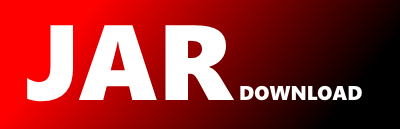
com.paypal.api.payments.PotentialPayerInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Tue Jan 31 13:36:37 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class PotentialPayerInfo extends PayPalModel {
/**
* Email address representing the potential payer.
*/
private String email;
/**
* ExternalRememberMe id representing the potential payer
*/
private String externalRememberMeId;
/**
* Account Number representing the potential payer
*/
private String accountNumber;
/**
* Billing address of the potential payer.
*/
private Address billingAddress;
/**
* Default Constructor
*/
public PotentialPayerInfo() {
}
/**
* Email address representing the potential payer.
*/
@java.lang.SuppressWarnings("all")
public String getEmail() {
return this.email;
}
/**
* ExternalRememberMe id representing the potential payer
*/
@java.lang.SuppressWarnings("all")
public String getExternalRememberMeId() {
return this.externalRememberMeId;
}
/**
* Account Number representing the potential payer
*/
@java.lang.SuppressWarnings("all")
public String getAccountNumber() {
return this.accountNumber;
}
/**
* Billing address of the potential payer.
*/
@java.lang.SuppressWarnings("all")
public Address getBillingAddress() {
return this.billingAddress;
}
/**
* Email address representing the potential payer.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PotentialPayerInfo setEmail(final String email) {
this.email = email;
return this;
}
/**
* ExternalRememberMe id representing the potential payer
* @return this
*/
@java.lang.SuppressWarnings("all")
public PotentialPayerInfo setExternalRememberMeId(final String externalRememberMeId) {
this.externalRememberMeId = externalRememberMeId;
return this;
}
/**
* Account Number representing the potential payer
* @return this
*/
@java.lang.SuppressWarnings("all")
public PotentialPayerInfo setAccountNumber(final String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Billing address of the potential payer.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PotentialPayerInfo setBillingAddress(final Address billingAddress) {
this.billingAddress = billingAddress;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PotentialPayerInfo)) return false;
final PotentialPayerInfo other = (PotentialPayerInfo) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$email = this.getEmail();
final java.lang.Object other$email = other.getEmail();
if (this$email == null ? other$email != null : !this$email.equals(other$email)) return false;
final java.lang.Object this$externalRememberMeId = this.getExternalRememberMeId();
final java.lang.Object other$externalRememberMeId = other.getExternalRememberMeId();
if (this$externalRememberMeId == null ? other$externalRememberMeId != null : !this$externalRememberMeId.equals(other$externalRememberMeId)) return false;
final java.lang.Object this$accountNumber = this.getAccountNumber();
final java.lang.Object other$accountNumber = other.getAccountNumber();
if (this$accountNumber == null ? other$accountNumber != null : !this$accountNumber.equals(other$accountNumber)) return false;
final java.lang.Object this$billingAddress = this.getBillingAddress();
final java.lang.Object other$billingAddress = other.getBillingAddress();
if (this$billingAddress == null ? other$billingAddress != null : !this$billingAddress.equals(other$billingAddress)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PotentialPayerInfo;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $email = this.getEmail();
result = result * PRIME + ($email == null ? 43 : $email.hashCode());
final java.lang.Object $externalRememberMeId = this.getExternalRememberMeId();
result = result * PRIME + ($externalRememberMeId == null ? 43 : $externalRememberMeId.hashCode());
final java.lang.Object $accountNumber = this.getAccountNumber();
result = result * PRIME + ($accountNumber == null ? 43 : $accountNumber.hashCode());
final java.lang.Object $billingAddress = this.getBillingAddress();
result = result * PRIME + ($billingAddress == null ? 43 : $billingAddress.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy