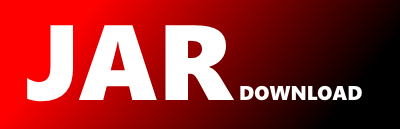
com.paypal.base.SDKUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
package com.paypal.base;
import com.paypal.base.rest.PayPalRESTException;
import java.util.*;
import java.util.Map.Entry;
/**
* SDKUtil class holds utility methods for processing data transformation
*
*/
public final class SDKUtil {
private SDKUtil() {
}
/**
* Constructs a Map from a {@link Properties} object by
* combining the default values. See {@link ConfigManager} for default
* values
*
* @param properties
* Input {@link Properties}
* @return Map
*/
public static Map constructMap(Properties properties) {
Properties combinedProperties = ConfigManager.combineDefaultProperties(properties);
Map propsMap = new HashMap();
// Since the default properties are only searchable
Enumeration> keys = combinedProperties.propertyNames();
while (keys.hasMoreElements()) {
String key = keys.nextElement().toString().trim();
String value = combinedProperties.getProperty(key).trim();
propsMap.put(key, value);
}
return propsMap;
}
/**
* Combines some {@link Map} with default values. See {@link ConfigManager}
* for default values.
*
* @param receivedMap
* {@link Map} used to combine with Default {@link Map}
* @return Combined {@link Map}
*/
public static Map combineDefaultMap(Map receivedMap) {
return combineMap(receivedMap, ConfigManager.getDefaultSDKMap());
}
public static Map combineMap(Map highMap, Map lowMap) {
lowMap = lowMap != null ? lowMap : new HashMap();
highMap = highMap != null ? highMap : new HashMap();
lowMap.putAll(highMap);
return lowMap;
}
/**
* Utility method to validate if the key exists in the provided map, and
* returns string value of the object
*
* @param map
* Map of String based key and values
* @param key
* object to be found in the key
* @return String value of the key
* @throws PayPalRESTException
*/
public static String validateAndGet(Map map, String key) throws PayPalRESTException {
if (map == null || key == null) {
throw new PayPalRESTException("Map or Key cannot be null");
}
String value = map.get(key);
if (value == null || value.equals("")) {
for (Iterator> itemIter = map.entrySet().iterator(); itemIter.hasNext();) {
Entry entry = itemIter.next();
if (entry.getKey().equalsIgnoreCase(key)) {
value = entry.getValue();
break;
}
}
if (value == null || value.equals("")) {
throw new PayPalRESTException(key + " cannot be null");
}
}
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy