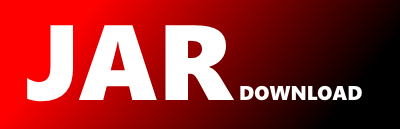
com.paypal.api.payments.PrivateLabelCard Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Tue Jan 31 13:36:37 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class PrivateLabelCard extends PayPalModel {
/**
* encrypted identifier of the private label card instrument.
*/
private String id;
/**
* last 4 digits of the card number.
*/
private String cardNumber;
/**
* Merchants providing private label store cards have associated issuer account. This value indicates encrypted account number of the associated issuer account.
*/
private String issuerId;
/**
* Merchants providing private label store cards have associated issuer account. This value indicates name on the issuer account.
*/
private String issuerName;
/**
* This value indicates URL to access PLCC program logo image
*/
private String imageKey;
/**
* Default Constructor
*/
public PrivateLabelCard() {
}
/**
* encrypted identifier of the private label card instrument.
*/
@java.lang.SuppressWarnings("all")
public String getId() {
return this.id;
}
/**
* last 4 digits of the card number.
*/
@java.lang.SuppressWarnings("all")
public String getCardNumber() {
return this.cardNumber;
}
/**
* Merchants providing private label store cards have associated issuer account. This value indicates encrypted account number of the associated issuer account.
*/
@java.lang.SuppressWarnings("all")
public String getIssuerId() {
return this.issuerId;
}
/**
* Merchants providing private label store cards have associated issuer account. This value indicates name on the issuer account.
*/
@java.lang.SuppressWarnings("all")
public String getIssuerName() {
return this.issuerName;
}
/**
* This value indicates URL to access PLCC program logo image
*/
@java.lang.SuppressWarnings("all")
public String getImageKey() {
return this.imageKey;
}
/**
* encrypted identifier of the private label card instrument.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PrivateLabelCard setId(final String id) {
this.id = id;
return this;
}
/**
* last 4 digits of the card number.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PrivateLabelCard setCardNumber(final String cardNumber) {
this.cardNumber = cardNumber;
return this;
}
/**
* Merchants providing private label store cards have associated issuer account. This value indicates encrypted account number of the associated issuer account.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PrivateLabelCard setIssuerId(final String issuerId) {
this.issuerId = issuerId;
return this;
}
/**
* Merchants providing private label store cards have associated issuer account. This value indicates name on the issuer account.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PrivateLabelCard setIssuerName(final String issuerName) {
this.issuerName = issuerName;
return this;
}
/**
* This value indicates URL to access PLCC program logo image
* @return this
*/
@java.lang.SuppressWarnings("all")
public PrivateLabelCard setImageKey(final String imageKey) {
this.imageKey = imageKey;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PrivateLabelCard)) return false;
final PrivateLabelCard other = (PrivateLabelCard) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$cardNumber = this.getCardNumber();
final java.lang.Object other$cardNumber = other.getCardNumber();
if (this$cardNumber == null ? other$cardNumber != null : !this$cardNumber.equals(other$cardNumber)) return false;
final java.lang.Object this$issuerId = this.getIssuerId();
final java.lang.Object other$issuerId = other.getIssuerId();
if (this$issuerId == null ? other$issuerId != null : !this$issuerId.equals(other$issuerId)) return false;
final java.lang.Object this$issuerName = this.getIssuerName();
final java.lang.Object other$issuerName = other.getIssuerName();
if (this$issuerName == null ? other$issuerName != null : !this$issuerName.equals(other$issuerName)) return false;
final java.lang.Object this$imageKey = this.getImageKey();
final java.lang.Object other$imageKey = other.getImageKey();
if (this$imageKey == null ? other$imageKey != null : !this$imageKey.equals(other$imageKey)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PrivateLabelCard;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $cardNumber = this.getCardNumber();
result = result * PRIME + ($cardNumber == null ? 43 : $cardNumber.hashCode());
final java.lang.Object $issuerId = this.getIssuerId();
result = result * PRIME + ($issuerId == null ? 43 : $issuerId.hashCode());
final java.lang.Object $issuerName = this.getIssuerName();
result = result * PRIME + ($issuerName == null ? 43 : $issuerName.hashCode());
final java.lang.Object $imageKey = this.getImageKey();
result = result * PRIME + ($imageKey == null ? 43 : $imageKey.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy