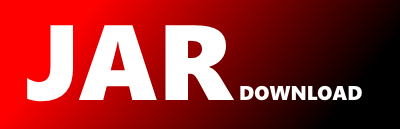
com.pdftools.image.DocumentList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
The newest version!
/****************************************************************************
*
* File: DocumentList.java
*
* Description: PDFTOOLS DocumentList Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.image;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
import java.lang.reflect.Array;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
/**
* List of image documents
*/
public class DocumentList extends NativeObject implements List
{
protected DocumentList(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static DocumentList createDynamicObject(long handle)
{
return new DocumentList(handle);
}
/**
*
*/
public DocumentList()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
private static native long newNative();
/////////////////////////////////////////////////////
// List implementation
/////////////////////////////////////////////////////
@Override
public boolean addAll(Collection extends com.pdftools.image.Document> elements)
{
if (elements == null)
throw new NullPointerException("'elements'");
if (elements.isEmpty())
return false;
for (com.pdftools.image.Document element : elements)
add(element);
return true;
}
@Override
public boolean addAll(int index, Collection extends com.pdftools.image.Document> elements)
{
if (elements == null)
throw new NullPointerException("'elements'");
if (elements.isEmpty())
return false;
for (com.pdftools.image.Document element : elements)
add(index++, element);
return true;
}
@Override
public boolean contains(Object object)
{
if (object == null)
throw new NullPointerException("'object'");
for (com.pdftools.image.Document element : this)
if (element.equals(object))
return true;
return false;
}
@Override
public boolean containsAll(Collection> objects)
{
for (Object element : objects)
if (!contains(element))
return false;
return true;
}
@Override
public boolean isEmpty()
{
return size() == 0;
}
@Override
public Iterator iterator()
{
return listIterator();
}
@Override
public ListIterator listIterator()
{
return listIterator(0);
}
@Override
public ListIterator listIterator(int index)
{
return new IndexedIterator(this, index);
}
/**
* Not supported.
*/
@Override
public boolean remove(Object object)
{
if (object == null)
throw new NullPointerException("'object'");
int index = indexOf(object);
if (index < 0)
return false;
remove(index);
return true;
}
/**
* Not supported.
*/
@Override
public boolean removeAll(Collection> objects)
{
if (objects == null)
throw new NullPointerException("'objects'");
boolean changed = false;
for (Object object : objects)
changed = remove(object) || changed;
return changed;
}
/**
* Not supported.
*/
@Override
public boolean retainAll(Collection> objects)
{
if (objects == null)
throw new NullPointerException("'objects'");
boolean changed = false;
Iterator it = this.iterator();
while (it.hasNext())
{
if (!objects.contains(it.next()))
{
it.remove();
changed = true;
}
}
return changed;
}
@Override
public List subList(int fromIndex, int toIndex)
{
return new SubList(this, fromIndex, toIndex - fromIndex);
}
@Override
public Object[] toArray()
{
Object[] array = new Object[size()];
for (int index = 0; index < size(); index++)
array[index] = get(index);
return array;
}
@SuppressWarnings("unchecked")
@Override
public T[] toArray(T[] array)
{
int size = size();
if (array.length < size)
{
// If array is too small, allocate the new one with the same
// component type
array = (T[]) Array.newInstance(array.getClass().getComponentType(), size);
}
else if (array.length > size)
{
// If array is to large, set the first unassigned element to null
array[size] = null;
}
int i = 0;
for (com.pdftools.image.Document e : this)
{
// No need for checked cast - ArrayStoreException will be thrown
// if types are incompatible, just as required
array[i] = (T) e;
i++;
}
return array;
}
@Override
public int size()
{
int n = sizeNative(getHandle());
if (n == -1)
throwLastRuntimeException(false);
return n;
}
/**
* Not supported.
*/
@Override
public void clear()
{
throw new UnsupportedOperationException("Method 'clear' is not supported by this class.");
}
@Override
public int indexOf(Object obj)
{
if (obj == null)
throw new NullPointerException("'obj'");
for (int index = 0; index < size(); index++)
if (obj.equals(get(index)))
return index;
return -1;
}
@Override
public int lastIndexOf(Object obj)
{
if (obj == null)
throw new NullPointerException("'obj'");
for (int index = size() - 1; index >= 0; index--)
if (obj.equals(get(index)))
return index;
return -1;
}
@Override
public boolean add(com.pdftools.image.Document element)
{
if (element == null)
throw new NullPointerException("'element'");
if (!addNative(getHandle(), getHandle(element), element))
throwLastRuntimeException(false);
return true;
}
/**
* Not supported.
*/
@Override
public void add(int index, com.pdftools.image.Document element)
{
if (element == null)
throw new NullPointerException("'element'");
if (!insertNative(getHandle(), index, getHandle(element), element))
throwLastRuntimeException(false);
}
@Override
public com.pdftools.image.Document get(int index)
{
long handle = getNative(getHandle(), index);
return createObject(handle);
}
/**
* Not supported.
*/
@Override
public com.pdftools.image.Document remove(int index)
{
throw new UnsupportedOperationException("Method 'remove' is not supported by this class.");
}
/**
* Not supported.
*/
@Override
public com.pdftools.image.Document set(int index, com.pdftools.image.Document element)
{
throw new UnsupportedOperationException("Method 'set' is not supported by this class.");
}
protected com.pdftools.image.Document createObject(long handle)
{
if (handle == 0)
throwLastRuntimeException(false);
return com.pdftools.image.Document.createDynamicObject(handle);
}
private native int sizeNative(long handle);
private native boolean addNative(long handle, long elementHandle, com.pdftools.image.Document element);
private native boolean insertNative(long handle, int index, long elementHandle, com.pdftools.image.Document element);
private native long getNative(long handle, int index);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy