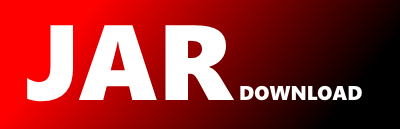
com.pdftools.internal.NativeStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
/****************************************************************************
*
* File: NativeStream.java
*
* Description: System Native Stream Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.internal;
import com.pdftools.sys.*;
import java.io.IOException;
/**
* @hidden
*/
public class NativeStream implements Stream
{
private long handle;
private long readHandle;
private long getLengthHandle;
private long seekHandle;
private long tellHandle;
private long writeHandle;
private long releaseHandle;
/**
* Get the length of the stream in bytes
* @return the length of the stream in bytes
*/
public long getLength()
throws IOException
{
if (handle == 0)
throw new IllegalStateException();
long retVal = getLengthNative(handle, getLengthHandle);
if (retVal == -1)
throwLastError();
return retVal;
}
/**
* Set byte position
* @param position The new position of the stream (-1 for EOS)
* @return true if successful
*/
public boolean seek(long position)
throws IOException
{
if (handle == 0)
throw new IllegalStateException();
if (!seekNative(handle, seekHandle, position))
throwLastError();
return true;
}
/**
* Get current byte position
* @return byte position, -1 if position unknown
*/
public long tell()
throws IOException
{
if (handle == 0)
throw new IllegalStateException();
long retVal = tellNative(handle, tellHandle);
if (retVal == -1)
throwLastError();
return retVal;
}
/**
* Read from the stream
* @param buffer The buffer where the data is written
* @param offset The starting element in the buffer
* @param length The maximum number of bytes to be read
* @return The actual number of bytes read (-1 if EOS)
*/
public int read(byte[] buffer, int offset, int length)
throws IOException
{
if (handle == 0)
throw new IllegalStateException();
int retVal = readNative(handle, readHandle, buffer, offset, length);
if (retVal == -1)
throwLastError();
return retVal;
}
/**
* Write to the stream
* @param buffer The buffer where the data lies
* @param offset The starting element in the buffer
* @param length The maximum number of bytes to be written
*/
public void write(byte[] buffer, int offset, int length)
throws IOException
{
if (handle == 0)
throw new IllegalStateException();
long retVal = writeNative(handle, writeHandle, buffer, offset, length);
if (retVal == -1)
throwLastError();
}
/**
* Copy the content of another stream
* @param stream The stream of which the content is copied
*/
public void copy(Stream stream)
throws IOException
{
if (handle == 0)
throw new IllegalStateException();
byte[] buffer = new byte[8192];
int read;
while ((read = stream.read(buffer, 0, 8192)) >= 0)
write(buffer, 0, read);
}
/**
* Close the stream
*/
public void close()
throws IOException
{
if (handle == 0)
throw new IllegalStateException();
try
{
closeNative(handle, releaseHandle);
}
finally
{
handle = 0;
}
}
protected void finalize()
throws Throwable
{
try
{
finalize(handle);
}
finally
{
super.finalize();
}
}
private void throwLastError()
throws IOException
{
switch (NativeBase.getLastErrorCode())
{
case 1: throw new UnsupportedOperationException(NativeBase.getLastErrorMessage());
case 2: throw new IllegalStateException(NativeBase.getLastErrorMessage());
case 3: throw new IllegalArgumentException(NativeBase.getLastErrorMessage());
default: NativeBase.throwLastRuntimeException(false);
}
}
private static native int readNative(long handle, long readHandle, byte[] buffer, int offset, int length);
private static native long getLengthNative(long handle, long getLengthHandle);
private static native boolean seekNative(long handle, long seekHandle, long position);
private static native long tellNative(long handle, long tellHandle);
private static native long writeNative(long handle, long writeHandle, byte[] buffer, int offset, int length);
private static native void closeNative(long handle, long releaseHandle);
private static native void finalize(long hObject);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy