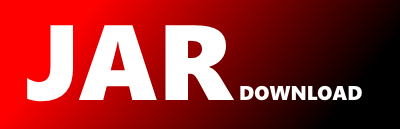
com.paypal.api.payments.PaymentHistory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy