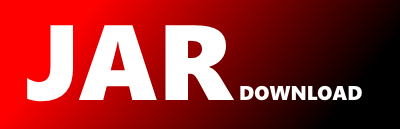
com.pdftools.image2pdf.Auto Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: Auto.java
*
* Description: PDFTOOLS Auto Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.image2pdf;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The image mapping that automatically determines a suitable conversion
*
* Images with a meaningful resolution, e.g. scans or graphics,
* are converted to PDF pages fitting the image. The
* image size is preserved if it is smaller than {@link Auto#getMaxPageSize }.
* Otherwise, it is scaled down.
* For all images except scans, a margin {@link Auto#getDefaultPageMargin } is used.
*
* Images with no meaningful resolution, e.g. photos are scaled, to fit onto
* {@link Auto#getMaxPageSize }.
*
*/
public class Auto extends com.pdftools.image2pdf.ImageMapping
{
protected Auto(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Auto createDynamicObject(long handle)
{
return new Auto(handle);
}
/**
*
*/
public Auto()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* The maximum page size (Getter)
*
* Each image is scaled individually such that neither the
* width nor the height exceeds the maximum page size.
* For landscape images the maximum page size is assumed
* to be landscape, and equivalently for portrait images.
*
* Default value: "A4" (210mm 297mm)
*
*/
public com.pdftools.geometry.units.Size getMaxPageSize()
{
com.pdftools.geometry.units.Size retVal = getMaxPageSizeNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The maximum page size (Setter)
*
* Each image is scaled individually such that neither the
* width nor the height exceeds the maximum page size.
* For landscape images the maximum page size is assumed
* to be landscape, and equivalently for portrait images.
*
* Default value: "A4" (210mm 297mm)
*
*
* @throws IllegalArgumentException The argument is smaller than "3pt 3pt" or larger than "14400pt 14400pt".
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setMaxPageSize(com.pdftools.geometry.units.Size value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setMaxPageSizeNative(getHandle(), value.getWidthValue(), value.getHeightValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The default page margin (Getter)
* Default value: 20mm (0.79in)
*/
public com.pdftools.geometry.units.Margin getDefaultPageMargin()
{
com.pdftools.geometry.units.Margin retVal = getDefaultPageMarginNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The default page margin (Setter)
* Default value: 20mm (0.79in)
*
* @throws IllegalArgumentException The argument has negative margin values.
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setDefaultPageMargin(com.pdftools.geometry.units.Margin value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setDefaultPageMarginNative(getHandle(), value.getLeftValue(), value.getBottomValue(), value.getRightValue(), value.getTopValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
private static native long newNative();
private native com.pdftools.geometry.units.Size getMaxPageSizeNative(long handle);
private native boolean setMaxPageSizeNative(long handle, double valueWidth, double valueHeight);
private native com.pdftools.geometry.units.Margin getDefaultPageMarginNative(long handle);
private native boolean setDefaultPageMarginNative(long handle, double valueLeft, double valueBottom, double valueRight, double valueTop);
}