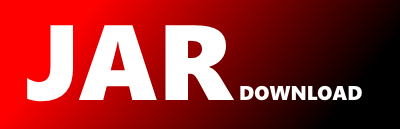
com.pcloud.networking.api.ApiComposer Maven / Gradle / Ivy
/*
* Copyright (c) 2017 pCloud AG
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.pcloud.networking.api;
import com.pcloud.networking.client.PCloudAPIClient;
import com.pcloud.networking.serialization.Transformer;
import java.lang.annotation.Annotation;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* Composes implementations for interfaces in which you have provided methods that describe your network calls
*
* @see PCloudAPIClient
* @see ResponseInterceptor
*/
@SuppressWarnings({"WeakerAccess", "unused"})
public class ApiComposer {
/**
* Creates and returns a {@linkplain Builder} to build the {@linkplain ApiComposer} with
*
* @return A new instance of a {@linkplain Builder} to build the {@linkplain ApiComposer} with
*/
public static Builder create() {
return new Builder();
}
/**
* Internal list of {@linkplain ApiMethod.Factory} factories
*
* Holds the list of available {@linkplain ApiMethod.Factory} implementations.
*
* Note that the order of elements is important, please consider it before modification.
*/
private static final List BUILT_IN_API_METHOD_FACTORIES = Arrays.asList(
MultiCallWrappedApiMethod.FACTORY,
CallWrappedApiMethod.FACTORY,
DirectApiMethod.FACTORY
);
/**
* The built-in set of {@linkplain CallAdapter.Factory} implementations
*/
private static final List BUILT_IN_CALL_ADAPTER_FACTORIES = Arrays.asList(
CallWrappedCallAdapter.FACTORY,
DirectCallAdapter.FACTORY
);
private final PCloudAPIClient apiClient;
private final Transformer transformer;
private final List interceptors;
private final boolean loadEagerly;
private final Map> apiMethodsCache = new ConcurrentHashMap<>();
private final List factories = new ArrayList<>(BUILT_IN_API_METHOD_FACTORIES);
private final List callAdapterFactories = new ArrayList<>(BUILT_IN_CALL_ADAPTER_FACTORIES);
private ApiComposer(Builder builder) {
if (builder.apiClient == null) {
throw new IllegalArgumentException("PCloudAPIClient instance cannot be null.");
}
this.apiClient = builder.apiClient;
this.transformer = builder.transformer != null ? builder.transformer : Transformer.create().build();
this.interceptors = new ArrayList<>(builder.interceptors);
this.callAdapterFactories.addAll(builder.callAdapterFactories);
this.loadEagerly = builder.loadEagerly;
}
/**
* Returns the {@linkplain Transformer} you provided in the {@linkplain Builder}
*
* @return The {@linkplain Transformer} you provided in the {@linkplain Builder}
*/
public Transformer transformer() {
return transformer;
}
/**
* Returns the {@linkplain PCloudAPIClient} you provided in the {@linkplain Builder}
*
* @return The {@linkplain PCloudAPIClient} you provided in the {@linkplain Builder}
*/
public PCloudAPIClient apiClient() {
return apiClient;
}
/**
* Returns a {@linkplain List} of all the {@linkplain ResponseInterceptor}
* objects you provided in the {@linkplain Builder}
*
* @return A {@linkplain List} of all the {@linkplain ResponseInterceptor}
* objects you provided in the {@linkplain Builder}
*/
public List interceptors() {
return interceptors;
}
/**
* Composes an instance of the java interface which houses the network call methods
*
* Note that you should provide an interface which does not extend anything or else this wont work!
*
* @param apiType The class of the java interface in which you have
* written the methods to represent the network calls
* @param The generic type of the returned instance
* @return An instance of a class of the same generic type as the class you provided as an argument
* implementing the interface in which you have written methods to represent your network calls
* @throws RuntimeException if {@linkplain #loadEagerly} is set to true and if the instantiation fails
*/
@SuppressWarnings("unchecked")
public T compose(Class apiType) {
validateApiInterface(apiType);
if (loadEagerly) {
Method[] methods = apiType.getDeclaredMethods();
for (Method method : methods) {
loadApiMethod(method);
}
}
return (T) Proxy.newProxyInstance(apiType.getClassLoader(), new Class>[]{apiType},
new InvocationHandler() {
@Override
public Object invoke(Object proxy, java.lang.reflect.Method method, Object[] args)
throws Throwable {
// If the method is a method from Object then defer to normal invocation.
if (method.getDeclaringClass() == Object.class) {
return method.invoke(this, args);
}
if (args == null) {
args = ApiMethod.EMPTY_ARGS;
}
ApiMethod