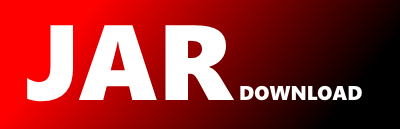
com.pdftools.optimization.profiles.Mrc Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: Mrc.java
*
* Description: PDFTOOLS Mrc Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.optimization.profiles;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The optimization profile suitable for documents with Mixed Raster Content
* Reduce the file size for documents containing large images, e.g. scanned pages, while maintaining the readability of text.
* This is accomplished by separating the images into a foreground, background and a mask layer.
* The foreground and background layers are heavily down-sampled and compressed.
* The textual information is stored in the mask with a lossless compression type.
* Additionally, redundant objects are removed, resources are optimized and embedded fonts are merged.
*/
public class Mrc extends com.pdftools.optimization.profiles.Profile
{
protected Mrc(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Mrc createDynamicObject(long handle)
{
return new Mrc(handle);
}
/**
*
*/
public Mrc()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* The image quality for MRC foreground and background layers (Getter)
*
* This is a value between {@code 0} (lowest quality) and {@code 1} (highest quality).
*
* Default:
*
* -
* {@link com.pdftools.optimization.CompressionAlgorithmSelection#PRESERVE_QUALITY pdftools.optimization.CompressionAlgorithmSelection.PRESERVE_QUALITY} algorithm: {@code 1}.
*
* -
* {@link com.pdftools.optimization.CompressionAlgorithmSelection#BALANCED pdftools.optimization.CompressionAlgorithmSelection.BALANCED} algorithm: {@code 0.25}.
*
* -
* {@link com.pdftools.optimization.CompressionAlgorithmSelection#SPEED pdftools.optimization.CompressionAlgorithmSelection.SPEED} algorithm: {@code 0.25}.
*
*
*/
public double getLayerCompressionQuality()
{
double retVal = getLayerCompressionQualityNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The image quality for MRC foreground and background layers (Setter)
*
* This is a value between {@code 0} (lowest quality) and {@code 1} (highest quality).
*
* Default:
*
* -
* {@link com.pdftools.optimization.CompressionAlgorithmSelection#PRESERVE_QUALITY pdftools.optimization.CompressionAlgorithmSelection.PRESERVE_QUALITY} algorithm: {@code 1}.
*
* -
* {@link com.pdftools.optimization.CompressionAlgorithmSelection#BALANCED pdftools.optimization.CompressionAlgorithmSelection.BALANCED} algorithm: {@code 0.25}.
*
* -
* {@link com.pdftools.optimization.CompressionAlgorithmSelection#SPEED pdftools.optimization.CompressionAlgorithmSelection.SPEED} algorithm: {@code 0.25}.
*
*
*
* @throws IllegalArgumentException The given value is smaller than {@code 0} or greater than {@code 1}.
*/
public void setLayerCompressionQuality(double value)
{
boolean retVal = setLayerCompressionQualityNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The target resolution in DPI (dots per inch) for downsampling MRC foreground and background layers (Getter)
*
* Valid values are 1, or 10000, or in between.
* Set to {@code null} to deactivate downsampling of images.
*
* Default: {@code 70}.
*
*/
public Double getLayerResolutionDPI()
{
Double retVal = getLayerResolutionDPINative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The target resolution in DPI (dots per inch) for downsampling MRC foreground and background layers (Setter)
*
* Valid values are 1, or 10000, or in between.
* Set to {@code null} to deactivate downsampling of images.
*
* Default: {@code 70}.
*
*
* @throws IllegalArgumentException The given value is smaller than 1 or greater than 10000.
*/
public void setLayerResolutionDPI(Double value)
{
boolean retVal = setLayerResolutionDPINative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The option to recognize photographic regions when doing MRC. (Getter)
*
* Regardless of this property’s setting, monochrome (grayscale) images are always treated as entire photographic regions (cutout pictures)
* by the MRC algorithm.
*
* Default: {@code false}.
*
*/
public boolean getRecognizePictures()
{
boolean retVal = getRecognizePicturesNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The option to recognize photographic regions when doing MRC. (Setter)
*
* Regardless of this property’s setting, monochrome (grayscale) images are always treated as entire photographic regions (cutout pictures)
* by the MRC algorithm.
*
* Default: {@code false}.
*
*/
public void setRecognizePictures(boolean value)
{
boolean retVal = setRecognizePicturesNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
private static native long newNative();
private native double getLayerCompressionQualityNative(long handle);
private native boolean setLayerCompressionQualityNative(long handle, double value);
private native Double getLayerResolutionDPINative(long handle);
private native boolean setLayerResolutionDPINative(long handle, Double value);
private native boolean getRecognizePicturesNative(long handle);
private native boolean setRecognizePicturesNative(long handle, boolean value);
}