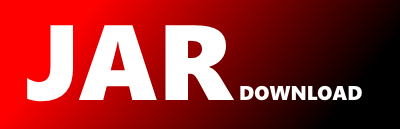
com.pdftools.geometry.units.Margin Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
The newest version!
/****************************************************************************
*
* File: Margin.java
*
* Description: Margin Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.geometry.units;
import java.util.Objects;
import com.pdftools.geometry.units.Length.Units;
/**
* The margin defines the space around elements.
*
The class is based on the respective distances left, bottom, right, and top of type {@link Length}.
*/
public class Margin
{
private double left;
private double bottom;
private double right;
private double top;
/**
* @hidden
*/
public Margin(double left, double bottom, double right, double top) {
this.left = left;
this.bottom = bottom;
this.right = right;
this.top = top;
}
/**
* @hidden
*/
public double getLeftValue() {
return this.left;
}
/**
* @hidden
*/
public double getBottomValue() {
return this.bottom;
}
/**
* @hidden
*/
public double getRightValue() {
return this.right;
}
/**
* @hidden
*/
public double getTopValue() {
return this.top;
}
/**
* Construct {@link Margin} object from individual values
* @param left as {@link Length} type
* @param bottom as {@link Length} type
* @param right as {@link Length} type
* @param top as {@link Length} type
*/
public Margin(Length left, Length bottom, Length right, Length top) {
this(left.getValue(), bottom.getValue(), right.getValue(), top.getValue());
}
/**
* Construct {@link Margin} object from values and a common unit.
* @param left as numerical value
* @param bottom as numerical value
* @param right as numerical value
* @param top as numerical value
* @param unit the unit
*/
public Margin(double left, double bottom, double right, double top, Units unit) {
this(new Length(left, unit), new Length(bottom, unit), new Length(right, unit), new Length(top, unit));
}
/**
* Construct a {@link Margin} object of equal margins
* @param margin as {@link Length} type
*/
public Margin(Length margin) {
this(margin.getValue(), margin.getValue(), margin.getValue(), margin.getValue());
}
/**
* Construct a {@link Margin} object of equal margins
* @param margin as numerical value
* @param unit the unit
*/
public Margin(double margin, Units unit) {
Length l = new Length(margin, unit);
this.left = l.getValue();
this.bottom = l.getValue();
this.right = l.getValue();
this.top = l.getValue();
}
/**
* Create a {@link Margin} object from a string representation.
* @param value Allowed are value-unit pairs of the form "<left_value><left_unit> <bottom_value><bottom_unit> <right_value><right_unit> <top_value><top_unit>". Example: "12.3cm 23.4mm 34.5mm 45.6mm"
* @return
*/
public static Margin parse(String value) {
Length[] lengths = Length.parseArray(value, 4);
return new Margin(lengths[0], lengths[1], lengths[2], lengths[3]);
}
/**
* Creates a string representation as left-bottom-right-top-tuple with associated suitable metric units, "m", "cm" or "mm".
*/
@Override
public String toString() {
return this.getLeft().toString() + " " + this.getBottom().toString() + " " + this.getRight().toString() + " " + this.getTop().toString();
}
/**
* Creates a string representation as left-bottom-right-top-tuple with the specified unit.
*/
public String toString(Units unit) {
return this.getLeft().toString(unit) + " " + this.getBottom().toString(unit) + " " + this.getRight().toString(unit) + " " + this.getTop().toString(unit);
}
/**
* Gets the left margin as {@link Length} object.
*/
public Length getLeft() {
return new Length(left, Units.POINT);
}
/**
* Gets the bottom margin as {@link Length} object.
*/
public Length getBottom() {
return new Length(bottom, Units.POINT);
}
/**
* Gets the right margin as {@link Length} object.
*/
public Length getRight() {
return new Length(right, Units.POINT);
}
/**
* Gets the top margin as {@link Length} object.
*/
public Length getTop() {
return new Length(top, Units.POINT);
}
@Override
public boolean equals(Object obj)
{
if (obj != null && obj instanceof Margin)
{
if (((Margin) obj).left != left)
return false;
if (((Margin) obj).bottom != bottom)
return false;
if (((Margin) obj).right != right)
return false;
if (((Margin) obj).top != top)
return false;
return true;
}
return false;
}
@Override
public int hashCode() {
return Objects.hash(left, bottom, right, top);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy