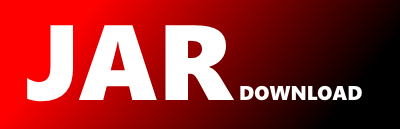
com.pdftools.documentassembly.DocumentAssembler Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: DocumentAssembler.java
*
* Description: PDFTOOLS DocumentAssembler Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.documentassembly;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The class for splitting or merging PDF documents
*/
public class DocumentAssembler extends NativeObject implements AutoCloseable
{
protected DocumentAssembler(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static DocumentAssembler createDynamicObject(long handle)
{
return new DocumentAssembler(handle);
}
/**
*
* @param outStream
* The stream to which the output PDF is written
*
* @throws com.pdftools.LicenseException The license check has failed.
* @throws IllegalArgumentException An invalid encryption was specified in {@link com.pdftools.documentassembly.DocumentAssembler# outOptions}.
* @throws java.io.IOException Unable to write to the stream.
* @throws com.pdftools.GenericException A generic error occurred.
* @throws IllegalArgumentException if {@code outStream} is {@code null}
*/
public DocumentAssembler(com.pdftools.sys.Stream outStream)
throws
java.io.IOException,
com.pdftools.GenericException,
com.pdftools.LicenseException
{
this(outStream, null, null);
}
/**
*
* @param outStream
* The stream to which the output PDF is written
* @param outOptions
* The PDF output options, e.g. to encrypt the output document.
*
* @throws com.pdftools.LicenseException The license check has failed.
* @throws IllegalArgumentException An invalid encryption was specified in {@link com.pdftools.documentassembly.DocumentAssembler# outOptions}.
* @throws java.io.IOException Unable to write to the stream.
* @throws com.pdftools.GenericException A generic error occurred.
* @throws IllegalArgumentException if {@code outStream} is {@code null}
*/
public DocumentAssembler(com.pdftools.sys.Stream outStream, com.pdftools.pdf.OutputOptions outOptions)
throws
java.io.IOException,
com.pdftools.GenericException,
com.pdftools.LicenseException
{
this(outStream, outOptions, null);
}
/**
*
* @param outStream
* The stream to which the output PDF is written
* @param outOptions
* The PDF output options, e.g. to encrypt the output document.
* @param conformance
*
* The required conformance level of the PDF document.
* Adding pages or content from incompatible documents or using
* incompatible features will lead to a conformance error.
*
* When using {@code null}, the conformance is determined automatically,
* based on the conformance of the input documents used in the {@link DocumentAssembler#append } method
* and the requirements of the used features.
*
*
* @throws com.pdftools.LicenseException The license check has failed.
* @throws IllegalArgumentException An invalid encryption was specified in {@link com.pdftools.documentassembly.DocumentAssembler# outOptions}.
* @throws java.io.IOException Unable to write to the stream.
* @throws com.pdftools.GenericException A generic error occurred.
* @throws IllegalArgumentException if {@code outStream} is {@code null}
*/
public DocumentAssembler(com.pdftools.sys.Stream outStream, com.pdftools.pdf.OutputOptions outOptions, com.pdftools.pdf.Conformance conformance)
throws
java.io.IOException,
com.pdftools.GenericException,
com.pdftools.LicenseException
{
this(newHelper(outStream, outOptions, conformance));
}
private static long newHelper(com.pdftools.sys.Stream outStream, com.pdftools.pdf.OutputOptions outOptions, com.pdftools.pdf.Conformance conformance)
throws
java.io.IOException,
com.pdftools.GenericException,
com.pdftools.LicenseException
{
if (outStream == null)
throw new IllegalArgumentException("Argument 'outStream' must not be null.", new NullPointerException("'outStream'"));
long handle = newNative(outStream, getHandle(outOptions), outOptions, conformance == null ? 0 : conformance.getValue(), conformance == null);
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
case 4: throw new java.io.IOException(getLastErrorMessage());
case 10: throw new com.pdftools.GenericException(getLastErrorMessage());
case 12: throw new com.pdftools.LicenseException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return handle;
}
/**
*
* This method copies document properties and a range of pages from {@link com.pdftools.documentassembly.DocumentAssembler#append inDoc}.
* @param inDoc
*
* @throws com.pdftools.LicenseException The license check has failed.
* @throws IllegalArgumentException The {@link com.pdftools.documentassembly.DocumentAssembler#append firstPage} or {@link com.pdftools.documentassembly.DocumentAssembler#append lastPage} are not in the allowed range.
* @throws IllegalArgumentException If the method has already been called with any of the following properties set to {@code true}:
*
* -
* {@link DocumentCopyOptions#getCopyMetadata }
* -
* {@link DocumentCopyOptions#getCopyViewerSettings }
* -
* {@link DocumentCopyOptions#getCopyOutputIntent }
*
* @throws com.pdftools.ConformanceException The conformance level of the input document is not compatible
* with the conformance level of the output document.
* @throws com.pdftools.ConformanceException The explicitly requested conformance level is PDF/A Level A
* ({@link com.pdftools.pdf.Conformance#PDF_A1_A pdftools.pdf.Conformance.PDF_A1_A}, {@link com.pdftools.pdf.Conformance#PDF_A2_A pdftools.pdf.Conformance.PDF_A2_A},
* or {@link com.pdftools.pdf.Conformance#PDF_A3_A pdftools.pdf.Conformance.PDF_A3_A})
* and the copy option {@link PageCopyOptions#getCopyLogicalStructure } is set to {@code false}.
* @throws IllegalStateException If {@link DocumentAssembler#assemble } has already been called.
* @throws com.pdftools.ProcessingException The processing has failed.
* @throws IllegalArgumentException if {@code inDoc} is {@code null}
*/
public void append(com.pdftools.pdf.Document inDoc)
throws
com.pdftools.LicenseException,
com.pdftools.ConformanceException,
com.pdftools.ProcessingException
{
if (inDoc == null)
throw new IllegalArgumentException("Argument 'inDoc' must not be null.", new NullPointerException("'inDoc'"));
append(inDoc, null, null, null, null);
}
/**
*
* This method copies document properties and a range of pages from {@link com.pdftools.documentassembly.DocumentAssembler#append inDoc}.
* @param inDoc
* @param firstPage
*
* Optional parameter denoting the index of the first page to be copied. This index is one-based.
* If set, the number must be in the range of {@code 1} (first page) to {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount} (last page).
*
* If not set, {@code 1} is used.
*
*
* @throws com.pdftools.LicenseException The license check has failed.
* @throws IllegalArgumentException The {@link com.pdftools.documentassembly.DocumentAssembler#append firstPage} or {@link com.pdftools.documentassembly.DocumentAssembler#append lastPage} are not in the allowed range.
* @throws IllegalArgumentException If the method has already been called with any of the following properties set to {@code true}:
*
* -
* {@link DocumentCopyOptions#getCopyMetadata }
* -
* {@link DocumentCopyOptions#getCopyViewerSettings }
* -
* {@link DocumentCopyOptions#getCopyOutputIntent }
*
* @throws com.pdftools.ConformanceException The conformance level of the input document is not compatible
* with the conformance level of the output document.
* @throws com.pdftools.ConformanceException The explicitly requested conformance level is PDF/A Level A
* ({@link com.pdftools.pdf.Conformance#PDF_A1_A pdftools.pdf.Conformance.PDF_A1_A}, {@link com.pdftools.pdf.Conformance#PDF_A2_A pdftools.pdf.Conformance.PDF_A2_A},
* or {@link com.pdftools.pdf.Conformance#PDF_A3_A pdftools.pdf.Conformance.PDF_A3_A})
* and the copy option {@link PageCopyOptions#getCopyLogicalStructure } is set to {@code false}.
* @throws IllegalStateException If {@link DocumentAssembler#assemble } has already been called.
* @throws com.pdftools.ProcessingException The processing has failed.
* @throws IllegalArgumentException if {@code inDoc} is {@code null}
*/
public void append(com.pdftools.pdf.Document inDoc, Integer firstPage)
throws
com.pdftools.LicenseException,
com.pdftools.ConformanceException,
com.pdftools.ProcessingException
{
if (inDoc == null)
throw new IllegalArgumentException("Argument 'inDoc' must not be null.", new NullPointerException("'inDoc'"));
append(inDoc, firstPage, null, null, null);
}
/**
*
* This method copies document properties and a range of pages from {@link com.pdftools.documentassembly.DocumentAssembler#append inDoc}.
* @param inDoc
* @param firstPage
*
* Optional parameter denoting the index of the first page to be copied. This index is one-based.
* If set, the number must be in the range of {@code 1} (first page) to {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount} (last page).
*
* If not set, {@code 1} is used.
*
* @param lastPage
*
* Optional parameter denoting the index of the last page to be copied. This index is one-based.
* If set, the number must be in the range of {@code 1} (first page) to {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount} (last page).
*
* If not set, {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount} is used.
*
*
* @throws com.pdftools.LicenseException The license check has failed.
* @throws IllegalArgumentException The {@link com.pdftools.documentassembly.DocumentAssembler#append firstPage} or {@link com.pdftools.documentassembly.DocumentAssembler#append lastPage} are not in the allowed range.
* @throws IllegalArgumentException If the method has already been called with any of the following properties set to {@code true}:
*
* -
* {@link DocumentCopyOptions#getCopyMetadata }
* -
* {@link DocumentCopyOptions#getCopyViewerSettings }
* -
* {@link DocumentCopyOptions#getCopyOutputIntent }
*
* @throws com.pdftools.ConformanceException The conformance level of the input document is not compatible
* with the conformance level of the output document.
* @throws com.pdftools.ConformanceException The explicitly requested conformance level is PDF/A Level A
* ({@link com.pdftools.pdf.Conformance#PDF_A1_A pdftools.pdf.Conformance.PDF_A1_A}, {@link com.pdftools.pdf.Conformance#PDF_A2_A pdftools.pdf.Conformance.PDF_A2_A},
* or {@link com.pdftools.pdf.Conformance#PDF_A3_A pdftools.pdf.Conformance.PDF_A3_A})
* and the copy option {@link PageCopyOptions#getCopyLogicalStructure } is set to {@code false}.
* @throws IllegalStateException If {@link DocumentAssembler#assemble } has already been called.
* @throws com.pdftools.ProcessingException The processing has failed.
* @throws IllegalArgumentException if {@code inDoc} is {@code null}
*/
public void append(com.pdftools.pdf.Document inDoc, Integer firstPage, Integer lastPage)
throws
com.pdftools.LicenseException,
com.pdftools.ConformanceException,
com.pdftools.ProcessingException
{
if (inDoc == null)
throw new IllegalArgumentException("Argument 'inDoc' must not be null.", new NullPointerException("'inDoc'"));
append(inDoc, firstPage, lastPage, null, null);
}
/**
*
* This method copies document properties and a range of pages from {@link com.pdftools.documentassembly.DocumentAssembler#append inDoc}.
* @param inDoc
* @param firstPage
*
* Optional parameter denoting the index of the first page to be copied. This index is one-based.
* If set, the number must be in the range of {@code 1} (first page) to {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount} (last page).
*
* If not set, {@code 1} is used.
*
* @param lastPage
*
* Optional parameter denoting the index of the last page to be copied. This index is one-based.
* If set, the number must be in the range of {@code 1} (first page) to {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount} (last page).
*
* If not set, {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount} is used.
*
* @param documentCopyOptions
*
* @throws com.pdftools.LicenseException The license check has failed.
* @throws IllegalArgumentException The {@link com.pdftools.documentassembly.DocumentAssembler#append firstPage} or {@link com.pdftools.documentassembly.DocumentAssembler#append lastPage} are not in the allowed range.
* @throws IllegalArgumentException If the method has already been called with any of the following properties set to {@code true}:
*
* -
* {@link DocumentCopyOptions#getCopyMetadata }
* -
* {@link DocumentCopyOptions#getCopyViewerSettings }
* -
* {@link DocumentCopyOptions#getCopyOutputIntent }
*
* @throws com.pdftools.ConformanceException The conformance level of the input document is not compatible
* with the conformance level of the output document.
* @throws com.pdftools.ConformanceException The explicitly requested conformance level is PDF/A Level A
* ({@link com.pdftools.pdf.Conformance#PDF_A1_A pdftools.pdf.Conformance.PDF_A1_A}, {@link com.pdftools.pdf.Conformance#PDF_A2_A pdftools.pdf.Conformance.PDF_A2_A},
* or {@link com.pdftools.pdf.Conformance#PDF_A3_A pdftools.pdf.Conformance.PDF_A3_A})
* and the copy option {@link PageCopyOptions#getCopyLogicalStructure } is set to {@code false}.
* @throws IllegalStateException If {@link DocumentAssembler#assemble } has already been called.
* @throws com.pdftools.ProcessingException The processing has failed.
* @throws IllegalArgumentException if {@code inDoc} is {@code null}
*/
public void append(com.pdftools.pdf.Document inDoc, Integer firstPage, Integer lastPage, com.pdftools.documentassembly.DocumentCopyOptions documentCopyOptions)
throws
com.pdftools.LicenseException,
com.pdftools.ConformanceException,
com.pdftools.ProcessingException
{
if (inDoc == null)
throw new IllegalArgumentException("Argument 'inDoc' must not be null.", new NullPointerException("'inDoc'"));
append(inDoc, firstPage, lastPage, documentCopyOptions, null);
}
/**
*
* This method copies document properties and a range of pages from {@link com.pdftools.documentassembly.DocumentAssembler#append inDoc}.
* @param inDoc
* @param firstPage
*
* Optional parameter denoting the index of the first page to be copied. This index is one-based.
* If set, the number must be in the range of {@code 1} (first page) to {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount} (last page).
*
* If not set, {@code 1} is used.
*
* @param lastPage
*
* Optional parameter denoting the index of the last page to be copied. This index is one-based.
* If set, the number must be in the range of {@code 1} (first page) to {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount} (last page).
*
* If not set, {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount} is used.
*
* @param documentCopyOptions
* @param pageCopyOptions
*
* @throws com.pdftools.LicenseException The license check has failed.
* @throws IllegalArgumentException The {@link com.pdftools.documentassembly.DocumentAssembler#append firstPage} or {@link com.pdftools.documentassembly.DocumentAssembler#append lastPage} are not in the allowed range.
* @throws IllegalArgumentException If the method has already been called with any of the following properties set to {@code true}:
*
* -
* {@link DocumentCopyOptions#getCopyMetadata }
* -
* {@link DocumentCopyOptions#getCopyViewerSettings }
* -
* {@link DocumentCopyOptions#getCopyOutputIntent }
*
* @throws com.pdftools.ConformanceException The conformance level of the input document is not compatible
* with the conformance level of the output document.
* @throws com.pdftools.ConformanceException The explicitly requested conformance level is PDF/A Level A
* ({@link com.pdftools.pdf.Conformance#PDF_A1_A pdftools.pdf.Conformance.PDF_A1_A}, {@link com.pdftools.pdf.Conformance#PDF_A2_A pdftools.pdf.Conformance.PDF_A2_A},
* or {@link com.pdftools.pdf.Conformance#PDF_A3_A pdftools.pdf.Conformance.PDF_A3_A})
* and the copy option {@link PageCopyOptions#getCopyLogicalStructure } is set to {@code false}.
* @throws IllegalStateException If {@link DocumentAssembler#assemble } has already been called.
* @throws com.pdftools.ProcessingException The processing has failed.
* @throws IllegalArgumentException if {@code inDoc} is {@code null}
*/
public void append(com.pdftools.pdf.Document inDoc, Integer firstPage, Integer lastPage, com.pdftools.documentassembly.DocumentCopyOptions documentCopyOptions, com.pdftools.documentassembly.PageCopyOptions pageCopyOptions)
throws
com.pdftools.LicenseException,
com.pdftools.ConformanceException,
com.pdftools.ProcessingException
{
if (inDoc == null)
throw new IllegalArgumentException("Argument 'inDoc' must not be null.", new NullPointerException("'inDoc'"));
boolean retVal = appendNative(getHandle(), getHandle(inDoc), inDoc, firstPage, lastPage, getHandle(documentCopyOptions), documentCopyOptions, getHandle(pageCopyOptions), pageCopyOptions);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
case 3: throw new IllegalArgumentException(getLastErrorMessage());
case 12: throw new com.pdftools.LicenseException(getLastErrorMessage());
case 18: throw new com.pdftools.ConformanceException(getLastErrorMessage());
case 21: throw new com.pdftools.ProcessingException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* Assemble the input documents
* The input documents appended with {@link DocumentAssembler#append } are assembled into the output PDF.
* @return
* The assembled PDF, which can be used as a new input for further processing.
*
* @throws IllegalStateException If {@link DocumentAssembler#assemble } has already been called.
*/
public com.pdftools.pdf.Document assemble()
{
long retHandle = assembleNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return com.pdftools.pdf.Document.createDynamicObject(retHandle);
}
private static native long newNative(com.pdftools.sys.Stream outStream, long outOptions, com.pdftools.pdf.OutputOptions outOptionsObj, int conformance, boolean isNullconformance);
private native boolean appendNative(long handle, long inDoc, com.pdftools.pdf.Document inDocObj, Integer firstPage, Integer lastPage, long documentCopyOptions, com.pdftools.documentassembly.DocumentCopyOptions documentCopyOptionsObj, long pageCopyOptions, com.pdftools.documentassembly.PageCopyOptions pageCopyOptionsObj);
private native long assembleNative(long handle);
/**
* Close the object.
* Release all resources associated with the object.
* @throws com.pdftools.PdfToolsException only explicitly stated in a superclass
*/
public void close()
throws com.pdftools.PdfToolsException,
java.io.IOException
{
try
{
if (!close(getHandle()))
throwLastError();
}
finally
{
setHandle(0);
}
}
private native boolean close(long hObject);
}