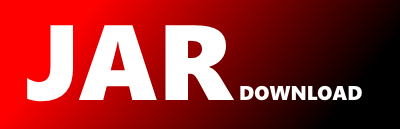
com.pdftools.geometry.units.Rectangle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
/****************************************************************************
*
* File: Rectangle.java
*
* Description: Rectangle Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.geometry.units;
import java.util.Objects;
import java.lang.Math;
import com.pdftools.geometry.units.Length.Units;
/**
* A class that is based on a position (origin) of type {@link Point} and a size of type {@link Size} that spans the rectangle.
*/
public class Rectangle
{
private double x;
private double y;
private double width;
private double height;
/**
* @hidden
*/
public Rectangle(double x, double y, double width, double height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
/**
* @hidden
*/
public double getXValue() {
return this.x;
}
/**
* @hidden
*/
public double getYValue() {
return this.y;
}
/**
* @hidden
*/
public double getWidthValue() {
return this.width;
}
/**
* @hidden
*/
public double getHeightValue() {
return this.height;
}
/**
* Construct {@link Rectangle} object from individual values
* @param x as {@link Length} type
* @param y as {@link Length} type
* @param width as {@link Length} type
* @param height as {@link Length} type
*/
public Rectangle(Length x, Length y, Length width, Length height) {
this(x.getValue(), y.getValue(), width.getValue(), height.getValue());
}
/**
* Construct {@link Rectangle} object from values and a common unit.
* @param x as numerical value
* @param y as numerical value
* @param width as numerical value
* @param height as numerical value
* @param unit the unit
*/
public Rectangle(double x, double y, double width, double height, Units unit) {
this(new Length(x, unit), new Length(y, unit), new Length(width, unit), new Length(height, unit));
}
/**
* Create a {@link Rectangle} object from the four edges left, bottom, right and top as {@link Length} objects.
* @param left as {@link Length} object
* @param bottom as {@link Length} object
* @param right as {@link Length} object
* @param top as {@link Length} object
* @return
*/
public static Rectangle fromEdges(Length left, Length bottom, Length right, Length top) {
return new Rectangle(left, bottom, right.subtract(left), top.subtract(bottom));
}
/**
* Create a {@link Rectangle} object from a string representation.
* @param value Allowed are value-unit pairs of the form "<x_value><x_unit> <y_value><y_unit> <right_value><right_unit> <height_value><height_unit>". Example: "12.3cm 23.4mm 34.5mm 45.6mm"
* @return
*/
public static Rectangle parse(String value) {
Length[] lengths = Length.parseArray(value, 4);
return new Rectangle(lengths[0], lengths[1], lengths[2], lengths[3]);
}
/**
* Creates a string representation as x-y-width-height-tuple with associated suitable metric units, "m", "cm" or "mm".
*/
@Override
public String toString() {
return this.getPosition().toString() + " " + this.getSize().toString();
}
/**
* Creates a string representation as x-y-width-height-tuple with the specified unit.
*/
public String toString(Units unit) {
return this.getPosition().toString(unit) + " " + this.getSize().toString(unit);
}
/**
* Determines whether another rectangle is non-strictly inscribed into the present rectangle.
* @param other as {@link Rectangle} object
* @return
*/
public boolean contains(Rectangle other)
{
Rectangle thisNorm = this.normalize();
Rectangle otherNorm = other.normalize();
return thisNorm.getLeft().getValue() <= otherNorm.getLeft().getValue() && thisNorm.getRight().getValue() >= otherNorm.getRight().getValue() &&
thisNorm.getTop().getValue() >= otherNorm.getTop().getValue() && thisNorm.getBottom().getValue() <= otherNorm.getBottom().getValue();
}
private Rectangle normalize()
{
double normalizedLeft = Math.min(this.getLeft().getValue(), this.getRight().getValue());
double normalizedRight = Math.max(this.getLeft().getValue(), this.getRight().getValue());
double normalizedBottom = Math.min(this.getBottom().getValue(), this.getTop().getValue());
double normalizedTop = Math.max(this.getBottom().getValue(), this.getTop().getValue());
return new Rectangle(normalizedLeft, normalizedBottom, normalizedRight - normalizedLeft, normalizedTop - normalizedLeft);
}
/**
* Get the position (origin) of the rectangle as {@link Point} object.
*/
public Point getPosition() {
return new Point(new Length(x, Units.POINT), new Length(y, Units.POINT));
}
/**
* Get the size of the rectangle as {@link Size} object.
*/
public Size getSize() {
return new Size(new Length(width, Units.POINT), new Length(height, Units.POINT));
}
/**
* Gets the left coordinate of the rectangle as {@link Length} object.
*/
public Length getLeft() {
return getPosition().getX();
}
/**
* Gets the top coordinate of the rectangle as {@link Length} object.
*/
public Length getTop() {
return getPosition().getY().add(getSize().getHeight());
}
/**
* Gets the right coordinate of the rectangle as {@link Length} object.
*/
public Length getRight() {
return getPosition().getX().add(getSize().getWidth());
}
/**
* Gets the bottom coordinate of the rectangle as {@link Length} object.
*/
public Length getBottom() {
return getPosition().getY();
}
@Override
public boolean equals(Object obj)
{
if (obj != null && obj instanceof Rectangle)
{
if (((Rectangle) obj).x != x)
return false;
if (((Rectangle) obj).y != y)
return false;
if (((Rectangle) obj).width != width)
return false;
if (((Rectangle) obj).height != height)
return false;
return true;
}
return false;
}
@Override
public int hashCode() {
return Objects.hash(x, y, width, height);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy