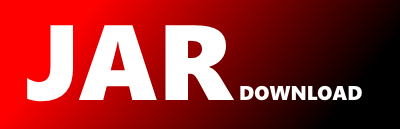
com.paypal.api.payments.ExternalFunding Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Tue Jan 31 13:36:37 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class ExternalFunding extends PayPalModel {
/**
* Unique identifier for the external funding
*/
private String referenceId;
/**
* Generic identifier for the external funding
*/
private String code;
/**
* Encrypted PayPal Account identifier for the funding account
*/
private String fundingAccountId;
/**
* Description of the external funding being applied
*/
private String displayText;
/**
* Amount being funded by the external funding account
*/
private Amount amount;
/**
* Indicates that the Payment should be fully funded by External Funded Incentive
*/
private String fundingInstruction;
/**
* Default Constructor
*/
public ExternalFunding() {
}
/**
* Parameterized Constructor
*/
public ExternalFunding(String referenceId, String fundingAccountId, Amount amount) {
this.referenceId = referenceId;
this.fundingAccountId = fundingAccountId;
this.amount = amount;
}
/**
* Unique identifier for the external funding
*/
@java.lang.SuppressWarnings("all")
public String getReferenceId() {
return this.referenceId;
}
/**
* Generic identifier for the external funding
*/
@java.lang.SuppressWarnings("all")
public String getCode() {
return this.code;
}
/**
* Encrypted PayPal Account identifier for the funding account
*/
@java.lang.SuppressWarnings("all")
public String getFundingAccountId() {
return this.fundingAccountId;
}
/**
* Description of the external funding being applied
*/
@java.lang.SuppressWarnings("all")
public String getDisplayText() {
return this.displayText;
}
/**
* Amount being funded by the external funding account
*/
@java.lang.SuppressWarnings("all")
public Amount getAmount() {
return this.amount;
}
/**
* Indicates that the Payment should be fully funded by External Funded Incentive
*/
@java.lang.SuppressWarnings("all")
public String getFundingInstruction() {
return this.fundingInstruction;
}
/**
* Unique identifier for the external funding
* @return this
*/
@java.lang.SuppressWarnings("all")
public ExternalFunding setReferenceId(final String referenceId) {
this.referenceId = referenceId;
return this;
}
/**
* Generic identifier for the external funding
* @return this
*/
@java.lang.SuppressWarnings("all")
public ExternalFunding setCode(final String code) {
this.code = code;
return this;
}
/**
* Encrypted PayPal Account identifier for the funding account
* @return this
*/
@java.lang.SuppressWarnings("all")
public ExternalFunding setFundingAccountId(final String fundingAccountId) {
this.fundingAccountId = fundingAccountId;
return this;
}
/**
* Description of the external funding being applied
* @return this
*/
@java.lang.SuppressWarnings("all")
public ExternalFunding setDisplayText(final String displayText) {
this.displayText = displayText;
return this;
}
/**
* Amount being funded by the external funding account
* @return this
*/
@java.lang.SuppressWarnings("all")
public ExternalFunding setAmount(final Amount amount) {
this.amount = amount;
return this;
}
/**
* Indicates that the Payment should be fully funded by External Funded Incentive
* @return this
*/
@java.lang.SuppressWarnings("all")
public ExternalFunding setFundingInstruction(final String fundingInstruction) {
this.fundingInstruction = fundingInstruction;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ExternalFunding)) return false;
final ExternalFunding other = (ExternalFunding) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$referenceId = this.getReferenceId();
final java.lang.Object other$referenceId = other.getReferenceId();
if (this$referenceId == null ? other$referenceId != null : !this$referenceId.equals(other$referenceId)) return false;
final java.lang.Object this$code = this.getCode();
final java.lang.Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final java.lang.Object this$fundingAccountId = this.getFundingAccountId();
final java.lang.Object other$fundingAccountId = other.getFundingAccountId();
if (this$fundingAccountId == null ? other$fundingAccountId != null : !this$fundingAccountId.equals(other$fundingAccountId)) return false;
final java.lang.Object this$displayText = this.getDisplayText();
final java.lang.Object other$displayText = other.getDisplayText();
if (this$displayText == null ? other$displayText != null : !this$displayText.equals(other$displayText)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$fundingInstruction = this.getFundingInstruction();
final java.lang.Object other$fundingInstruction = other.getFundingInstruction();
if (this$fundingInstruction == null ? other$fundingInstruction != null : !this$fundingInstruction.equals(other$fundingInstruction)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ExternalFunding;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $referenceId = this.getReferenceId();
result = result * PRIME + ($referenceId == null ? 43 : $referenceId.hashCode());
final java.lang.Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final java.lang.Object $fundingAccountId = this.getFundingAccountId();
result = result * PRIME + ($fundingAccountId == null ? 43 : $fundingAccountId.hashCode());
final java.lang.Object $displayText = this.getDisplayText();
result = result * PRIME + ($displayText == null ? 43 : $displayText.hashCode());
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $fundingInstruction = this.getFundingInstruction();
result = result * PRIME + ($fundingInstruction == null ? 43 : $fundingInstruction.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy