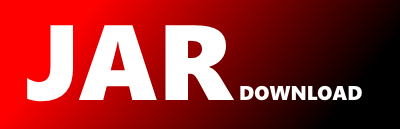
com.pdftools.crypto.ValidationInformation Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: ValidationInformation.java
*
* Description: ValidationInformation Enumeration
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.crypto;
/**
* Whether to embed validation information to enable the long-term validation (LTV) of the signature
*
* Embed revocation information such as online certificate status response (OCSP - RFC2560) and certificate revocation lists (CRL - RFC3280).
* Revocation information of a certificate is provided by a validation service at the time of signing and acts as proof that the certificate
* was valid at the time of signing.
* This is useful because even when the certificate expires or is revoked at a later time, the signature in the signed document remains valid.
*
* Embedding revocation information is optional but suggested when applying advanced or qualified electronic signatures.
* This feature is not always available.
* It has to be supported by the signing certificate and the cryptographic provider.
* Also, it is not supported by document time-stamp signatures.
* For these cases, a subsequent invocation of {@link com.pdftools.sign.Signer#process pdftools.sign.Signer.process} with
* {@link com.pdftools.sign.OutputOptions#getAddValidationInformation pdftools.sign.OutputOptions.getAddValidationInformation}
* is required.
*
* Revocation information is embedded for the signing certificate and all certificates of its trust chain.
* This implies that both OCSP responses and CRLs can be present in the same message.
* The disadvantages of embedding revocation information are the increase of the file size (normally by around 20KB),
* and that it requires a web request to a validation service, which delays the process of signing.
* Embedding revocation information requires an online connection to the CA that issues them.
* The firewall must be configured accordingly.
* If a web proxy is used (see {@link com.pdftools.Sdk#getProxy pdftools.Sdk.getProxy}), make sure the following MIME types are supported:
*
* -
* {@code application/ocsp-request}
* -
* {@code application/ocsp-response}
*
*/
public enum ValidationInformation
{
/**
* Basic: Do not add any validation information
*/
NONE(0),
/**
* LTV: Embed validation information into the signature
* This is only possible for Legacy PAdES Basic signatures (signature format {@link SignatureFormat#ADBE_PKCS7_DETACHED }).
*/
EMBED_IN_SIGNATURE(1),
/**
* LTV: Embed validation information into the document
*
* Embedding validation information into the document security store (DSS) is recommended,
* because it creates smaller files and is supported for all signature formats.
*
* The document security store has been standardized in 2009 by the standard for PAdES-LTV Profiles (ETSI TS 102 778-4).
* Therefore, some legacy signature validation software may not support this.
* For these cases, it is necessary to use {@code EmbedInSignature}.
*
*/
EMBED_IN_DOCUMENT(2);
ValidationInformation(int value)
{
this.value = value;
}
/**
* @hidden
*/
public static ValidationInformation fromValue(int value)
{
switch (value)
{
case 0: return NONE;
case 1: return EMBED_IN_SIGNATURE;
case 2: return EMBED_IN_DOCUMENT;
}
throw new IllegalArgumentException("Unknown value for ValidationInformation: " + value);
}
/**
* @hidden
*/
public int getValue()
{
return value;
}
private int value;
}