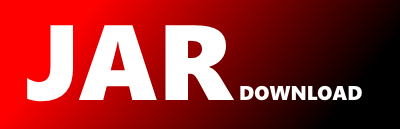
com.pdftools.image2pdf.Converter Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: Converter.java
*
* Description: PDFTOOLS Converter Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.image2pdf;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The class to convert one or more images to a PDF document
*/
public class Converter extends NativeObject
{
protected Converter(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Converter createDynamicObject(long handle)
{
return new Converter(handle);
}
/**
*
*/
public Converter()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* Convert an image to a PDF document
* @param image
* The input image document containing one or more pages.
* @param outStream
* The stream to which the PDF is written.
* @param profile
*
* The profile defines the properties of the output document and
* how the images are placed onto the pages.
*
* For details, see {@link com.pdftools.image2pdf.profiles.Profile profiles.Profile}.
*
* @return
*
* The resulting output PDF which can be used as a new input
* for further processing.
*
* Note that, this object must be disposed before the output stream
* object (method argument {@link com.pdftools.image2pdf.Converter#convert outStream}).
*
*
* @throws com.pdftools.LicenseException The license is invalid.
* @throws java.io.IOException Writing to the output PDF failed.
* @throws com.pdftools.CorruptException The input image document is corrupt and cannot be read.
* @throws com.pdftools.GenericException An unexpected failure occurred.
* @throws com.pdftools.ProcessingException The conversion failed.
* @throws IllegalArgumentException The {@link com.pdftools.image2pdf.Converter#convert profile} specifies invalid options.
* @throws IllegalArgumentException The {@link com.pdftools.image2pdf.Converter#convert outOptions} specifies document encryption and the {@link com.pdftools.image2pdf.Converter#convert profile} PDF/A conformance, which is not allowed.
* @throws IllegalArgumentException if {@code image} is {@code null}
* @throws IllegalArgumentException if {@code outStream} is {@code null}
* @throws IllegalArgumentException if {@code profile} is {@code null}
*/
public com.pdftools.pdf.Document convert(com.pdftools.image.Document image, com.pdftools.sys.Stream outStream, com.pdftools.image2pdf.profiles.Profile profile)
throws
java.io.IOException,
com.pdftools.GenericException,
com.pdftools.LicenseException,
com.pdftools.CorruptException,
com.pdftools.ProcessingException
{
if (image == null)
throw new IllegalArgumentException("Argument 'image' must not be null.", new NullPointerException("'image'"));
if (outStream == null)
throw new IllegalArgumentException("Argument 'outStream' must not be null.", new NullPointerException("'outStream'"));
if (profile == null)
throw new IllegalArgumentException("Argument 'profile' must not be null.", new NullPointerException("'profile'"));
return convert(image, outStream, profile, null);
}
/**
* Convert an image to a PDF document
* @param image
* The input image document containing one or more pages.
* @param outStream
* The stream to which the PDF is written.
* @param profile
*
* The profile defines the properties of the output document and
* how the images are placed onto the pages.
*
* For details, see {@link com.pdftools.image2pdf.profiles.Profile profiles.Profile}.
*
* @param outOptions
* The PDF output options, e.g. to encrypt the output document.
* @return
*
* The resulting output PDF which can be used as a new input
* for further processing.
*
* Note that, this object must be disposed before the output stream
* object (method argument {@link com.pdftools.image2pdf.Converter#convert outStream}).
*
*
* @throws com.pdftools.LicenseException The license is invalid.
* @throws java.io.IOException Writing to the output PDF failed.
* @throws com.pdftools.CorruptException The input image document is corrupt and cannot be read.
* @throws com.pdftools.GenericException An unexpected failure occurred.
* @throws com.pdftools.ProcessingException The conversion failed.
* @throws IllegalArgumentException The {@link com.pdftools.image2pdf.Converter#convert profile} specifies invalid options.
* @throws IllegalArgumentException The {@link com.pdftools.image2pdf.Converter#convert outOptions} specifies document encryption and the {@link com.pdftools.image2pdf.Converter#convert profile} PDF/A conformance, which is not allowed.
* @throws IllegalArgumentException if {@code image} is {@code null}
* @throws IllegalArgumentException if {@code outStream} is {@code null}
* @throws IllegalArgumentException if {@code profile} is {@code null}
*/
public com.pdftools.pdf.Document convert(com.pdftools.image.Document image, com.pdftools.sys.Stream outStream, com.pdftools.image2pdf.profiles.Profile profile, com.pdftools.pdf.OutputOptions outOptions)
throws
java.io.IOException,
com.pdftools.GenericException,
com.pdftools.LicenseException,
com.pdftools.CorruptException,
com.pdftools.ProcessingException
{
if (image == null)
throw new IllegalArgumentException("Argument 'image' must not be null.", new NullPointerException("'image'"));
if (outStream == null)
throw new IllegalArgumentException("Argument 'outStream' must not be null.", new NullPointerException("'outStream'"));
if (profile == null)
throw new IllegalArgumentException("Argument 'profile' must not be null.", new NullPointerException("'profile'"));
long retHandle = convertNative(getHandle(), getHandle(image), image, outStream, getHandle(profile), profile, getHandle(outOptions), outOptions);
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
case 4: throw new java.io.IOException(getLastErrorMessage());
case 10: throw new com.pdftools.GenericException(getLastErrorMessage());
case 12: throw new com.pdftools.LicenseException(getLastErrorMessage());
case 16: throw new com.pdftools.CorruptException(getLastErrorMessage());
case 21: throw new com.pdftools.ProcessingException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return com.pdftools.pdf.Document.createDynamicObject(retHandle);
}
/**
* Convert a list of images to a PDF document
* @param images
* The input image document list, each image containing one or more pages.
* @param outStream
* The stream to which the PDF is written.
* @param profile
*
* The profile defines the properties of the output document and
* how the images are placed onto the pages.
*
* For details, see {@link com.pdftools.image2pdf.profiles.Profile profiles.Profile}.
*
* @return
*
* The resulting output PDF which can be used as a new input
* for further processing.
*
* Note that, this object must be disposed before the output stream
* object (method argument {@link com.pdftools.image2pdf.Converter#convertMultiple outStream}).
*
*
* @throws com.pdftools.LicenseException The license is invalid.
* @throws java.io.IOException Writing to the output PDF failed.
* @throws com.pdftools.CorruptException An input image document is corrupt and cannot be read.
* @throws com.pdftools.GenericException An unexpected failure occurred.
* @throws com.pdftools.ProcessingException The conversion failed.
* @throws IllegalArgumentException The {@link com.pdftools.image2pdf.Converter#convertMultiple profile} specifies invalid options.
* @throws IllegalArgumentException The {@link com.pdftools.image2pdf.Converter#convertMultiple outOptions} specifies document encryption and the {@link com.pdftools.image2pdf.Converter#convertMultiple profile} PDF/A conformance, which is not allowed.
* @throws IllegalArgumentException if {@code images} is {@code null}
* @throws IllegalArgumentException if {@code outStream} is {@code null}
* @throws IllegalArgumentException if {@code profile} is {@code null}
*/
public com.pdftools.pdf.Document convertMultiple(com.pdftools.image.DocumentList images, com.pdftools.sys.Stream outStream, com.pdftools.image2pdf.profiles.Profile profile)
throws
java.io.IOException,
com.pdftools.GenericException,
com.pdftools.LicenseException,
com.pdftools.CorruptException,
com.pdftools.ProcessingException
{
if (images == null)
throw new IllegalArgumentException("Argument 'images' must not be null.", new NullPointerException("'images'"));
if (outStream == null)
throw new IllegalArgumentException("Argument 'outStream' must not be null.", new NullPointerException("'outStream'"));
if (profile == null)
throw new IllegalArgumentException("Argument 'profile' must not be null.", new NullPointerException("'profile'"));
return convertMultiple(images, outStream, profile, null);
}
/**
* Convert a list of images to a PDF document
* @param images
* The input image document list, each image containing one or more pages.
* @param outStream
* The stream to which the PDF is written.
* @param profile
*
* The profile defines the properties of the output document and
* how the images are placed onto the pages.
*
* For details, see {@link com.pdftools.image2pdf.profiles.Profile profiles.Profile}.
*
* @param outOptions
* The PDF output options, e.g. to encrypt the output document.
* @return
*
* The resulting output PDF which can be used as a new input
* for further processing.
*
* Note that, this object must be disposed before the output stream
* object (method argument {@link com.pdftools.image2pdf.Converter#convertMultiple outStream}).
*
*
* @throws com.pdftools.LicenseException The license is invalid.
* @throws java.io.IOException Writing to the output PDF failed.
* @throws com.pdftools.CorruptException An input image document is corrupt and cannot be read.
* @throws com.pdftools.GenericException An unexpected failure occurred.
* @throws com.pdftools.ProcessingException The conversion failed.
* @throws IllegalArgumentException The {@link com.pdftools.image2pdf.Converter#convertMultiple profile} specifies invalid options.
* @throws IllegalArgumentException The {@link com.pdftools.image2pdf.Converter#convertMultiple outOptions} specifies document encryption and the {@link com.pdftools.image2pdf.Converter#convertMultiple profile} PDF/A conformance, which is not allowed.
* @throws IllegalArgumentException if {@code images} is {@code null}
* @throws IllegalArgumentException if {@code outStream} is {@code null}
* @throws IllegalArgumentException if {@code profile} is {@code null}
*/
public com.pdftools.pdf.Document convertMultiple(com.pdftools.image.DocumentList images, com.pdftools.sys.Stream outStream, com.pdftools.image2pdf.profiles.Profile profile, com.pdftools.pdf.OutputOptions outOptions)
throws
java.io.IOException,
com.pdftools.GenericException,
com.pdftools.LicenseException,
com.pdftools.CorruptException,
com.pdftools.ProcessingException
{
if (images == null)
throw new IllegalArgumentException("Argument 'images' must not be null.", new NullPointerException("'images'"));
if (outStream == null)
throw new IllegalArgumentException("Argument 'outStream' must not be null.", new NullPointerException("'outStream'"));
if (profile == null)
throw new IllegalArgumentException("Argument 'profile' must not be null.", new NullPointerException("'profile'"));
long retHandle = convertMultipleNative(getHandle(), getHandle(images), images, outStream, getHandle(profile), profile, getHandle(outOptions), outOptions);
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
case 4: throw new java.io.IOException(getLastErrorMessage());
case 10: throw new com.pdftools.GenericException(getLastErrorMessage());
case 12: throw new com.pdftools.LicenseException(getLastErrorMessage());
case 16: throw new com.pdftools.CorruptException(getLastErrorMessage());
case 21: throw new com.pdftools.ProcessingException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return com.pdftools.pdf.Document.createDynamicObject(retHandle);
}
private static native long newNative();
private native long convertNative(long handle, long image, com.pdftools.image.Document imageObj, com.pdftools.sys.Stream outStream, long profile, com.pdftools.image2pdf.profiles.Profile profileObj, long outOptions, com.pdftools.pdf.OutputOptions outOptionsObj);
private native long convertMultipleNative(long handle, long images, com.pdftools.image.DocumentList imagesObj, com.pdftools.sys.Stream outStream, long profile, com.pdftools.image2pdf.profiles.Profile profileObj, long outOptions, com.pdftools.pdf.OutputOptions outOptionsObj);
}