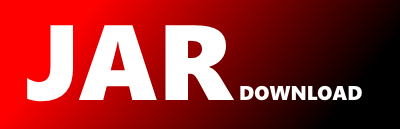
com.paypal.api.payments.PayoutSenderBatchHeader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Tue Jan 31 13:36:37 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class PayoutSenderBatchHeader extends PayPalModel {
/**
* A sender-specified ID number. Tracks the batch payout in an accounting system.Note: PayPal prevents duplicate batches from being processed. If you specify a `sender_batch_id` that was used in the last 30 days, the API rejects the request and returns an error message that indicates the duplicate `sender_batch_id` and includes a HATEOAS link to the original batch payout with the same `sender_batch_id`. If you receive a HTTP `5nn` status code, you can safely retry the request with the same `sender_batch_id`. In any case, the API completes a payment only once for a specific `sender_batch_id` that is used within 30 days.
*/
private String senderBatchId;
/**
* The subject line text for the email that PayPal sends when a payout item completes. The subject line is the same for all recipients. Value is an alphanumeric string with a maximum length of 255 single-byte characters.
*/
private String emailSubject;
/**
* The type of ID that identifies the payment receiver. Value is:EMAIL
. Unencrypted email. Value is a string of up to 127 single-byte characters.PHONE
. Unencrypted phone number.Note: The PayPal sandbox does not support the PHONE
recipient type.
PAYPAL_ID
. Encrypted PayPal account number.
If the sender_batch_header
includes the recipient_type
attribute, any payout item without its own recipient_type
attribute uses the recipient_type
value from sender_batch_header
. If the sender_batch_header
omits the recipient_type
attribute, each payout item must include its own recipient_type
value.
*/
private String recipientType;
/**
* Default Constructor
*/
public PayoutSenderBatchHeader() {
}
/**
* A sender-specified ID number. Tracks the batch payout in an accounting system.Note: PayPal prevents duplicate batches from being processed. If you specify a `sender_batch_id` that was used in the last 30 days, the API rejects the request and returns an error message that indicates the duplicate `sender_batch_id` and includes a HATEOAS link to the original batch payout with the same `sender_batch_id`. If you receive a HTTP `5nn` status code, you can safely retry the request with the same `sender_batch_id`. In any case, the API completes a payment only once for a specific `sender_batch_id` that is used within 30 days.
*/
@java.lang.SuppressWarnings("all")
public String getSenderBatchId() {
return this.senderBatchId;
}
/**
* The subject line text for the email that PayPal sends when a payout item completes. The subject line is the same for all recipients. Value is an alphanumeric string with a maximum length of 255 single-byte characters.
*/
@java.lang.SuppressWarnings("all")
public String getEmailSubject() {
return this.emailSubject;
}
/**
* The type of ID that identifies the payment receiver. Value is:EMAIL
. Unencrypted email. Value is a string of up to 127 single-byte characters.PHONE
. Unencrypted phone number.Note: The PayPal sandbox does not support the PHONE
recipient type.
PAYPAL_ID
. Encrypted PayPal account number.
If the sender_batch_header
includes the recipient_type
attribute, any payout item without its own recipient_type
attribute uses the recipient_type
value from sender_batch_header
. If the sender_batch_header
omits the recipient_type
attribute, each payout item must include its own recipient_type
value.
*/
@java.lang.SuppressWarnings("all")
public String getRecipientType() {
return this.recipientType;
}
/**
* A sender-specified ID number. Tracks the batch payout in an accounting system.Note: PayPal prevents duplicate batches from being processed. If you specify a `sender_batch_id` that was used in the last 30 days, the API rejects the request and returns an error message that indicates the duplicate `sender_batch_id` and includes a HATEOAS link to the original batch payout with the same `sender_batch_id`. If you receive a HTTP `5nn` status code, you can safely retry the request with the same `sender_batch_id`. In any case, the API completes a payment only once for a specific `sender_batch_id` that is used within 30 days.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutSenderBatchHeader setSenderBatchId(final String senderBatchId) {
this.senderBatchId = senderBatchId;
return this;
}
/**
* The subject line text for the email that PayPal sends when a payout item completes. The subject line is the same for all recipients. Value is an alphanumeric string with a maximum length of 255 single-byte characters.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutSenderBatchHeader setEmailSubject(final String emailSubject) {
this.emailSubject = emailSubject;
return this;
}
/**
* The type of ID that identifies the payment receiver. Value is:EMAIL
. Unencrypted email. Value is a string of up to 127 single-byte characters.PHONE
. Unencrypted phone number.Note: The PayPal sandbox does not support the PHONE
recipient type.
PAYPAL_ID
. Encrypted PayPal account number.
If the sender_batch_header
includes the recipient_type
attribute, any payout item without its own recipient_type
attribute uses the recipient_type
value from sender_batch_header
. If the sender_batch_header
omits the recipient_type
attribute, each payout item must include its own recipient_type
value.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutSenderBatchHeader setRecipientType(final String recipientType) {
this.recipientType = recipientType;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PayoutSenderBatchHeader)) return false;
final PayoutSenderBatchHeader other = (PayoutSenderBatchHeader) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$senderBatchId = this.getSenderBatchId();
final java.lang.Object other$senderBatchId = other.getSenderBatchId();
if (this$senderBatchId == null ? other$senderBatchId != null : !this$senderBatchId.equals(other$senderBatchId)) return false;
final java.lang.Object this$emailSubject = this.getEmailSubject();
final java.lang.Object other$emailSubject = other.getEmailSubject();
if (this$emailSubject == null ? other$emailSubject != null : !this$emailSubject.equals(other$emailSubject)) return false;
final java.lang.Object this$recipientType = this.getRecipientType();
final java.lang.Object other$recipientType = other.getRecipientType();
if (this$recipientType == null ? other$recipientType != null : !this$recipientType.equals(other$recipientType)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PayoutSenderBatchHeader;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $senderBatchId = this.getSenderBatchId();
result = result * PRIME + ($senderBatchId == null ? 43 : $senderBatchId.hashCode());
final java.lang.Object $emailSubject = this.getEmailSubject();
result = result * PRIME + ($emailSubject == null ? 43 : $emailSubject.hashCode());
final java.lang.Object $recipientType = this.getRecipientType();
result = result * PRIME + ($recipientType == null ? 43 : $recipientType.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy