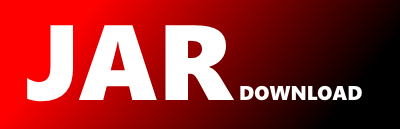
com.pdftools.image.ListCommon Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
The newest version!
/****************************************************************************
*
* File: ListCommon.java
*
* Description: PDFTOOLS internal classes of list implementations.
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.image;
import java.util.AbstractList;
import java.util.List;
import java.util.ListIterator;
import java.util.NoSuchElementException;
/**
* @hidden
* In Java, the file name should be always the same as a class name.
* This dummy class is to fulfill that requirement because some tools, e.g. javadoc, cannot process the file otherwise.
*/
class ListCommon
{}
class SubList extends AbstractList
{
SubList(List parent, int startIndex, int size)
{
this.parent = parent;
this.startIndex = startIndex;
this.size = size;
}
@Override
public E get(int index)
{
if (index < 0 || index >= size)
throw new IndexOutOfBoundsException();
return parent.get(index + startIndex);
}
@Override
public int size()
{
return size;
}
@Override
public void add(int index, E element)
{
if (index < 0 || index > size)
throw new IndexOutOfBoundsException();
parent.add(index + startIndex, element);
size++;
}
@Override
public E remove(int index)
{
if (index < 0 || index >= size)
throw new IndexOutOfBoundsException();
E element = parent.remove(index + startIndex);
size--;
return element;
}
private List parent;
private int startIndex;
private int size;
}
class IndexedIterator implements ListIterator
{
IndexedIterator(List list, int index)
{
this.list = list;
this.index = index;
this.lastIndex = -1;
}
@Override
public void add(E e)
{
list.add(index++, e);
lastIndex = -1;
}
@Override
public boolean hasNext()
{
return index < list.size();
}
@Override
public boolean hasPrevious()
{
return index > 0;
}
@Override
public E next()
{
if (!hasNext())
throw new NoSuchElementException();
lastIndex = index++;
return list.get(lastIndex);
}
@Override
public int nextIndex()
{
return index;
}
@Override
public E previous()
{
if (!hasPrevious())
throw new NoSuchElementException();
lastIndex = --index;
return list.get(lastIndex);
}
@Override
public int previousIndex()
{
return index - 1;
}
@Override
public void remove()
{
if (lastIndex < 0)
throw new IllegalStateException();
list.remove(lastIndex);
index = lastIndex;
lastIndex = -1;
}
@Override
public void set(E e)
{
if (lastIndex < 0 || lastIndex >= list.size())
throw new IllegalStateException();
list.set(lastIndex, e);
}
List list;
int index;
int lastIndex;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy