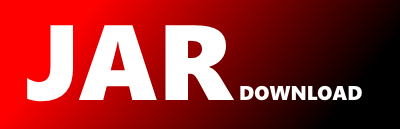
com.paypal.api.payments.FundingDetail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Tue Jan 31 13:36:37 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class FundingDetail extends PayPalModel {
/**
* Expected clearing time
*/
private String clearingTime;
/**
* [DEPRECATED] Hold-off duration of the payment. payment_debit_date should be used instead.
*/
private String paymentHoldDate;
/**
* Date when funds will be debited from the payer's account
*/
private String paymentDebitDate;
/**
* Processing type of the payment card
*/
private String processingType;
/**
* Default Constructor
*/
public FundingDetail() {
}
/**
* Expected clearing time
*/
@java.lang.SuppressWarnings("all")
public String getClearingTime() {
return this.clearingTime;
}
/**
* [DEPRECATED] Hold-off duration of the payment. payment_debit_date should be used instead.
*/
@java.lang.SuppressWarnings("all")
public String getPaymentHoldDate() {
return this.paymentHoldDate;
}
/**
* Date when funds will be debited from the payer's account
*/
@java.lang.SuppressWarnings("all")
public String getPaymentDebitDate() {
return this.paymentDebitDate;
}
/**
* Processing type of the payment card
*/
@java.lang.SuppressWarnings("all")
public String getProcessingType() {
return this.processingType;
}
/**
* Expected clearing time
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingDetail setClearingTime(final String clearingTime) {
this.clearingTime = clearingTime;
return this;
}
/**
* [DEPRECATED] Hold-off duration of the payment. payment_debit_date should be used instead.
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingDetail setPaymentHoldDate(final String paymentHoldDate) {
this.paymentHoldDate = paymentHoldDate;
return this;
}
/**
* Date when funds will be debited from the payer's account
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingDetail setPaymentDebitDate(final String paymentDebitDate) {
this.paymentDebitDate = paymentDebitDate;
return this;
}
/**
* Processing type of the payment card
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingDetail setProcessingType(final String processingType) {
this.processingType = processingType;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof FundingDetail)) return false;
final FundingDetail other = (FundingDetail) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$clearingTime = this.getClearingTime();
final java.lang.Object other$clearingTime = other.getClearingTime();
if (this$clearingTime == null ? other$clearingTime != null : !this$clearingTime.equals(other$clearingTime)) return false;
final java.lang.Object this$paymentHoldDate = this.getPaymentHoldDate();
final java.lang.Object other$paymentHoldDate = other.getPaymentHoldDate();
if (this$paymentHoldDate == null ? other$paymentHoldDate != null : !this$paymentHoldDate.equals(other$paymentHoldDate)) return false;
final java.lang.Object this$paymentDebitDate = this.getPaymentDebitDate();
final java.lang.Object other$paymentDebitDate = other.getPaymentDebitDate();
if (this$paymentDebitDate == null ? other$paymentDebitDate != null : !this$paymentDebitDate.equals(other$paymentDebitDate)) return false;
final java.lang.Object this$processingType = this.getProcessingType();
final java.lang.Object other$processingType = other.getProcessingType();
if (this$processingType == null ? other$processingType != null : !this$processingType.equals(other$processingType)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof FundingDetail;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $clearingTime = this.getClearingTime();
result = result * PRIME + ($clearingTime == null ? 43 : $clearingTime.hashCode());
final java.lang.Object $paymentHoldDate = this.getPaymentHoldDate();
result = result * PRIME + ($paymentHoldDate == null ? 43 : $paymentHoldDate.hashCode());
final java.lang.Object $paymentDebitDate = this.getPaymentDebitDate();
result = result * PRIME + ($paymentDebitDate == null ? 43 : $paymentDebitDate.hashCode());
final java.lang.Object $processingType = this.getProcessingType();
result = result * PRIME + ($processingType == null ? 43 : $processingType.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy