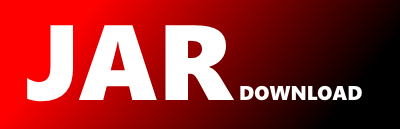
com.pdftools.documentassembly.PageCopyOptions Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: PageCopyOptions.java
*
* Description: PDFTOOLS PageCopyOptions Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.documentassembly;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
*
* This class determines whether and how different PDF elements are copied.
*/
public class PageCopyOptions extends NativeObject
{
protected PageCopyOptions(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static PageCopyOptions createDynamicObject(long handle)
{
return new PageCopyOptions(handle);
}
/**
*
*/
public PageCopyOptions()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* Copy strategy for links. (Getter)
*
* Specifies how links (document internal and external links) are treated
* when copying a page.
*
* Default value: {@link CopyStrategy#COPY }
*/
public com.pdftools.documentassembly.CopyStrategy getLinks()
{
int retVal = getLinksNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.documentassembly.CopyStrategy.fromValue(retVal);
}
/**
* Copy strategy for links. (Setter)
*
* Specifies how links (document internal and external links) are treated
* when copying a page.
*
* Default value: {@link CopyStrategy#COPY }
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setLinks(com.pdftools.documentassembly.CopyStrategy value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setLinksNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Copy strategy for form fields. (Getter)
*
* Specifies how form fields are treated when copying a page.
*
* Default value: {@link CopyStrategy#COPY }
*/
public com.pdftools.documentassembly.CopyStrategy getFormFields()
{
int retVal = getFormFieldsNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.documentassembly.CopyStrategy.fromValue(retVal);
}
/**
* Copy strategy for form fields. (Setter)
*
* Specifies how form fields are treated when copying a page.
*
* Default value: {@link CopyStrategy#COPY }
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setFormFields(com.pdftools.documentassembly.CopyStrategy value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setFormFieldsNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Removal strategy for signed signature fields. (Getter)
*
* Signed digital signatures are always invalidated when copying a page
* and therefore have to be removed.
* This property specifies, whether the visual representation of the signature
* is preserved.
*
* Default value: {@link RemovalStrategy#REMOVE }
*/
public com.pdftools.documentassembly.RemovalStrategy getSignedSignatures()
{
int retVal = getSignedSignaturesNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.documentassembly.RemovalStrategy.fromValue(retVal);
}
/**
* Removal strategy for signed signature fields. (Setter)
*
* Signed digital signatures are always invalidated when copying a page
* and therefore have to be removed.
* This property specifies, whether the visual representation of the signature
* is preserved.
*
* Default value: {@link RemovalStrategy#REMOVE }
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setSignedSignatures(com.pdftools.documentassembly.RemovalStrategy value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setSignedSignaturesNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Copy strategy for unsigned signature fields. (Getter)
*
* Specifies how signature fields are treated, that are not yet signed.
*
* Default value: {@link CopyStrategy#COPY }
*/
public com.pdftools.documentassembly.CopyStrategy getUnsignedSignatures()
{
int retVal = getUnsignedSignaturesNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.documentassembly.CopyStrategy.fromValue(retVal);
}
/**
* Copy strategy for unsigned signature fields. (Setter)
*
* Specifies how signature fields are treated, that are not yet signed.
*
* Default value: {@link CopyStrategy#COPY }
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setUnsignedSignatures(com.pdftools.documentassembly.CopyStrategy value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setUnsignedSignaturesNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Copy strategy for annotations. (Getter)
*
* Specifies how interactive annotations (like sticky notes or text highlights)
* are treated when copying a page.
* This does not include links, form fields and signature fields which
* are not considered annotations in this product.
*
* Default value: {@link CopyStrategy#COPY }
*/
public com.pdftools.documentassembly.CopyStrategy getAnnotations()
{
int retVal = getAnnotationsNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.documentassembly.CopyStrategy.fromValue(retVal);
}
/**
* Copy strategy for annotations. (Setter)
*
* Specifies how interactive annotations (like sticky notes or text highlights)
* are treated when copying a page.
* This does not include links, form fields and signature fields which
* are not considered annotations in this product.
*
* Default value: {@link CopyStrategy#COPY }
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setAnnotations(com.pdftools.documentassembly.CopyStrategy value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setAnnotationsNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Copy outline items (bookmarks). (Getter)
*
* Specifies whether outline items (also known as bookmarks) pointing to the copied page
* should be copied to the target document automatically.
*
* Default value: {@code true}
*/
public boolean getCopyOutlineItems()
{
boolean retVal = getCopyOutlineItemsNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Copy outline items (bookmarks). (Setter)
*
* Specifies whether outline items (also known as bookmarks) pointing to the copied page
* should be copied to the target document automatically.
*
* Default value: {@code true}
*/
public void setCopyOutlineItems(boolean value)
{
boolean retVal = setCopyOutlineItemsNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Copy associated files. (Getter)
*
* Specifies whether embedded files associated with a page or any of its
* subobjects are also copied when copying the page.
*
* Default value: {@code true}
*/
public boolean getCopyAssociatedFiles()
{
boolean retVal = getCopyAssociatedFilesNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Copy associated files. (Setter)
*
* Specifies whether embedded files associated with a page or any of its
* subobjects are also copied when copying the page.
*
* Default value: {@code true}
*/
public void setCopyAssociatedFiles(boolean value)
{
boolean retVal = setCopyAssociatedFilesNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Copy the logical structure and tagging information. (Getter)
*
* Specifies whether the logical structure and tagging information associated
* with a page or its content is also copied when copying the page.
*
* This is required if the target document conformance is PDF/A Level a.
*
* Default value: {@code true}
*/
public boolean getCopyLogicalStructure()
{
boolean retVal = getCopyLogicalStructureNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Copy the logical structure and tagging information. (Setter)
*
* Specifies whether the logical structure and tagging information associated
* with a page or its content is also copied when copying the page.
*
* This is required if the target document conformance is PDF/A Level a.
*
* Default value: {@code true}
*/
public void setCopyLogicalStructure(boolean value)
{
boolean retVal = setCopyLogicalStructureNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Resolution of conflicting form field names. (Getter)
*
* Form field of different files can have the same name (identifier).
* This property specifies how name conflicts are resolved,
* when copying pages from multiple source files.
*
* Default value: {@link NameConflictResolution#MERGE }
*/
public com.pdftools.documentassembly.NameConflictResolution getFormFieldConflictResolution()
{
int retVal = getFormFieldConflictResolutionNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.documentassembly.NameConflictResolution.fromValue(retVal);
}
/**
* Resolution of conflicting form field names. (Setter)
*
* Form field of different files can have the same name (identifier).
* This property specifies how name conflicts are resolved,
* when copying pages from multiple source files.
*
* Default value: {@link NameConflictResolution#MERGE }
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setFormFieldConflictResolution(com.pdftools.documentassembly.NameConflictResolution value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setFormFieldConflictResolutionNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Copy strategy for named destinations (Getter)
*
* Specify whether named destinations are resolved when copying a page.
*
* Default value: {@link NamedDestinationCopyStrategy#COPY }
*/
public com.pdftools.documentassembly.NamedDestinationCopyStrategy getNamedDestinations()
{
int retVal = getNamedDestinationsNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.documentassembly.NamedDestinationCopyStrategy.fromValue(retVal);
}
/**
* Copy strategy for named destinations (Setter)
*
* Specify whether named destinations are resolved when copying a page.
*
* Default value: {@link NamedDestinationCopyStrategy#COPY }
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setNamedDestinations(com.pdftools.documentassembly.NamedDestinationCopyStrategy value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setNamedDestinationsNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Find and merge redundant resources. (Getter)
*
* Find and merge redundant resources such as fonts and images.
* This can lead to much smaller files, especially when copying
* pages from multiple similar source files.
* However, it also results in longer processing time.
*
* Default value: {@code true}
*/
public boolean getOptimizeResources()
{
boolean retVal = getOptimizeResourcesNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Find and merge redundant resources. (Setter)
*
* Find and merge redundant resources such as fonts and images.
* This can lead to much smaller files, especially when copying
* pages from multiple similar source files.
* However, it also results in longer processing time.
*
* Default value: {@code true}
*/
public void setOptimizeResources(boolean value)
{
boolean retVal = setOptimizeResourcesNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Specify how page(s) should be rotated. (Getter)
*
*
* Default: {@link PageRotation#NO_ROTATION }
*/
public com.pdftools.documentassembly.PageRotation getPageRotation()
{
int retVal = getPageRotationNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.documentassembly.PageRotation.fromValue(retVal);
}
/**
* Specify how page(s) should be rotated. (Setter)
*
*
* Default: {@link PageRotation#NO_ROTATION }
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setPageRotation(com.pdftools.documentassembly.PageRotation value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setPageRotationNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
private static native long newNative();
private native int getLinksNative(long handle);
private native boolean setLinksNative(long handle, int value);
private native int getFormFieldsNative(long handle);
private native boolean setFormFieldsNative(long handle, int value);
private native int getSignedSignaturesNative(long handle);
private native boolean setSignedSignaturesNative(long handle, int value);
private native int getUnsignedSignaturesNative(long handle);
private native boolean setUnsignedSignaturesNative(long handle, int value);
private native int getAnnotationsNative(long handle);
private native boolean setAnnotationsNative(long handle, int value);
private native boolean getCopyOutlineItemsNative(long handle);
private native boolean setCopyOutlineItemsNative(long handle, boolean value);
private native boolean getCopyAssociatedFilesNative(long handle);
private native boolean setCopyAssociatedFilesNative(long handle, boolean value);
private native boolean getCopyLogicalStructureNative(long handle);
private native boolean setCopyLogicalStructureNative(long handle, boolean value);
private native int getFormFieldConflictResolutionNative(long handle);
private native boolean setFormFieldConflictResolutionNative(long handle, int value);
private native int getNamedDestinationsNative(long handle);
private native boolean setNamedDestinationsNative(long handle, int value);
private native boolean getOptimizeResourcesNative(long handle);
private native boolean setOptimizeResourcesNative(long handle, boolean value);
private native int getPageRotationNative(long handle);
private native boolean setPageRotationNative(long handle, int value);
}