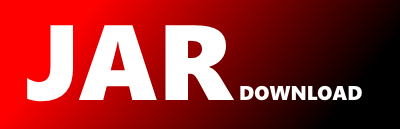
com.paypal.api.payments.Template Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Tue Jan 31 13:36:37 CST 2017
package com.paypal.api.payments;
import com.google.gson.annotations.SerializedName;
import com.paypal.base.rest.*;
import java.util.List;
public class Template extends PayPalResource {
/**
* Unique identifier id of the template.
*/
private String templateId;
/**
* Name of the template.
*/
private String name;
/**
* Indicates that this template is merchant's default. There can be only one template which can be a default.
*/
@SerializedName("default")
private Boolean isDefault;
/**
* Customized invoice data which is saved as template
*/
private TemplateData templateData;
/**
* Settings for each template
*/
private List settings;
/**
* Unit of measure for the template, possible values are Quantity, Hours, Amount.
*/
private String unitOfMeasure;
/**
* Indicates whether this is a custom template created by the merchant. Non custom templates are system generated
*/
private Boolean custom;
/**
* Default Constructor
*/
public Template() {
}
/**
* Retrieve the details for a particular template by passing the template ID to the request URI.
* @param apiContext
* {@link APIContext} used for the API call.
* @param templateId
* String
* @return Template
* @throws PayPalRESTException
*/
public static Template get(APIContext apiContext, String templateId) throws PayPalRESTException {
if (templateId == null) {
throw new IllegalArgumentException("templateId cannot be null");
}
Object[] parameters = new Object[] {templateId};
String pattern = "v1/invoicing/templates/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, Template.class);
}
/**
* Retrieves the template information of the merchant.
* @param apiContext
* {@link APIContext} used for the API call.
* @return Templates
* @throws PayPalRESTException
*/
public static Templates getAll(APIContext apiContext) throws PayPalRESTException {
String resourcePath = "v1/invoicing/templates";
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, Templates.class);
}
/**
* Delete a particular template by passing the template ID to the request URI.
* @param apiContext
* {@link APIContext} used for the API call.
* @return
* @throws PayPalRESTException
*/
public void delete(APIContext apiContext) throws PayPalRESTException {
if (this.getTemplateId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
apiContext.setMaskRequestId(true);
Object[] parameters = new Object[] {this.getTemplateId()};
String pattern = "v1/invoicing/templates/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
configureAndExecute(apiContext, HttpMethod.DELETE, resourcePath, payLoad, null);
}
/**
* Creates a template.
* @param apiContext
* {@link APIContext} used for the API call.
* @return Template
* @throws PayPalRESTException
*/
public Template create(APIContext apiContext) throws PayPalRESTException {
String resourcePath = "v1/invoicing/templates";
String payLoad = this.toJSON();
return configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, Template.class);
}
/**
* Update an existing template by passing the template ID to the request URI. In addition, pass a complete template object in the request JSON. Partial updates are not supported.
* @param apiContext
* {@link APIContext} used for the API call.
* Template
* @return Template
* @throws PayPalRESTException
*/
public Template update(APIContext apiContext) throws PayPalRESTException {
if (this.getTemplateId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
Object[] parameters = new Object[] {this.getTemplateId()};
String pattern = "v1/invoicing/templates/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = this.toJSON();
return configureAndExecute(apiContext, HttpMethod.PUT, resourcePath, payLoad, Template.class);
}
/**
* Unique identifier id of the template.
*/
@java.lang.SuppressWarnings("all")
public String getTemplateId() {
return this.templateId;
}
/**
* Name of the template.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* Indicates that this template is merchant's default. There can be only one template which can be a default.
*/
@java.lang.SuppressWarnings("all")
public Boolean getIsDefault() {
return this.isDefault;
}
/**
* Customized invoice data which is saved as template
*/
@java.lang.SuppressWarnings("all")
public TemplateData getTemplateData() {
return this.templateData;
}
/**
* Settings for each template
*/
@java.lang.SuppressWarnings("all")
public List getSettings() {
return this.settings;
}
/**
* Unit of measure for the template, possible values are Quantity, Hours, Amount.
*/
@java.lang.SuppressWarnings("all")
public String getUnitOfMeasure() {
return this.unitOfMeasure;
}
/**
* Indicates whether this is a custom template created by the merchant. Non custom templates are system generated
*/
@java.lang.SuppressWarnings("all")
public Boolean getCustom() {
return this.custom;
}
/**
* Unique identifier id of the template.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Template setTemplateId(final String templateId) {
this.templateId = templateId;
return this;
}
/**
* Name of the template.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Template setName(final String name) {
this.name = name;
return this;
}
/**
* Indicates that this template is merchant's default. There can be only one template which can be a default.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Template setIsDefault(final Boolean isDefault) {
this.isDefault = isDefault;
return this;
}
/**
* Customized invoice data which is saved as template
* @return this
*/
@java.lang.SuppressWarnings("all")
public Template setTemplateData(final TemplateData templateData) {
this.templateData = templateData;
return this;
}
/**
* Settings for each template
* @return this
*/
@java.lang.SuppressWarnings("all")
public Template setSettings(final List settings) {
this.settings = settings;
return this;
}
/**
* Unit of measure for the template, possible values are Quantity, Hours, Amount.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Template setUnitOfMeasure(final String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* Indicates whether this is a custom template created by the merchant. Non custom templates are system generated
* @return this
*/
@java.lang.SuppressWarnings("all")
public Template setCustom(final Boolean custom) {
this.custom = custom;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Template)) return false;
final Template other = (Template) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$templateId = this.getTemplateId();
final java.lang.Object other$templateId = other.getTemplateId();
if (this$templateId == null ? other$templateId != null : !this$templateId.equals(other$templateId)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$isDefault = this.getIsDefault();
final java.lang.Object other$isDefault = other.getIsDefault();
if (this$isDefault == null ? other$isDefault != null : !this$isDefault.equals(other$isDefault)) return false;
final java.lang.Object this$templateData = this.getTemplateData();
final java.lang.Object other$templateData = other.getTemplateData();
if (this$templateData == null ? other$templateData != null : !this$templateData.equals(other$templateData)) return false;
final java.lang.Object this$settings = this.getSettings();
final java.lang.Object other$settings = other.getSettings();
if (this$settings == null ? other$settings != null : !this$settings.equals(other$settings)) return false;
final java.lang.Object this$unitOfMeasure = this.getUnitOfMeasure();
final java.lang.Object other$unitOfMeasure = other.getUnitOfMeasure();
if (this$unitOfMeasure == null ? other$unitOfMeasure != null : !this$unitOfMeasure.equals(other$unitOfMeasure)) return false;
final java.lang.Object this$custom = this.getCustom();
final java.lang.Object other$custom = other.getCustom();
if (this$custom == null ? other$custom != null : !this$custom.equals(other$custom)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Template;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $templateId = this.getTemplateId();
result = result * PRIME + ($templateId == null ? 43 : $templateId.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $isDefault = this.getIsDefault();
result = result * PRIME + ($isDefault == null ? 43 : $isDefault.hashCode());
final java.lang.Object $templateData = this.getTemplateData();
result = result * PRIME + ($templateData == null ? 43 : $templateData.hashCode());
final java.lang.Object $settings = this.getSettings();
result = result * PRIME + ($settings == null ? 43 : $settings.hashCode());
final java.lang.Object $unitOfMeasure = this.getUnitOfMeasure();
result = result * PRIME + ($unitOfMeasure == null ? 43 : $unitOfMeasure.hashCode());
final java.lang.Object $custom = this.getCustom();
result = result * PRIME + ($custom == null ? 43 : $custom.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy