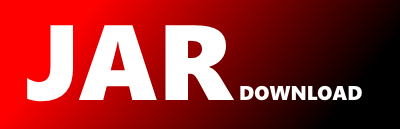
com.pdftools.crypto.providers.globalsigndss.Session Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: Session.java
*
* Description: PDFTOOLS Session Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.crypto.providers.globalsigndss;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* GlobalSign Digital Signing Service
*
* In this session, signatures can be created using different identities, i.e. signing certificates.
* Signing sessions and signing certificates expire after 10 minutes.
* After this time, they are renewed automatically.
*
* When signing with this provider, these errors can occur:
*
* -
* {@link com.pdftools.PermissionException }: If the account's quota is reached.
*
* -
*
* {@link com.pdftools.RetryException }: If one of the account's rate limits is exceeded.
*
* The service enforces rate limits for both creating new identities and signing operations.
* So, if multiple documents must be signed at once, it is advisable to re-use the signature configuration
* (and hence its signing certificates) for signing.
*
* -
* {@link com.pdftools.HttpException }: If a network error occurs or the service is not operational.
*
*
*/
public class Session extends com.pdftools.crypto.providers.Provider
{
protected Session(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Session createDynamicObject(long handle)
{
return new Session(handle);
}
/**
* Establish a session to the service
* @param url
*
* The URL to the service endpoint.
*
* Typically: {@code https://emea.api.dss.globalsign.com:8443}
* @param api_key
* Your account credentials’ key parameter for the login request.
* @param api_secret
* Your account credentials’ secret parameter for the login request.
* @param httpClientHandler
* The SSL configuration with the client certificate and trust store.
* Use {@link com.pdftools.HttpClientHandler#setClientCertificateAndKey pdftools.HttpClientHandler.setClientCertificateAndKey} to set your SSL client certificate "clientcert.crt"
* and private key "privateKey.key" of your GlobalSign account.
*
* @throws com.pdftools.HttpException If a network error occurs.
* @throws com.pdftools.PermissionException If a login error occurs, e.g. because the client certificate is rejected or the credentials are incorrect.
* @throws com.pdftools.RetryException If the login rate limit is exceeded.
* @throws IllegalArgumentException if {@code url} is {@code null}
* @throws IllegalArgumentException if {@code api_key} is {@code null}
* @throws IllegalArgumentException if {@code api_secret} is {@code null}
* @throws IllegalArgumentException if {@code httpClientHandler} is {@code null}
*/
public Session(java.net.URI url, String api_key, String api_secret, com.pdftools.HttpClientHandler httpClientHandler)
throws
com.pdftools.PermissionException,
com.pdftools.HttpException,
com.pdftools.RetryException
{
this(newHelper(url, api_key, api_secret, httpClientHandler));
}
private static long newHelper(java.net.URI url, String api_key, String api_secret, com.pdftools.HttpClientHandler httpClientHandler)
throws
com.pdftools.PermissionException,
com.pdftools.HttpException,
com.pdftools.RetryException
{
if (url == null)
throw new IllegalArgumentException("Argument 'url' must not be null.", new NullPointerException("'url'"));
if (api_key == null)
throw new IllegalArgumentException("Argument 'api_key' must not be null.", new NullPointerException("'api_key'"));
if (api_secret == null)
throw new IllegalArgumentException("Argument 'api_secret' must not be null.", new NullPointerException("'api_secret'"));
if (httpClientHandler == null)
throw new IllegalArgumentException("Argument 'httpClientHandler' must not be null.", new NullPointerException("'httpClientHandler'"));
long handle = newNative(url.toString(), api_key, api_secret, getHandle(httpClientHandler), httpClientHandler);
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 23: throw new com.pdftools.PermissionException(getLastErrorMessage());
case 24: throw new com.pdftools.HttpException(getLastErrorMessage());
case 25: throw new com.pdftools.RetryException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* Create a signing certificate for an account with a static identity
* The returned signature configuration can be used for multiple signature operations.
* @return
*
* @throws com.pdftools.HttpException If a network error occurs.
* @throws com.pdftools.PermissionException If the request is not authorized by the service.
* @throws com.pdftools.RetryException If the rate limit for creating new identities has been exceeded.
*/
public com.pdftools.crypto.providers.globalsigndss.SignatureConfiguration createSignatureForStaticIdentity()
throws
com.pdftools.PermissionException,
com.pdftools.HttpException,
com.pdftools.RetryException
{
long retHandle = createSignatureForStaticIdentityNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 23: throw new com.pdftools.PermissionException(getLastErrorMessage());
case 24: throw new com.pdftools.HttpException(getLastErrorMessage());
case 25: throw new com.pdftools.RetryException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return com.pdftools.crypto.providers.globalsigndss.SignatureConfiguration.createDynamicObject(retHandle);
}
/**
* Create a signing certificate for an account with a dynamic identity.
* @param identity
*
* The dynamic identity as JSON string.
*
* Example:
* {@code { "subject_dn": {"common_name": "John Doe" } }}
* @return
*
* @throws com.pdftools.HttpException If a network error occurs.
* @throws com.pdftools.PermissionException If the request is not authorized by the service.
* @throws com.pdftools.RetryException If the rate limit for creating new identities has been exceeded.
* @throws IllegalArgumentException if {@code identity} is {@code null}
*/
public com.pdftools.crypto.providers.globalsigndss.SignatureConfiguration createSignatureForDynamicIdentity(String identity)
throws
com.pdftools.PermissionException,
com.pdftools.HttpException,
com.pdftools.RetryException
{
if (identity == null)
throw new IllegalArgumentException("Argument 'identity' must not be null.", new NullPointerException("'identity'"));
long retHandle = createSignatureForDynamicIdentityNative(getHandle(), identity);
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 23: throw new com.pdftools.PermissionException(getLastErrorMessage());
case 24: throw new com.pdftools.HttpException(getLastErrorMessage());
case 25: throw new com.pdftools.RetryException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return com.pdftools.crypto.providers.globalsigndss.SignatureConfiguration.createDynamicObject(retHandle);
}
/**
* Create a time-stamp configuration
* @return
*/
public com.pdftools.crypto.providers.globalsigndss.TimestampConfiguration createTimestamp()
{
long retHandle = createTimestampNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.crypto.providers.globalsigndss.TimestampConfiguration.createDynamicObject(retHandle);
}
private static native long newNative(String url, String api_key, String api_secret, long httpClientHandler, com.pdftools.HttpClientHandler httpClientHandlerObj);
private native long createSignatureForStaticIdentityNative(long handle);
private native long createSignatureForDynamicIdentityNative(long handle, String identity);
private native long createTimestampNative(long handle);
}