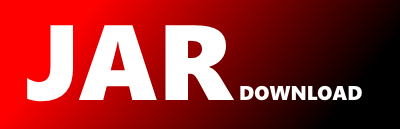
com.pdftools.HttpClientHandler Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: HttpClientHandler.java
*
* Description: PDFTOOLS HttpClientHandler Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The handler and options for communication to remote server
*
* This class can be used to configure HTTP and HTTPS communication.
*
* Also see {@link Sdk#getProxy } for the product wide proxy configuration.
*
* For HTTPS (SSL/TLS) communication, the server certificate's trustworthiness is verified using the system's default trust store (CA certificate store).
* If the server certificate's trustworthiness cannot be determined, the connection to the server is aborted.
*
* The default trust store is:
*
* -
*
* Windows:
*
* The Windows certificate store for "Trusted Root Certification Authorities" is used.
* You can manually install the root certificate of a private CA on a computer by using the {@code CertMgr} tool.
* The certificate store is only available if the user profile has been loaded.
*
* -
*
* Linux:
*
* The certificates available in {@code CAfile} and {@code CApath} are trusted:
*
* -
*
* {@code CAfile}:
*
* The file can contain a concatenated sequence of CA certificates in PEM format.
*
* The SDK searches for the file at the following locations:
*
* -
* The file of your local OpenSSL installation (if {@code libssl.so} is found), or
* -
* the environment variable {@code SSL_CERT_FILE}, or
* -
* the default location {@code /etc/ssl/cert.pem}.
*
* -
*
* {@code CApath}:
*
* A directory containing CA certificates in PEM format.
* The files are looked up by the CA subject name hash value, e.g. {@code 9d66eef0.0}.
*
* The SDK searches for the directory at the following locations:
*
* -
* The directory of your local OpenSSL installation (if {@code libssl.so} is found), or
* -
* the environment variable {@code SSL_CERT_DIR}, or
* -
* the default location {@code /etc/ssl/certs/}.
*
*
* -
*
* macOS:
*
* The trusted certificates from the macOS keychain are used.
* You can manually install the root certificate of a private CA by dragging the certificate file onto the Keychain Access app.
*
*
*
* You can add more certificates to the trust store using {@link HttpClientHandler#addTrustedCertificate }.
*
* Instances of this class can be used in multiple threads concurrently, as long as they are not modified concurrently.
*
*/
public class HttpClientHandler extends NativeObject
{
protected HttpClientHandler(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static HttpClientHandler createDynamicObject(long handle)
{
return new HttpClientHandler(handle);
}
/**
*
* The default values of newly created objects are not copied from the default handler {@link Sdk#getHttpClientHandler },
* but are as described in this documentation.
*/
public HttpClientHandler()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* Set the SSL/TLS client certificate as PFX (PKCS#12) archive
* The file must contain the certificate itself, all certificates of the trust chain, and the private key.
* @param archive
* The SSL client certificate in PKCS#12 format (.p12, .pfx)
* @param password
* The password required to decrypt the private key of the archive
*
* @throws com.pdftools.CorruptException The PFX (PKCS#12) archive is corrupt and cannot be read.
* @throws com.pdftools.PasswordException The password is invalid.
* @throws IllegalArgumentException The PFX (PKCS#12) archive is incomplete.
* @throws IllegalArgumentException if {@code archive} is {@code null}
*/
public void setClientCertificate(com.pdftools.sys.Stream archive, String password)
throws
com.pdftools.CorruptException,
com.pdftools.PasswordException
{
if (archive == null)
throw new IllegalArgumentException("Argument 'archive' must not be null.", new NullPointerException("'archive'"));
boolean retVal = setClientCertificateNative(getHandle(), archive, password);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
case 16: throw new com.pdftools.CorruptException(getLastErrorMessage());
case 17: throw new com.pdftools.PasswordException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* Set the SSL/TLS client certificate and private key
* The file must contain the certificate and its private key.
* It is also recommended to include all certificates of the trust chain.
* @param cert
* The certificate may be in either PEM (.pem, ASCII text) or DER (.cer, binary) form.
* @param key
* The encrypted private key of the certificate must be in PEM (ASCII text) form (.pem).
* @param password
* The password required to decrypt the private key.
*
* @throws com.pdftools.PasswordException The password is invalid.
* @throws com.pdftools.CorruptException The certificate or key cannot be read.
* @throws IllegalArgumentException if {@code cert} is {@code null}
* @throws IllegalArgumentException if {@code key} is {@code null}
*/
public void setClientCertificateAndKey(com.pdftools.sys.Stream cert, com.pdftools.sys.Stream key, String password)
throws
com.pdftools.CorruptException,
com.pdftools.PasswordException
{
if (cert == null)
throw new IllegalArgumentException("Argument 'cert' must not be null.", new NullPointerException("'cert'"));
if (key == null)
throw new IllegalArgumentException("Argument 'key' must not be null.", new NullPointerException("'key'"));
boolean retVal = setClientCertificateAndKeyNative(getHandle(), cert, key, password);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 16: throw new com.pdftools.CorruptException(getLastErrorMessage());
case 17: throw new com.pdftools.PasswordException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* Add a certificate to the trust store
* Add a certificate to the trust store of this {@code HttpClientHandler} instance.
* The certificates in the trust store are used to verify the certificate of the SSL/TLS server (see {@link HttpClientHandler }).
* You should add trusted certification authorities (Root CA) certificates to the trust store.
* However, you can also add server certificates (e.g. self-signed certificates) and intermediate CA certificates.
* @param cert
* The certificate may be in either PEM (.pem, ASCII text) or DER (.cer, binary) form.
*
* @throws com.pdftools.CorruptException The certificate cannot be read.
* @throws IllegalArgumentException if {@code cert} is {@code null}
*/
public void addTrustedCertificate(com.pdftools.sys.Stream cert)
throws
com.pdftools.CorruptException
{
if (cert == null)
throw new IllegalArgumentException("Argument 'cert' must not be null.", new NullPointerException("'cert'"));
boolean retVal = addTrustedCertificateNative(getHandle(), cert);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 16: throw new com.pdftools.CorruptException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* Verify the server certificate for SSL/TLS (Getter)
*
* If {@code true} the server certificate's trustworthiness is verified.
* If the verification process fails, the handshake is immediately terminated and the connection is aborted.
* The verification requires a trust store; otherwise, verification always fails.
*
* Default: {@code true}
*/
public boolean getSslVerifyServerCertificate()
{
boolean retVal = getSslVerifyServerCertificateNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Verify the server certificate for SSL/TLS (Setter)
*
* If {@code true} the server certificate's trustworthiness is verified.
* If the verification process fails, the handshake is immediately terminated and the connection is aborted.
* The verification requires a trust store; otherwise, verification always fails.
*
* Default: {@code true}
*/
public void setSslVerifyServerCertificate(boolean value)
{
boolean retVal = setSslVerifyServerCertificateNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
private static native long newNative();
private native boolean setClientCertificateNative(long handle, com.pdftools.sys.Stream archive, String password);
private native boolean setClientCertificateAndKeyNative(long handle, com.pdftools.sys.Stream cert, com.pdftools.sys.Stream key, String password);
private native boolean addTrustedCertificateNative(long handle, com.pdftools.sys.Stream cert);
private native boolean getSslVerifyServerCertificateNative(long handle);
private native boolean setSslVerifyServerCertificateNative(long handle, boolean value);
}