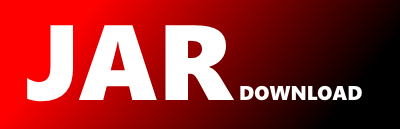
com.paypal.api.payments.WebProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Tue Jan 31 13:36:37 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.*;
import java.util.List;
public class WebProfile extends PayPalResource {
/**
* The unique ID of the web experience profile.
*/
private String id;
/**
* The web experience profile name. Unique for a specified merchant's profiles.
*/
private String name;
/**
* Indicates whether the profile persists for three hours or permanently. Set to `false` to persist the profile permanently. Set to `true` to persist the profile for three hours.
*/
private Boolean temporary;
/**
* Parameters for flow configuration.
*/
private FlowConfig flowConfig;
/**
* Parameters for input fields customization.
*/
private InputFields inputFields;
/**
* Parameters for style and presentation.
*/
private Presentation presentation;
/**
* Default Constructor
*/
public WebProfile() {
}
/**
* Parameterized Constructor
*/
public WebProfile(String name) {
this.name = name;
}
/**
* Creates a web experience profile. Pass the profile name and details in the JSON request body.
* @deprecated Please use {@link #create(APIContext)} instead.
* @param accessToken
* Access Token used for the API call.
* @return CreateProfileResponse
* @throws PayPalRESTException
*/
public CreateProfileResponse create(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return create(apiContext);
}
/**
* Creates a web experience profile. Pass the profile name and details in the JSON request body.
* @param apiContext
* {@link APIContext} used for the API call.
* @return CreateProfileResponse
* @throws PayPalRESTException
*/
public CreateProfileResponse create(APIContext apiContext) throws PayPalRESTException {
String resourcePath = "v1/payment-experience/web-profiles";
String payLoad = this.toJSON();
return configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, CreateProfileResponse.class);
}
/**
* Updates a web experience profile. Pass the ID of the profile to the request URI and pass the profile details in the JSON request body. If your request omits any profile detail fields, the operation removes the previously set values for those fields.
* @deprecated Please use {@link #update(APIContext)} instead.
* @param accessToken
* Access Token used for the API call.
* @return
* @throws PayPalRESTException
*/
public void update(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
update(apiContext);
}
/**
* Updates a web experience profile. Pass the ID of the profile to the request URI and pass the profile details in the JSON request body. If your request omits any profile detail fields, the operation removes the previously set values for those fields.
* @param apiContext
* {@link APIContext} used for the API call.
* @return
* @throws PayPalRESTException
*/
public void update(APIContext apiContext) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payment-experience/web-profiles/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = this.toJSON();
configureAndExecute(apiContext, HttpMethod.PUT, resourcePath, payLoad, null);
return;
}
/**
* Partially-updates a web experience profile. Pass the profile ID to the request URI. Pass a patch object with the operation, path of the profile location to update, and, if needed, a new value to complete the operation in the JSON request body.
* @deprecated Please use {@link #partialUpdate(APIContext, PatchRequest)} instead.
*
* @param accessToken
* Access Token used for the API call.
* @param patchRequest
* PatchRequest
* @return
* @throws PayPalRESTException
*/
public void partialUpdate(String accessToken, PatchRequest patchRequest) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
partialUpdate(apiContext, patchRequest);
}
/**
* Partially-updates a web experience profile. Pass the profile ID to the request URI. Pass a patch object with the operation, path of the profile location to update, and, if needed, a new value to complete the operation in the JSON request body.
* @param apiContext
* {@link APIContext} used for the API call.
* @param patchRequest
* PatchRequest
* @return
* @throws PayPalRESTException
*/
public void partialUpdate(APIContext apiContext, PatchRequest patchRequest) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (patchRequest == null) {
throw new IllegalArgumentException("patchRequest cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payment-experience/web-profiles/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = patchRequest.toJSON();
configureAndExecute(apiContext, HttpMethod.PATCH, resourcePath, payLoad, null);
return;
}
/**
* Shows details for a web experience profile, by ID.
* @deprecated Please use {@link #get(APIContext, String)} instead.
* @param accessToken
* Access Token used for the API call.
* @param profileId
* String
* @return WebProfile
* @throws PayPalRESTException
*/
public static WebProfile get(String accessToken, String profileId) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return get(apiContext, profileId);
}
/**
* Shows details for a web experience profile, by ID.
* @param apiContext
* {@link APIContext} used for the API call.
* @param profileId
* String
* @return WebProfile
* @throws PayPalRESTException
*/
public static WebProfile get(APIContext apiContext, String profileId) throws PayPalRESTException {
if (profileId == null) {
throw new IllegalArgumentException("profileId cannot be null");
}
Object[] parameters = new Object[] {profileId};
String pattern = "v1/payment-experience/web-profiles/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, WebProfile.class);
}
/**
* Lists all web experience profiles for a merchant or subject.
* @deprecated Please use {@link #getList(APIContext)} instead.
* @param accessToken
* Access Token used for the API call.
* @return WebProfileList
* @throws PayPalRESTException
*/
public static List getList(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return getList(apiContext);
}
/**
* Lists all web experience profiles for a merchant or subject.
* @param apiContext
* {@link APIContext} used for the API call.
* @return WebProfileList
* @throws PayPalRESTException
*/
public static List getList(APIContext apiContext) throws PayPalRESTException {
String resourcePath = "v1/payment-experience/web-profiles";
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, WebProfileList.class);
}
/**
* Deletes a web experience profile, by ID.
* @deprecated Please use {@link #delete(APIContext)} instead.
* @param accessToken
* Access Token used for the API call.
* @return
* @throws PayPalRESTException
*/
public void delete(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
delete(apiContext);
}
/**
* Deletes a web experience profile, by ID.
* @param apiContext
* {@link APIContext} used for the API call.
* @return
* @throws PayPalRESTException
*/
public void delete(APIContext apiContext) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payment-experience/web-profiles/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
configureAndExecute(apiContext, HttpMethod.DELETE, resourcePath, payLoad, null);
}
/**
* The unique ID of the web experience profile.
*/
@java.lang.SuppressWarnings("all")
public String getId() {
return this.id;
}
/**
* The web experience profile name. Unique for a specified merchant's profiles.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* Indicates whether the profile persists for three hours or permanently. Set to `false` to persist the profile permanently. Set to `true` to persist the profile for three hours.
*/
@java.lang.SuppressWarnings("all")
public Boolean getTemporary() {
return this.temporary;
}
/**
* Parameters for flow configuration.
*/
@java.lang.SuppressWarnings("all")
public FlowConfig getFlowConfig() {
return this.flowConfig;
}
/**
* Parameters for input fields customization.
*/
@java.lang.SuppressWarnings("all")
public InputFields getInputFields() {
return this.inputFields;
}
/**
* Parameters for style and presentation.
*/
@java.lang.SuppressWarnings("all")
public Presentation getPresentation() {
return this.presentation;
}
/**
* The unique ID of the web experience profile.
* @return this
*/
@java.lang.SuppressWarnings("all")
public WebProfile setId(final String id) {
this.id = id;
return this;
}
/**
* The web experience profile name. Unique for a specified merchant's profiles.
* @return this
*/
@java.lang.SuppressWarnings("all")
public WebProfile setName(final String name) {
this.name = name;
return this;
}
/**
* Indicates whether the profile persists for three hours or permanently. Set to `false` to persist the profile permanently. Set to `true` to persist the profile for three hours.
* @return this
*/
@java.lang.SuppressWarnings("all")
public WebProfile setTemporary(final Boolean temporary) {
this.temporary = temporary;
return this;
}
/**
* Parameters for flow configuration.
* @return this
*/
@java.lang.SuppressWarnings("all")
public WebProfile setFlowConfig(final FlowConfig flowConfig) {
this.flowConfig = flowConfig;
return this;
}
/**
* Parameters for input fields customization.
* @return this
*/
@java.lang.SuppressWarnings("all")
public WebProfile setInputFields(final InputFields inputFields) {
this.inputFields = inputFields;
return this;
}
/**
* Parameters for style and presentation.
* @return this
*/
@java.lang.SuppressWarnings("all")
public WebProfile setPresentation(final Presentation presentation) {
this.presentation = presentation;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof WebProfile)) return false;
final WebProfile other = (WebProfile) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$temporary = this.getTemporary();
final java.lang.Object other$temporary = other.getTemporary();
if (this$temporary == null ? other$temporary != null : !this$temporary.equals(other$temporary)) return false;
final java.lang.Object this$flowConfig = this.getFlowConfig();
final java.lang.Object other$flowConfig = other.getFlowConfig();
if (this$flowConfig == null ? other$flowConfig != null : !this$flowConfig.equals(other$flowConfig)) return false;
final java.lang.Object this$inputFields = this.getInputFields();
final java.lang.Object other$inputFields = other.getInputFields();
if (this$inputFields == null ? other$inputFields != null : !this$inputFields.equals(other$inputFields)) return false;
final java.lang.Object this$presentation = this.getPresentation();
final java.lang.Object other$presentation = other.getPresentation();
if (this$presentation == null ? other$presentation != null : !this$presentation.equals(other$presentation)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof WebProfile;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $temporary = this.getTemporary();
result = result * PRIME + ($temporary == null ? 43 : $temporary.hashCode());
final java.lang.Object $flowConfig = this.getFlowConfig();
result = result * PRIME + ($flowConfig == null ? 43 : $flowConfig.hashCode());
final java.lang.Object $inputFields = this.getInputFields();
result = result * PRIME + ($inputFields == null ? 43 : $inputFields.hashCode());
final java.lang.Object $presentation = this.getPresentation();
result = result * PRIME + ($presentation == null ? 43 : $presentation.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy