
result = result * PRIME + ($overrideChargeModels == null ? 43 : $overrideChargeModels.hashCode());
final java.lang.Object $plan = this.getPlan();
result = result * PRIME + ($plan == null ? 43 : $plan.hashCode());
final java.lang.Object $createTime = this.getCreateTime();
result = result * PRIME + ($createTime == null ? 43 : $createTime.hashCode());
final java.lang.Object $updateTime = this.getUpdateTime();
result = result * PRIME + ($updateTime == null ? 43 : $updateTime.hashCode());
final java.lang.Object $token = this.getToken();
result = result * PRIME + ($token == null ? 43 : $token.hashCode());
final java.lang.Object $links = this.getLinks();
result = result * PRIME + ($links == null ? 43 : $links.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy