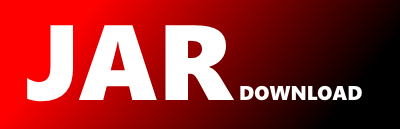
com.paypal.api.payments.CreditCardToken Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Tue Jan 31 13:36:37 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class CreditCardToken extends PayPalModel {
/**
* ID of credit card previously stored using `/vault/credit-card`.
*/
private String creditCardId;
/**
* A unique identifier that you can assign and track when storing a credit card or using a stored credit card. This ID can help to avoid unintentional use or misuse of credit cards. This ID can be any value you would like to associate with the saved card, such as a UUID, username, or email address. **Required when using a stored credit card if a payer_id was originally provided when storing the credit card in vault.**
*/
private String payerId;
/**
* Last four digits of the stored credit card number.
*/
private String last4;
/**
* Credit card type. Valid types are: `visa`, `mastercard`, `discover`, `amex`. Values are presented in lowercase and not should not be used for display.
*/
private String type;
/**
* Expiration month with no leading zero. Acceptable values are 1 through 12.
*/
private int expireMonth;
/**
* 4-digit expiration year.
*/
private int expireYear;
/**
* Default Constructor
*/
public CreditCardToken() {
}
/**
* Parameterized Constructor
*/
public CreditCardToken(String creditCardId) {
this.creditCardId = creditCardId;
}
/**
* ID of credit card previously stored using `/vault/credit-card`.
*/
@java.lang.SuppressWarnings("all")
public String getCreditCardId() {
return this.creditCardId;
}
/**
* A unique identifier that you can assign and track when storing a credit card or using a stored credit card. This ID can help to avoid unintentional use or misuse of credit cards. This ID can be any value you would like to associate with the saved card, such as a UUID, username, or email address. **Required when using a stored credit card if a payer_id was originally provided when storing the credit card in vault.**
*/
@java.lang.SuppressWarnings("all")
public String getPayerId() {
return this.payerId;
}
/**
* Last four digits of the stored credit card number.
*/
@java.lang.SuppressWarnings("all")
public String getLast4() {
return this.last4;
}
/**
* Credit card type. Valid types are: `visa`, `mastercard`, `discover`, `amex`. Values are presented in lowercase and not should not be used for display.
*/
@java.lang.SuppressWarnings("all")
public String getType() {
return this.type;
}
/**
* Expiration month with no leading zero. Acceptable values are 1 through 12.
*/
@java.lang.SuppressWarnings("all")
public int getExpireMonth() {
return this.expireMonth;
}
/**
* 4-digit expiration year.
*/
@java.lang.SuppressWarnings("all")
public int getExpireYear() {
return this.expireYear;
}
/**
* ID of credit card previously stored using `/vault/credit-card`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CreditCardToken setCreditCardId(final String creditCardId) {
this.creditCardId = creditCardId;
return this;
}
/**
* A unique identifier that you can assign and track when storing a credit card or using a stored credit card. This ID can help to avoid unintentional use or misuse of credit cards. This ID can be any value you would like to associate with the saved card, such as a UUID, username, or email address. **Required when using a stored credit card if a payer_id was originally provided when storing the credit card in vault.**
* @return this
*/
@java.lang.SuppressWarnings("all")
public CreditCardToken setPayerId(final String payerId) {
this.payerId = payerId;
return this;
}
/**
* Last four digits of the stored credit card number.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CreditCardToken setLast4(final String last4) {
this.last4 = last4;
return this;
}
/**
* Credit card type. Valid types are: `visa`, `mastercard`, `discover`, `amex`. Values are presented in lowercase and not should not be used for display.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CreditCardToken setType(final String type) {
this.type = type;
return this;
}
/**
* Expiration month with no leading zero. Acceptable values are 1 through 12.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CreditCardToken setExpireMonth(final int expireMonth) {
this.expireMonth = expireMonth;
return this;
}
/**
* 4-digit expiration year.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CreditCardToken setExpireYear(final int expireYear) {
this.expireYear = expireYear;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof CreditCardToken)) return false;
final CreditCardToken other = (CreditCardToken) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$creditCardId = this.getCreditCardId();
final java.lang.Object other$creditCardId = other.getCreditCardId();
if (this$creditCardId == null ? other$creditCardId != null : !this$creditCardId.equals(other$creditCardId)) return false;
final java.lang.Object this$payerId = this.getPayerId();
final java.lang.Object other$payerId = other.getPayerId();
if (this$payerId == null ? other$payerId != null : !this$payerId.equals(other$payerId)) return false;
final java.lang.Object this$last4 = this.getLast4();
final java.lang.Object other$last4 = other.getLast4();
if (this$last4 == null ? other$last4 != null : !this$last4.equals(other$last4)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
if (this.getExpireMonth() != other.getExpireMonth()) return false;
if (this.getExpireYear() != other.getExpireYear()) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof CreditCardToken;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $creditCardId = this.getCreditCardId();
result = result * PRIME + ($creditCardId == null ? 43 : $creditCardId.hashCode());
final java.lang.Object $payerId = this.getPayerId();
result = result * PRIME + ($payerId == null ? 43 : $payerId.hashCode());
final java.lang.Object $last4 = this.getLast4();
result = result * PRIME + ($last4 == null ? 43 : $last4.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
result = result * PRIME + this.getExpireMonth();
result = result * PRIME + this.getExpireYear();
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy