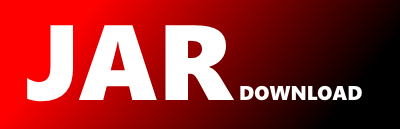
com.pdftools.optimization.profiles.Profile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
The newest version!
/****************************************************************************
*
* File: Profile.java
*
* Description: PDFTOOLS Profile Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.optimization.profiles;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The base class for PDF optimization profiles
* The profile defines the optimization parameters suitable for a particular
* use case, e.g. archiving, or publication on the web.
*/
public abstract class Profile extends NativeObject
{
protected Profile(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Profile createDynamicObject(long handle)
{
int type = getType(handle);
switch (type)
{
case 1:
return com.pdftools.optimization.profiles.Web.createDynamicObject(handle);
case 2:
return com.pdftools.optimization.profiles.Print.createDynamicObject(handle);
case 3:
return com.pdftools.optimization.profiles.Archive.createDynamicObject(handle);
case 4:
return com.pdftools.optimization.profiles.MinimalFileSize.createDynamicObject(handle);
case 5:
return com.pdftools.optimization.profiles.Mrc.createDynamicObject(handle);
default:
return null;
}
}
/**
* The image recompression options (Getter)
*
*/
public com.pdftools.optimization.ImageRecompressionOptions getImageRecompressionOptions()
{
long retHandle = getImageRecompressionOptionsNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.optimization.ImageRecompressionOptions.createDynamicObject(retHandle);
}
/**
* The font optimization options (Getter)
*
*/
public com.pdftools.optimization.FontOptions getFontOptions()
{
long retHandle = getFontOptionsNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.optimization.FontOptions.createDynamicObject(retHandle);
}
/**
* The parameters defining the optional data to remove or flatten (Getter)
*
*/
public com.pdftools.optimization.RemovalOptions getRemovalOptions()
{
long retHandle = getRemovalOptionsNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.optimization.RemovalOptions.createDynamicObject(retHandle);
}
/**
* Whether to copy metadata (Getter)
* Copy document information dictionary and XMP metadata.
* Default: {@code true}.
*/
public boolean getCopyMetadata()
{
boolean retVal = getCopyMetadataNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether to copy metadata (Setter)
* Copy document information dictionary and XMP metadata.
* Default: {@code true}.
*/
public void setCopyMetadata(boolean value)
{
boolean retVal = setCopyMetadataNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
private static native int getType(long handle);
private native long getImageRecompressionOptionsNative(long handle);
private native long getFontOptionsNative(long handle);
private native long getRemovalOptionsNative(long handle);
private native boolean getCopyMetadataNative(long handle);
private native boolean setCopyMetadataNative(long handle, boolean value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy