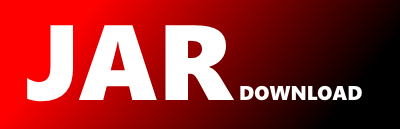
com.pdftools.MetadataDictionary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
The newest version!
/****************************************************************************
*
* File: MetadataDictionary.java
*
* Description: PDFTOOLS MetadataDictionary Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
import java.util.AbstractSet;
import java.util.Collection;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
/**
*
*/
public class MetadataDictionary extends NativeObject implements Map
{
protected MetadataDictionary(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static MetadataDictionary createDynamicObject(long handle)
{
return new MetadataDictionary(handle);
}
/**
*
*/
public MetadataDictionary()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
private static native long newNative();
/////////////////////////////////////////////////////
// Map implementation
/////////////////////////////////////////////////////
@Override
public void putAll(Map extends String, ? extends String> arg0)
{
if (arg0 == null)
throw new NullPointerException("'arg0'");
for (Entry extends String, ? extends String> e : arg0.entrySet())
put(e.getKey(), e.getValue());
}
@SuppressWarnings("unchecked")
@Override
public boolean containsKey(Object key)
{
if (key == null)
throw new NullPointerException("'key'");
return getIt((String)key) >= 0;
}
@Override
public boolean containsValue(Object value)
{
for (String val : values())
if (value == null ? val == null : value.equals(val))
return true;
return false;
}
@SuppressWarnings("unchecked")
@Override
public String get(Object key)
{
if (key == null)
throw new NullPointerException("'key'");
int it = getIt((String)key);
return getValue(it);
}
@Override
public Set> entrySet()
{
return new MetadataDictionaryEntrySet(this);
}
@Override
public boolean isEmpty()
{
return getBegin() == getEnd();
}
@Override
public Set keySet()
{
return new KeySet(this);
}
@SuppressWarnings("unchecked")
@Override
public String remove(Object key)
{
if (key == null)
throw new NullPointerException("'key'");
int it = getIt((String)key);
if (it == -1)
return null;
String value = getValue(it);
remove(it);
return value;
}
@Override
public Collection values()
{
return new ValueCollection(this);
}
@Override
public int size()
{
int size = size(getHandle());
if (size == -1)
throwLastRuntimeException(false);
return size;
}
@Override
public void clear()
{
if(!clear(getHandle()))
throwLastRuntimeException(false);
}
int getIt(String key)
{
if (key == null)
throw new NullPointerException("'key'");
int it = getIt(getHandle(), key);
if (it == -1 && getLastErrorCode() != 5)
throwLastRuntimeException(false);
return it;
}
int getBegin()
{
int retVal = getBegin(getHandle());
if (retVal == -1)
throwLastRuntimeException(false);
return retVal;
}
int getEnd()
{
int retVal = getEnd(getHandle());
if (retVal == -1)
throwLastRuntimeException(false);
return retVal;
}
int getNext(int it)
{
int retVal = getNext(getHandle(), it);
if (retVal == -1)
throwLastRuntimeException(false);
return retVal;
}
String getKey(int it)
{
String key = getKey(getHandle(), it);
if(key == null)
throwLastRuntimeException(false);
return key;
}
void remove(int it)
{
if(!remove(getHandle(), it))
throwLastRuntimeException(false);
}
@Override
public String put(String key, String value)
{
if (key == null)
throw new NullPointerException("'key'");
long handle = getHandle();
int it = getIt(key);
String prev = it >= 0 ? getValue(handle, it) : null;
if(!put(handle, key, value))
throwLastRuntimeException(false);
return prev;
}
String getValue(int it)
{
String retValue = getValue(getHandle(), it);
if (retValue == null)
{
throwLastRuntimeException();
return null;
}
return retValue;
}
void setValue(int it, String value)
{
if(!setValue(getHandle(), it, value))
throwLastRuntimeException(false);
}
private native int size(long handle);
private native boolean clear(long handle);
private native int getIt(long handle, String key);
private native int getBegin(long handle);
private native int getEnd(long handle);
private native String getKey(long handle, int it);
private native int getNext(long handle, int it);
private native boolean remove(long handle, int it);
private native boolean put(long handle, String key, String value);
private native String getValue(long handle, int it);
private native boolean setValue(long handle, int it, String value);
}
class MetadataDictionaryEntry implements Entry
{
public MetadataDictionaryEntry(MetadataDictionary map, int it)
{
this.map = map;
this.it = it;
}
@Override
public String getKey()
{
return map.getKey(it);
}
@Override
public String getValue()
{
return map.getValue(it);
}
@Override
public String setValue(String value)
{
String prev = map.getValue(it);
map.setValue(it, value);
return prev;
}
MetadataDictionary map;
int it;
}
class MetadataDictionaryEntryIterator implements Iterator>
{
public MetadataDictionaryEntryIterator(MetadataDictionary map)
{
this.map = map;
this.it = map.getBegin();
}
@Override
public boolean hasNext()
{
return it != map.getEnd();
}
@Override
public Entry next()
{
Entry entry = new MetadataDictionaryEntry(map, it);
it = map.getNext(it);
return entry;
}
@Override
public void remove()
{
map.remove(it);
}
MetadataDictionary map;
int it;
}
class MetadataDictionaryEntrySet extends AbstractSet>
{
public MetadataDictionaryEntrySet(MetadataDictionary map)
{
this.map = map;
}
/**
* Not supported.
*/
@Override
public boolean remove(Object o)
{
throw new UnsupportedOperationException();
}
@Override
public void clear()
{
map.clear();
}
/**
* Not supported.
*/
@Override
public boolean contains(Object object)
{
throw new UnsupportedOperationException();
}
@Override
public Iterator> iterator()
{
return new MetadataDictionaryEntryIterator(map);
}
@Override
public int size()
{
return map.size();
}
private MetadataDictionary map;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy