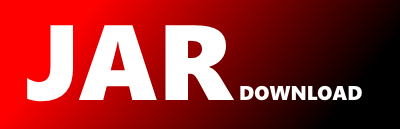
com.pdftools.crypto.providers.swisscomsigsrv.Session Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: Session.java
*
* Description: PDFTOOLS Session Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.crypto.providers.swisscomsigsrv;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The Swisscom Signing Service
*
*
* When signing with this provider, these errors can occur:
*
* -
* {@link com.pdftools.PermissionException }: The server did not accept the SSL client certificate or the Claimed Identity string.
*
* -
* {@link com.pdftools.PermissionException }: The requested requested distinguished name of the on-demand certificate is not allowed ({@link Session#createSignatureForOnDemandIdentity }).
*
* -
* {@link com.pdftools.RetryException }: The signing request could not be processed on time by the service.
* The service may be overloaded.
*
* -
* {@link IllegalArgumentException }: The key identity of the Claimed Identity string is invalid or not allowed.
*
* -
* {@link com.pdftools.HttpException }: If a network error occurs or the service is not operational.
*
*
*
* When signing with step-up authorization, these errors can also occur.
*
* -
* {@link com.pdftools.PermissionException }: The user canceled the authorization request or failed to enter correct authentication data (password, OTP).
*
*
*/
public class Session extends com.pdftools.crypto.providers.Provider
{
protected Session(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Session createDynamicObject(long handle)
{
return new Session(handle);
}
/**
*
* @param url
*
* The service endpoint base URL.
*
* Example: {@code https://ais.swisscom.com}
* @param httpClientHandler
* The SSL configuration with the client certificate and trust store.
* Use {@link com.pdftools.HttpClientHandler#setClientCertificate pdftools.HttpClientHandler.setClientCertificate} to set your SSL client certificate "clientcert.p12"
* of your Swisscom Signing Service account.
*
* @throws com.pdftools.HttpException If a network error occurs.
* @throws com.pdftools.PermissionException If the SSL client certificate is rejected.
* @throws IllegalArgumentException if {@code url} is {@code null}
* @throws IllegalArgumentException if {@code httpClientHandler} is {@code null}
*/
public Session(java.net.URI url, com.pdftools.HttpClientHandler httpClientHandler)
throws
com.pdftools.PermissionException,
com.pdftools.HttpException
{
this(newHelper(url, httpClientHandler));
}
private static long newHelper(java.net.URI url, com.pdftools.HttpClientHandler httpClientHandler)
throws
com.pdftools.PermissionException,
com.pdftools.HttpException
{
if (url == null)
throw new IllegalArgumentException("Argument 'url' must not be null.", new NullPointerException("'url'"));
if (httpClientHandler == null)
throw new IllegalArgumentException("Argument 'httpClientHandler' must not be null.", new NullPointerException("'httpClientHandler'"));
long handle = newNative(url.toString(), getHandle(httpClientHandler), httpClientHandler);
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 23: throw new com.pdftools.PermissionException(getLastErrorMessage());
case 24: throw new com.pdftools.HttpException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* Create a signature configuration for a static certificate.
* @param identity
*
* The Claimed Identity string as provided by Swisscom: {@code ‹customer name›:‹key identity›}
*
* Example: {@code "ais-90days-trial:static-saphir4-ch"}
* @param name
*
* Name of the signer.
* This parameter is not used for certificate selection, but for the signature appearance and signature description in the PDF only.
*
* Example: {@code "Signing Service TEST account"}
* @return
* @throws IllegalArgumentException if {@code identity} is {@code null}
* @throws IllegalArgumentException if {@code name} is {@code null}
*/
public com.pdftools.crypto.providers.swisscomsigsrv.SignatureConfiguration createSignatureForStaticIdentity(String identity, String name)
{
if (identity == null)
throw new IllegalArgumentException("Argument 'identity' must not be null.", new NullPointerException("'identity'"));
if (name == null)
throw new IllegalArgumentException("Argument 'name' must not be null.", new NullPointerException("'name'"));
long retHandle = createSignatureForStaticIdentityNative(getHandle(), identity, name);
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.crypto.providers.swisscomsigsrv.SignatureConfiguration.createDynamicObject(retHandle);
}
/**
* Create a signature configuration for an on-demand certificate
* @param identity
*
* The Claimed Identity string as provided by Swisscom: {@code ‹customer name›:‹key identity›}
*
* Example: {@code "ais-90days-trial:OnDemand-Advanced4"}
* @param distinguishedName
*
* The requested distinguished name of the on-demand certificate.
*
* Example: {@code "cn=Hans Muster,o=ACME,c=CH"}
* @param stepUp
* Options for step-up authorization using Mobile ID.
* @return
* @throws IllegalArgumentException if {@code identity} is {@code null}
* @throws IllegalArgumentException if {@code distinguishedName} is {@code null}
*/
public com.pdftools.crypto.providers.swisscomsigsrv.SignatureConfiguration createSignatureForOnDemandIdentity(String identity, String distinguishedName, com.pdftools.crypto.providers.swisscomsigsrv.StepUp stepUp)
{
if (identity == null)
throw new IllegalArgumentException("Argument 'identity' must not be null.", new NullPointerException("'identity'"));
if (distinguishedName == null)
throw new IllegalArgumentException("Argument 'distinguishedName' must not be null.", new NullPointerException("'distinguishedName'"));
long retHandle = createSignatureForOnDemandIdentityNative(getHandle(), identity, distinguishedName, getHandle(stepUp), stepUp);
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.crypto.providers.swisscomsigsrv.SignatureConfiguration.createDynamicObject(retHandle);
}
/**
* Create a time-stamp configuration
* @param identity
*
* The Claimed Identity string as provided by Swisscom: {@code ‹customer name›}
*
* Example: {@code "ais-90days-trial"}
* @return
* @throws IllegalArgumentException if {@code identity} is {@code null}
*/
public com.pdftools.crypto.providers.swisscomsigsrv.TimestampConfiguration createTimestamp(String identity)
{
if (identity == null)
throw new IllegalArgumentException("Argument 'identity' must not be null.", new NullPointerException("'identity'"));
long retHandle = createTimestampNative(getHandle(), identity);
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.crypto.providers.swisscomsigsrv.TimestampConfiguration.createDynamicObject(retHandle);
}
private static native long newNative(String url, long httpClientHandler, com.pdftools.HttpClientHandler httpClientHandlerObj);
private native long createSignatureForStaticIdentityNative(long handle, String identity, String name);
private native long createSignatureForOnDemandIdentityNative(long handle, String identity, String distinguishedName, long stepUp, com.pdftools.crypto.providers.swisscomsigsrv.StepUp stepUpObj);
private native long createTimestampNative(long handle, String identity);
}