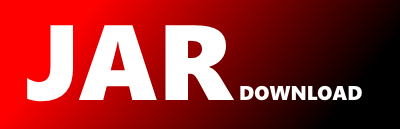
com.pdftools.Sdk Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: Sdk.java
*
* Description: PDFTOOLS Sdk Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* SDK initialization and product information
*/
public class Sdk extends NativeObject
{
protected Sdk(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Sdk createDynamicObject(long handle)
{
return new Sdk(handle);
}
/**
* Initialize the product and the license key.
* Before calling any of the other functions of the SDK, a license key must be set by calling this method.
* For licensing questions, contact [email protected].
* @param license
* The license key.
* The format of the license key is {@code ""}
*
* @throws com.pdftools.UnknownFormatException The format (version) of the {@link com.pdftools.Sdk#initialize license} argument is unknown.
* @throws com.pdftools.CorruptException The {@link com.pdftools.Sdk#initialize license} argument is not a correct license key.
* @throws com.pdftools.LicenseException The {@link com.pdftools.Sdk#initialize license} argument can be read but the license check failed.
* @throws com.pdftools.HttpException A network error occurred.
* @throws IllegalArgumentException if {@code license} is {@code null}
*/
public static void initialize(String license)
throws
com.pdftools.LicenseException,
com.pdftools.UnknownFormatException,
com.pdftools.CorruptException,
com.pdftools.HttpException
{
if (license == null)
throw new IllegalArgumentException("Argument 'license' must not be null.", new NullPointerException("'license'"));
initialize(license, null);
}
/**
* Initialize the product and the license key.
* Before calling any of the other functions of the SDK, a license key must be set by calling this method.
* For licensing questions, contact [email protected].
* @param license
* The license key.
* The format of the license key is {@code ""}
* @param producerSuffix
* If neither {@code null} nor empty, this string is appended to the producer string
* within metadata of PDF output documents (see {@link Sdk#getProducerFullName }).
*
* @throws com.pdftools.UnknownFormatException The format (version) of the {@link com.pdftools.Sdk#initialize license} argument is unknown.
* @throws com.pdftools.CorruptException The {@link com.pdftools.Sdk#initialize license} argument is not a correct license key.
* @throws com.pdftools.LicenseException The {@link com.pdftools.Sdk#initialize license} argument can be read but the license check failed.
* @throws com.pdftools.HttpException A network error occurred.
* @throws IllegalArgumentException if {@code license} is {@code null}
*/
public static void initialize(String license, String producerSuffix)
throws
com.pdftools.LicenseException,
com.pdftools.UnknownFormatException,
com.pdftools.CorruptException,
com.pdftools.HttpException
{
if (license == null)
throw new IllegalArgumentException("Argument 'license' must not be null.", new NullPointerException("'license'"));
boolean retVal = initializeNative(license, producerSuffix);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 12: throw new com.pdftools.LicenseException(getLastErrorMessage());
case 15: throw new com.pdftools.UnknownFormatException(getLastErrorMessage());
case 16: throw new com.pdftools.CorruptException(getLastErrorMessage());
case 24: throw new com.pdftools.HttpException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* Add custom font directory
* @param directory
* The path of the directory which contains additional font files to be considered during processing.
*
* @throws com.pdftools.NotFoundException The given directory path does not exist.
* @throws IllegalArgumentException if {@code directory} is {@code null}
*/
public static void addFontDirectory(String directory)
throws
com.pdftools.NotFoundException
{
if (directory == null)
throw new IllegalArgumentException("Argument 'directory' must not be null.", new NullPointerException("'directory'"));
boolean retVal = addFontDirectoryNative(directory);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 5: throw new com.pdftools.NotFoundException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The version of the SDK (Getter)
*
*/
public static String getVersion()
{
String retVal = getVersionNative();
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The suffix for the producer (Setter)
* Suffix that is appended to the producer string within metadata of PDF output documents (see {@link Sdk#getProducerFullName }).
*/
public static void setProducerSuffix(String value)
{
boolean retVal = setProducerSuffixNative(value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* The producer string that is set within the metadata of PDF output documents (Getter)
* The producer string depends on the license key and producer suffix set in {@link Sdk#initialize }.
*/
public static String getProducerFullName()
{
String retVal = getProducerFullNameNative();
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Proxy to use for all communication to remote servers (Getter)
*
* The SDK can use a proxy for all HTTP and HTTPS communication.
*
* The default is {@code null}, i.e. no proxy is used.
* Otherwise the property’s value must be a URI with the following elements:
*
* {@code http[s]://[‹user›[:‹password›]@]‹host›[:‹port›]}
*
* Where:
*
* -
* {@code http/https}: Protocol for connection to proxy.
* -
* {@code ‹user›:‹password›} (optional): Credentials for connection to proxy (basic authorization).
* -
* {@code ‹host›}: Hostname of proxy.
* -
* {@code ‹port›}: Port for connection to proxy.
*
*
* Example: {@code "http://myproxy:8080"}
*
* For SSL/TLS connections, e.g. to a signature service, the proxy must allow the {@code HTTP CONNECT} request to the remote server.
*
*/
public static java.net.URI getProxy()
{
String retVal = getProxyNative();
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
return null;
}
return java.net.URI.create(retVal);
}
/**
* Proxy to use for all communication to remote servers (Setter)
*
* The SDK can use a proxy for all HTTP and HTTPS communication.
*
* The default is {@code null}, i.e. no proxy is used.
* Otherwise the property’s value must be a URI with the following elements:
*
* {@code http[s]://[‹user›[:‹password›]@]‹host›[:‹port›]}
*
* Where:
*
* -
* {@code http/https}: Protocol for connection to proxy.
* -
* {@code ‹user›:‹password›} (optional): Credentials for connection to proxy (basic authorization).
* -
* {@code ‹host›}: Hostname of proxy.
* -
* {@code ‹port›}: Port for connection to proxy.
*
*
* Example: {@code "http://myproxy:8080"}
*
* For SSL/TLS connections, e.g. to a signature service, the proxy must allow the {@code HTTP CONNECT} request to the remote server.
*
*/
public static void setProxy(java.net.URI value)
{
boolean retVal = setProxyNative(value != null ? value.toString() : null);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* The default handler for communication to remote servers (Getter)
* This instance is used wherever there is no dedicated HTTP client handler parameter.
*/
public static com.pdftools.HttpClientHandler getHttpClientHandler()
{
long retHandle = getHttpClientHandlerNative();
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.HttpClientHandler.createDynamicObject(retHandle);
}
/**
* Property denoting whether the usage tracking is enabled or disabled (Getter)
*
* The SDK is allowed to track usage when this property is set to {@code true}.
* The collected data includes only non-sensitive information, such as the features used,
* the document type, the number of pages, etc.
*
* The default is {@code true}, i.e. usage tracking is enabled.
*
*/
public static boolean getUsageTracking()
{
boolean retVal = getUsageTrackingNative();
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Property denoting whether the usage tracking is enabled or disabled (Setter)
*
* The SDK is allowed to track usage when this property is set to {@code true}.
* The collected data includes only non-sensitive information, such as the features used,
* the document type, the number of pages, etc.
*
* The default is {@code true}, i.e. usage tracking is enabled.
*
*/
public static void setUsageTracking(boolean value)
{
boolean retVal = setUsageTrackingNative(value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Licensing service to use for all licensing requests (Getter)
*
* This property is relevant only for page-based licenses and is used to set the Licensing Gateway Service.
*
* The default is {@code "https://licensing.pdf-tools.com/api/v1/licenses/"} for the online Pdftools Licensing Service.
* If you plan to use the Licensing Gateway Service instead of the Pdftools Licensing Service, the property’s value must be a URI with the following elements:
*
* {@code http[s]://[‹user›[:‹password›]@]‹host›[:‹port›]}
*
* Where:
*
* -
* {@code http/https}: Protocol for connection to the Licensing Gateway Service.
*
* -
* {@code ‹user›:‹password›} (optional): Credentials for connection to the Licensing Gateway Service (basic authorization).
*
* -
* {@code ‹host›}: Hostname of the Licensing Gateway Service.
*
* -
* {@code ‹port›}: Port for connection to the Licensing Gateway Service.
*
*
*
* Example: {@code "http://localhost:9999"}
*/
public static java.net.URI getLicensingService()
{
String retVal = getLicensingServiceNative();
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return java.net.URI.create(retVal);
}
/**
* Licensing service to use for all licensing requests (Setter)
*
* This property is relevant only for page-based licenses and is used to set the Licensing Gateway Service.
*
* The default is {@code "https://licensing.pdf-tools.com/api/v1/licenses/"} for the online Pdftools Licensing Service.
* If you plan to use the Licensing Gateway Service instead of the Pdftools Licensing Service, the property’s value must be a URI with the following elements:
*
* {@code http[s]://[‹user›[:‹password›]@]‹host›[:‹port›]}
*
* Where:
*
* -
* {@code http/https}: Protocol for connection to the Licensing Gateway Service.
*
* -
* {@code ‹user›:‹password›} (optional): Credentials for connection to the Licensing Gateway Service (basic authorization).
*
* -
* {@code ‹host›}: Hostname of the Licensing Gateway Service.
*
* -
* {@code ‹port›}: Port for connection to the Licensing Gateway Service.
*
*
*
* Example: {@code "http://localhost:9999"}
*
* @throws IllegalArgumentException The URI is invalid.
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public static void setLicensingService(java.net.URI value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setLicensingServiceNative(value.toString());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
*
* A new snapshot is created whenever this property is accessed.
*
* Note: {@link Sdk#initialize } />must be called before accessing license information.
* Otherwise, the license is considered invalid.
*
*/
public static com.pdftools.LicenseInfo getLicenseInfoSnapshot()
{
long retHandle = getLicenseInfoSnapshotNative();
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.LicenseInfo.createDynamicObject(retHandle);
}
private static native boolean initializeNative(String license, String producerSuffix);
private static native boolean addFontDirectoryNative(String directory);
private static native String getVersionNative();
private static native boolean setProducerSuffixNative(String value);
private static native String getProducerFullNameNative();
private static native String getProxyNative();
private static native boolean setProxyNative(String value);
private static native long getHttpClientHandlerNative();
private static native boolean getUsageTrackingNative();
private static native boolean setUsageTrackingNative(boolean value);
private static native String getLicensingServiceNative();
private static native boolean setLicensingServiceNative(String value);
private static native long getLicenseInfoSnapshotNative();
}