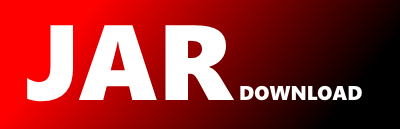
com.pdftools.optimization.RemovalOptions Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: RemovalOptions.java
*
* Description: PDFTOOLS RemovalOptions Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.optimization;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The parameters defining the optional data to remove or flatten
*
* Removal options specify the PDF data structures to copy or remove,
* e.g. article threads, metadata, or alternate images.
*
* In addition, the visual appearances of signatures, annotations, form fields,
* and links can be flattened.
*
* Flattening means, that the appearance of such a data structure is drawn as
* non-editable graphic onto the page; for visual appearances of signatures,
* flattening has a slightly different meaning
* (see property {@link RemovalOptions#getRemoveSignatureAppearances }).
*
*/
public class RemovalOptions extends NativeObject
{
protected RemovalOptions(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static RemovalOptions createDynamicObject(long handle)
{
return new RemovalOptions(handle);
}
/**
* Whether to remove additional or alternative versions of images (Getter)
* Default: {@code false} except in the profile {@link com.pdftools.optimization.profiles.Print profiles.Print}.
*/
public boolean getRemoveAlternateImages()
{
boolean retVal = getRemoveAlternateImagesNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether to remove additional or alternative versions of images (Setter)
* Default: {@code false} except in the profile {@link com.pdftools.optimization.profiles.Print profiles.Print}.
*/
public void setRemoveAlternateImages(boolean value)
{
boolean retVal = setRemoveAlternateImagesNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Whether to remove the sequential flows (threads) of articles (Getter)
* Default: {@code true} except in the profile {@link com.pdftools.optimization.profiles.Archive profiles.Archive}.
*/
public boolean getRemoveArticleThreads()
{
boolean retVal = getRemoveArticleThreadsNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether to remove the sequential flows (threads) of articles (Setter)
* Default: {@code true} except in the profile {@link com.pdftools.optimization.profiles.Archive profiles.Archive}.
*/
public void setRemoveArticleThreads(boolean value)
{
boolean retVal = setRemoveArticleThreadsNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Whether to remove document's XMP metadata (Getter)
* Default:
*
* -
* {@link com.pdftools.optimization.profiles.Web profiles.Web} profile: {@code true}
* -
* {@link com.pdftools.optimization.profiles.Print profiles.Print} profile: {@code false}
* -
* {@link com.pdftools.optimization.profiles.Archive profiles.Archive} profile: {@code false}
* -
* {@link com.pdftools.optimization.profiles.MinimalFileSize profiles.MinimalFileSize} profile: {@code true}
* -
* {@link com.pdftools.optimization.profiles.Mrc profiles.Mrc} profile: {@code false}
*
*/
public boolean getRemoveMetadata()
{
boolean retVal = getRemoveMetadataNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether to remove document's XMP metadata (Setter)
* Default:
*
* -
* {@link com.pdftools.optimization.profiles.Web profiles.Web} profile: {@code true}
* -
* {@link com.pdftools.optimization.profiles.Print profiles.Print} profile: {@code false}
* -
* {@link com.pdftools.optimization.profiles.Archive profiles.Archive} profile: {@code false}
* -
* {@link com.pdftools.optimization.profiles.MinimalFileSize profiles.MinimalFileSize} profile: {@code true}
* -
* {@link com.pdftools.optimization.profiles.Mrc profiles.Mrc} profile: {@code false}
*
*/
public void setRemoveMetadata(boolean value)
{
boolean retVal = setRemoveMetadataNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Whether to remove all output intents (Getter)
*
* Output intents provide a means for matching the color characteristics
* of PDF page content with those of a target output device or
* production environment in which the document will be printed.
*
* Default: {@code false} except in the profile {@link com.pdftools.optimization.profiles.MinimalFileSize profiles.MinimalFileSize}.
*
*/
public boolean getRemoveOutputIntents()
{
boolean retVal = getRemoveOutputIntentsNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether to remove all output intents (Setter)
*
* Output intents provide a means for matching the color characteristics
* of PDF page content with those of a target output device or
* production environment in which the document will be printed.
*
* Default: {@code false} except in the profile {@link com.pdftools.optimization.profiles.MinimalFileSize profiles.MinimalFileSize}.
*
*/
public void setRemoveOutputIntents(boolean value)
{
boolean retVal = setRemoveOutputIntentsNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Whether to remove the piece-info dictionary (private PDF processor data) (Getter)
*
* The removal of this proprietary application data has no effect on the document's
* visual appearance.
*
* Default: {@code true} except in the profile {@link com.pdftools.optimization.profiles.Archive profiles.Archive}.
*
*/
public boolean getRemovePieceInfo()
{
boolean retVal = getRemovePieceInfoNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether to remove the piece-info dictionary (private PDF processor data) (Setter)
*
* The removal of this proprietary application data has no effect on the document's
* visual appearance.
*
* Default: {@code true} except in the profile {@link com.pdftools.optimization.profiles.Archive profiles.Archive}.
*
*/
public void setRemovePieceInfo(boolean value)
{
boolean retVal = setRemovePieceInfoNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Whether to remove the data describing the logical structure of a PDF (Getter)
*
* The logical structure of the document is a description of the content of its pages.
* It consists of a fine granular hierarchical tagging that distinguishes between the actual content and artifacts (such as page numbers, layout artifacts, etc.).
* The tagging provides a meaningful description, for example "This is a header", "This color image shows a small sailing boat at sunset", etc.
* This information can be used e.g. to read the document to the visually impaired.
*
* Default: {@code true} except in the profile {@link com.pdftools.optimization.profiles.Archive profiles.Archive}.
*
*/
public boolean getRemoveStructureTree()
{
boolean retVal = getRemoveStructureTreeNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether to remove the data describing the logical structure of a PDF (Setter)
*
* The logical structure of the document is a description of the content of its pages.
* It consists of a fine granular hierarchical tagging that distinguishes between the actual content and artifacts (such as page numbers, layout artifacts, etc.).
* The tagging provides a meaningful description, for example "This is a header", "This color image shows a small sailing boat at sunset", etc.
* This information can be used e.g. to read the document to the visually impaired.
*
* Default: {@code true} except in the profile {@link com.pdftools.optimization.profiles.Archive profiles.Archive}.
*
*/
public void setRemoveStructureTree(boolean value)
{
boolean retVal = setRemoveStructureTreeNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Whether to remove thumbnail images which represent the PDF pages in miniature form (Getter)
* Default: {@code true} in all profiles.
*/
public boolean getRemoveThumbnails()
{
boolean retVal = getRemoveThumbnailsNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether to remove thumbnail images which represent the PDF pages in miniature form (Setter)
* Default: {@code true} in all profiles.
*/
public void setRemoveThumbnails(boolean value)
{
boolean retVal = setRemoveThumbnailsNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Whether to remove or flatten signature appearances (Getter)
*
* A signature in a PDF consist of two parts:
*
*
* -
* (a) The invisible digital signature in the PDF.
* -
* (b) The visual appearance that was attributed to the signature.
*
*
* Part (a) can be used by a viewing application, to verify that the PDF
* has not changed since it has been signed and report this to the user.
*
* During optimizing, the PDF is altered and hence its digital signature
* (a) is broken and must be removed.
*
*
* -
* {@link RemovalStrategy#FLATTEN }: (a) is removed and (b) is drawn as non-editable graphic onto the page.
* Within the context of signatures this is called "flattening".
* -
* {@link RemovalStrategy#REMOVE }: (a) and (b) are removed.
*
*
* Default: {@link RemovalStrategy#FLATTEN } in all profiles.
*
*/
public com.pdftools.optimization.RemovalStrategy getRemoveSignatureAppearances()
{
int retVal = getRemoveSignatureAppearancesNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.optimization.RemovalStrategy.fromValue(retVal);
}
/**
* Whether to remove or flatten signature appearances (Setter)
*
* A signature in a PDF consist of two parts:
*
*
* -
* (a) The invisible digital signature in the PDF.
* -
* (b) The visual appearance that was attributed to the signature.
*
*
* Part (a) can be used by a viewing application, to verify that the PDF
* has not changed since it has been signed and report this to the user.
*
* During optimizing, the PDF is altered and hence its digital signature
* (a) is broken and must be removed.
*
*
* -
* {@link RemovalStrategy#FLATTEN }: (a) is removed and (b) is drawn as non-editable graphic onto the page.
* Within the context of signatures this is called "flattening".
* -
* {@link RemovalStrategy#REMOVE }: (a) and (b) are removed.
*
*
* Default: {@link RemovalStrategy#FLATTEN } in all profiles.
*
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setRemoveSignatureAppearances(com.pdftools.optimization.RemovalStrategy value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setRemoveSignatureAppearancesNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* The conversion strategy for annotations (Getter)
*
* The conversion strategy for annotations.
*
* Annotations in PDF are interactive elements on the pages, such as:
*
* -
* Sticky notes
* -
* Free text annotations
* -
* Line, square, circle, and polygon annotations
* -
* Highlight, underline and strikeout annotations
* -
* Stamp annotations
* -
* Ink annotations
* -
* File attachment annotation
* -
* Sound and movie annotations
* -
* 3D annotations
*
*
* Note that this does not include form fields (see {@link RemovalOptions#getFormFields }) and links (see {@link RemovalOptions#getLinks }).
*
* Default: {@link ConversionStrategy#COPY } in all profiles.
*
*/
public com.pdftools.optimization.ConversionStrategy getAnnotations()
{
int retVal = getAnnotationsNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.optimization.ConversionStrategy.fromValue(retVal);
}
/**
* The conversion strategy for annotations (Setter)
*
* The conversion strategy for annotations.
*
* Annotations in PDF are interactive elements on the pages, such as:
*
* -
* Sticky notes
* -
* Free text annotations
* -
* Line, square, circle, and polygon annotations
* -
* Highlight, underline and strikeout annotations
* -
* Stamp annotations
* -
* Ink annotations
* -
* File attachment annotation
* -
* Sound and movie annotations
* -
* 3D annotations
*
*
* Note that this does not include form fields (see {@link RemovalOptions#getFormFields }) and links (see {@link RemovalOptions#getLinks }).
*
* Default: {@link ConversionStrategy#COPY } in all profiles.
*
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setAnnotations(com.pdftools.optimization.ConversionStrategy value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setAnnotationsNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* The conversion strategy for interactive forms (Getter)
* Default: {@link ConversionStrategy#COPY } in all profiles.
*/
public com.pdftools.optimization.ConversionStrategy getFormFields()
{
int retVal = getFormFieldsNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.optimization.ConversionStrategy.fromValue(retVal);
}
/**
* The conversion strategy for interactive forms (Setter)
* Default: {@link ConversionStrategy#COPY } in all profiles.
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setFormFields(com.pdftools.optimization.ConversionStrategy value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setFormFieldsNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* The conversion strategy for links (Getter)
* Default: {@link ConversionStrategy#COPY } in all profiles.
*/
public com.pdftools.optimization.ConversionStrategy getLinks()
{
int retVal = getLinksNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.optimization.ConversionStrategy.fromValue(retVal);
}
/**
* The conversion strategy for links (Setter)
* Default: {@link ConversionStrategy#COPY } in all profiles.
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setLinks(com.pdftools.optimization.ConversionStrategy value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setLinksNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
private native boolean getRemoveAlternateImagesNative(long handle);
private native boolean setRemoveAlternateImagesNative(long handle, boolean value);
private native boolean getRemoveArticleThreadsNative(long handle);
private native boolean setRemoveArticleThreadsNative(long handle, boolean value);
private native boolean getRemoveMetadataNative(long handle);
private native boolean setRemoveMetadataNative(long handle, boolean value);
private native boolean getRemoveOutputIntentsNative(long handle);
private native boolean setRemoveOutputIntentsNative(long handle, boolean value);
private native boolean getRemovePieceInfoNative(long handle);
private native boolean setRemovePieceInfoNative(long handle, boolean value);
private native boolean getRemoveStructureTreeNative(long handle);
private native boolean setRemoveStructureTreeNative(long handle, boolean value);
private native boolean getRemoveThumbnailsNative(long handle);
private native boolean setRemoveThumbnailsNative(long handle, boolean value);
private native int getRemoveSignatureAppearancesNative(long handle);
private native boolean setRemoveSignatureAppearancesNative(long handle, int value);
private native int getAnnotationsNative(long handle);
private native boolean setAnnotationsNative(long handle, int value);
private native int getFormFieldsNative(long handle);
private native boolean setFormFieldsNative(long handle, int value);
private native int getLinksNative(long handle);
private native boolean setLinksNative(long handle, int value);
}