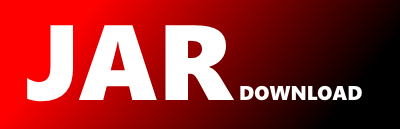
com.paypal.api.payments.DetailedRefund Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Tue Jan 31 13:36:37 CST 2017
package com.paypal.api.payments;
import java.util.List;
public class DetailedRefund extends Refund {
/**
* free-form field for the use of clients
*/
private String custom;
/**
* Amount refunded to payer of the original transaction, in the current Refund call
*/
private Currency refundToPayer;
/**
* List of external funding that were refunded by the Refund call. Each external_funding unit should have a unique reference_id
*/
private List refundToExternalFunding;
/**
* Transaction fee refunded to original recipient of payment.
*/
private Currency refundFromTransactionFee;
/**
* Amount subtracted from PayPal balance of the original recipient of payment, to make this refund.
*/
private Currency refundFromReceivedAmount;
/**
* Total amount refunded so far from the original purchase. Say, for example, a buyer makes $100 purchase, the buyer was refunded $20 a week ago and is refunded $30 in this transaction. The gross refund amount is $30 (in this transaction). The total refunded amount is $50.
*/
private Currency totalRefundedAmount;
/**
* Default Constructor
*/
public DetailedRefund() {
}
/**
* free-form field for the use of clients
*/
@java.lang.SuppressWarnings("all")
public String getCustom() {
return this.custom;
}
/**
* Amount refunded to payer of the original transaction, in the current Refund call
*/
@java.lang.SuppressWarnings("all")
public Currency getRefundToPayer() {
return this.refundToPayer;
}
/**
* List of external funding that were refunded by the Refund call. Each external_funding unit should have a unique reference_id
*/
@java.lang.SuppressWarnings("all")
public List getRefundToExternalFunding() {
return this.refundToExternalFunding;
}
/**
* Transaction fee refunded to original recipient of payment.
*/
@java.lang.SuppressWarnings("all")
public Currency getRefundFromTransactionFee() {
return this.refundFromTransactionFee;
}
/**
* Amount subtracted from PayPal balance of the original recipient of payment, to make this refund.
*/
@java.lang.SuppressWarnings("all")
public Currency getRefundFromReceivedAmount() {
return this.refundFromReceivedAmount;
}
/**
* Total amount refunded so far from the original purchase. Say, for example, a buyer makes $100 purchase, the buyer was refunded $20 a week ago and is refunded $30 in this transaction. The gross refund amount is $30 (in this transaction). The total refunded amount is $50.
*/
@java.lang.SuppressWarnings("all")
public Currency getTotalRefundedAmount() {
return this.totalRefundedAmount;
}
/**
* free-form field for the use of clients
* @return this
*/
@java.lang.SuppressWarnings("all")
public DetailedRefund setCustom(final String custom) {
this.custom = custom;
return this;
}
/**
* Amount refunded to payer of the original transaction, in the current Refund call
* @return this
*/
@java.lang.SuppressWarnings("all")
public DetailedRefund setRefundToPayer(final Currency refundToPayer) {
this.refundToPayer = refundToPayer;
return this;
}
/**
* List of external funding that were refunded by the Refund call. Each external_funding unit should have a unique reference_id
* @return this
*/
@java.lang.SuppressWarnings("all")
public DetailedRefund setRefundToExternalFunding(final List refundToExternalFunding) {
this.refundToExternalFunding = refundToExternalFunding;
return this;
}
/**
* Transaction fee refunded to original recipient of payment.
* @return this
*/
@java.lang.SuppressWarnings("all")
public DetailedRefund setRefundFromTransactionFee(final Currency refundFromTransactionFee) {
this.refundFromTransactionFee = refundFromTransactionFee;
return this;
}
/**
* Amount subtracted from PayPal balance of the original recipient of payment, to make this refund.
* @return this
*/
@java.lang.SuppressWarnings("all")
public DetailedRefund setRefundFromReceivedAmount(final Currency refundFromReceivedAmount) {
this.refundFromReceivedAmount = refundFromReceivedAmount;
return this;
}
/**
* Total amount refunded so far from the original purchase. Say, for example, a buyer makes $100 purchase, the buyer was refunded $20 a week ago and is refunded $30 in this transaction. The gross refund amount is $30 (in this transaction). The total refunded amount is $50.
* @return this
*/
@java.lang.SuppressWarnings("all")
public DetailedRefund setTotalRefundedAmount(final Currency totalRefundedAmount) {
this.totalRefundedAmount = totalRefundedAmount;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof DetailedRefund)) return false;
final DetailedRefund other = (DetailedRefund) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$custom = this.getCustom();
final java.lang.Object other$custom = other.getCustom();
if (this$custom == null ? other$custom != null : !this$custom.equals(other$custom)) return false;
final java.lang.Object this$refundToPayer = this.getRefundToPayer();
final java.lang.Object other$refundToPayer = other.getRefundToPayer();
if (this$refundToPayer == null ? other$refundToPayer != null : !this$refundToPayer.equals(other$refundToPayer)) return false;
final java.lang.Object this$refundToExternalFunding = this.getRefundToExternalFunding();
final java.lang.Object other$refundToExternalFunding = other.getRefundToExternalFunding();
if (this$refundToExternalFunding == null ? other$refundToExternalFunding != null : !this$refundToExternalFunding.equals(other$refundToExternalFunding)) return false;
final java.lang.Object this$refundFromTransactionFee = this.getRefundFromTransactionFee();
final java.lang.Object other$refundFromTransactionFee = other.getRefundFromTransactionFee();
if (this$refundFromTransactionFee == null ? other$refundFromTransactionFee != null : !this$refundFromTransactionFee.equals(other$refundFromTransactionFee)) return false;
final java.lang.Object this$refundFromReceivedAmount = this.getRefundFromReceivedAmount();
final java.lang.Object other$refundFromReceivedAmount = other.getRefundFromReceivedAmount();
if (this$refundFromReceivedAmount == null ? other$refundFromReceivedAmount != null : !this$refundFromReceivedAmount.equals(other$refundFromReceivedAmount)) return false;
final java.lang.Object this$totalRefundedAmount = this.getTotalRefundedAmount();
final java.lang.Object other$totalRefundedAmount = other.getTotalRefundedAmount();
if (this$totalRefundedAmount == null ? other$totalRefundedAmount != null : !this$totalRefundedAmount.equals(other$totalRefundedAmount)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof DetailedRefund;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $custom = this.getCustom();
result = result * PRIME + ($custom == null ? 43 : $custom.hashCode());
final java.lang.Object $refundToPayer = this.getRefundToPayer();
result = result * PRIME + ($refundToPayer == null ? 43 : $refundToPayer.hashCode());
final java.lang.Object $refundToExternalFunding = this.getRefundToExternalFunding();
result = result * PRIME + ($refundToExternalFunding == null ? 43 : $refundToExternalFunding.hashCode());
final java.lang.Object $refundFromTransactionFee = this.getRefundFromTransactionFee();
result = result * PRIME + ($refundFromTransactionFee == null ? 43 : $refundFromTransactionFee.hashCode());
final java.lang.Object $refundFromReceivedAmount = this.getRefundFromReceivedAmount();
result = result * PRIME + ($refundFromReceivedAmount == null ? 43 : $refundFromReceivedAmount.hashCode());
final java.lang.Object $totalRefundedAmount = this.getTotalRefundedAmount();
result = result * PRIME + ($totalRefundedAmount == null ? 43 : $totalRefundedAmount.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy