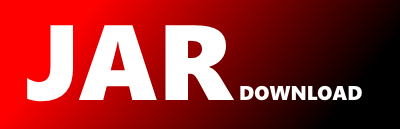
com.paypal.springboot.resteasy.JaxrsApplicationScanner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resteasy-spring-boot-starter Show documentation
Show all versions of resteasy-spring-boot-starter Show documentation
A Spring Boot starter for RESTEasy
package com.paypal.springboot.resteasy;
import org.apache.commons.io.FilenameUtils;
import org.reflections.Reflections;
import org.reflections.scanners.SubTypesScanner;
import org.reflections.util.ClasspathHelper;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.ws.rs.core.Application;
import java.io.File;
import java.net.URL;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
/**
* Helper class to scan the classpath searching for
* JAX-RS Application sub-classes
*
* @author Fabio Carvalho ([email protected] or [email protected])
*/
public abstract class JaxrsApplicationScanner {
private static final Logger logger = LoggerFactory.getLogger(JaxrsApplicationScanner.class);
private static Set> applications;
public static Set> getApplications() {
if(applications == null) {
applications = findJaxrsApplicationClasses();
}
return applications;
}
/*
* Scan the classpath looking for JAX-RS Application sub-classes
*/
private static Set> findJaxrsApplicationClasses() {
logger.info("Scanning classpath to find JAX-RS Application classes");
final Collection systemPropertyURLs = ClasspathHelper.forJavaClassPath();
final Collection classLoaderURLs = ClasspathHelper.forClassLoader();
Set classpathURLs = new HashSet();
copyValidClasspathEntries(systemPropertyURLs, classpathURLs);
copyValidClasspathEntries(classLoaderURLs, classpathURLs);
logger.debug("Classpath URLs to be scanned: " + classpathURLs);
Reflections reflections = new Reflections(classpathURLs, new SubTypesScanner());
return reflections.getSubTypesOf(Application.class);
}
/*
* Copy all entries that are a JAR file or a directory
*/
private static void copyValidClasspathEntries(Collection source, Set destination) {
String fileName;
boolean isJarFile;
boolean isDirectory;
for (URL url : source) {
if(destination.contains(url)) {
continue;
}
fileName = url.getFile();
isJarFile = FilenameUtils.isExtension(fileName, "jar");
isDirectory = new File(fileName).isDirectory();
if (isJarFile || isDirectory) {
destination.add(url);
} else if (logger.isDebugEnabled()) {
logger.debug("Ignored classpath entry: " + fileName);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy