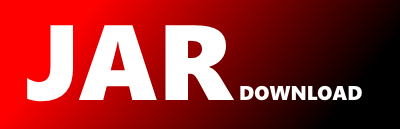
com.pdftools.pdf.Conformance Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: Conformance.java
*
* Description: Conformance class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.pdf;
import java.util.Collections;
import java.util.Map;
import java.util.Map.Entry;
import java.util.concurrent.ConcurrentHashMap;
import java.util.Objects;
/**
* The conformance of a PDF document
*/
public class Conformance
{
private static class COMPLIANCE
{
// public final static int ePDFUnk = 0x0000;
public final static int ePDF10 = 0x1000;
public final static int ePDF11 = 0x1100;
public final static int ePDF12 = 0x1200;
public final static int ePDF13 = 0x1300;
public final static int ePDF14 = 0x1400;
public final static int ePDF15 = 0x1500;
public final static int ePDF16 = 0x1600;
public final static int ePDF17 = 0x1700;
public final static int ePDF20 = 0x2000;
public final static int ePDFA1b = 0x1401;
public final static int ePDFA1a = 0x1402;
public final static int ePDFA2b = 0x1701;
public final static int ePDFA2u = 0x1702;
public final static int ePDFA2a = 0x1703;
public final static int ePDFA3b = 0x1711;
public final static int ePDFA3u = 0x1712;
public final static int ePDFA3a = 0x1713;
}
/**
* The PDF version number
*/
public static class Version implements Comparable
{
private int major;
private int minor;
public Version(int major, int minor)
{
this.major = major;
this.minor = minor;
}
public int getMajor()
{
return major;
}
public int getMinor()
{
return minor;
}
@Override
public String toString()
{
return String.format("%d.%d", major, minor);
}
@Override
public boolean equals(Object other)
{
if (other != null && other instanceof Version)
{
if (((Version) other).major != major)
return false;
if (((Version) other).minor != minor)
return false;
return true;
}
return false;
}
@Override
public int hashCode()
{
return Objects.hash(major, minor);
}
@Override
public int compareTo(Conformance.Version o)
{
if (major != o.major)
return major - o.major;
return minor - o.minor;
}
}
/**
* The attributes of a PDF/A version
*/
public static class PdfAVersion implements Comparable
{
/**
* The PDF/A conformance level that defines the requirements on the quality of the document's content.
*/
public enum Level
{
/**
* Level B (basic)
*
* Visual integrity - clear, long-term visual reproduction of static content.
*
*/
B(1),
/**
* Level U (Unicode)
*
* Searchability of text and copying of Unicode text for digitally created PDF documents and documents scanned using optical character recognition (OCR).
*
*
* This is in addition to level B. Therefore, all level U documents are also valid level B documents.
*
*/
U(2),
/**
* Level A (accessibility)
*
* Accessibility - semantic correctness and structure (tagged PDF). Archive PDF, including complete accessibility to all types of content.
*
*
* This is in addition to level U. Therefore, all level A documents are also valid level U and level B documents.
*
*/
A(3);
/**
* @hidden
*/
Level(int value)
{
this.value = value;
}
/**
* @hidden
*/
public int getValue()
{
return value;
}
private int value;
@Override
public String toString()
{
switch (this)
{
case B: return "B";
case U: return "U";
case A: return "A";
}
throw new IllegalArgumentException(String.format("Invalid PDF/A level %d.", value));
}
}
private int part;
private Level level;
/**
* Create a new PDF/A version.
*
* @param part The PDF/A part. Must be 1, 2, or 3 for PDF/A-1, PDF/A-2, or PDF/A-3 (see {@link PdfAVersion#getPart()}).
* @param level The conformance level. Must be supported by the PDF/A part (see {@link Level}).
*/
public PdfAVersion(int part, Level level)
{
this.part = part;
this.level = level;
}
/**
* The PDF/A part (Standard)
*
* There are currently three different PDF/A Standards supported.
*
* -
* PDF/A-1 (ISO 19005-1)
*
* PDF/A was published by the ISO in 2005 to support long-term archiving of PDF documents.
* The first release, PDF/A-1, was based on the original PDF 1.4 version where a set of standards criteria was introduced such as ensuring the visual reproducibility of PDF documents regardless of future changes to viewer and printing technologies, and making PDF documents accessible to persons with eye vision challenges.
*
*
* -
* PDF/A-2 (ISO 19005-2)
*
* In July 2011 the ISO released the PDF/A-2 standard that takes advantages of features that only became available in later versions of PDF, up to and including PDF version 1.7.
* This includes the following features: JPEG2000 compression, embedded PDF/A files, transparency, and optional content (layers).
*
*
* -
* PDF/A-3 (ISO 19005-3)
*
* The ISO Committee released the third edition of the standard in October 2012.
* PDF/A-3 contains just one change that is necessary but controversial:
* PDF/A-2 already enabled the embedding of PDF/A-conform document as attachments.
* PDF/A-3, however, makes it possible to embed any document format such as Excel, Word, HTML, CAD or XML files for the first time ever.
*
*
*
*/
public int getPart()
{
return part;
}
/**
* The PDF/A conformance level
*/
public Level getLevel()
{
return level;
}
@Override
public String toString()
{
return String.format("PFD/A-%d%s", part, level.toString().toLowerCase());
}
@Override
public boolean equals(Object other)
{
if (other != null && other instanceof PdfAVersion)
{
if (((PdfAVersion) other).part != part)
return false;
if (((PdfAVersion) other).level != level)
return false;
return true;
}
return false;
}
@Override
public int hashCode()
{
return Objects.hash(part, level);
}
@Override
public int compareTo(PdfAVersion o)
{
if (part != o.part)
return part - o.part;
return level.getValue() - o.level.getValue();
}
}
/**
* @hidden
*/
public Conformance(int value)
{
this.value = value;
}
/**
* @hidden
*/
public int getValue()
{
return value;
}
private int value;
/**
* Create a new conformance for a specific PDF version.
*/
public Conformance(Version version)
{
switch (version.getMajor())
{
case 1:
switch (version.getMinor())
{
case 0: value = COMPLIANCE.ePDF10; break;
case 1: value = COMPLIANCE.ePDF11; break;
case 2: value = COMPLIANCE.ePDF12; break;
case 3: value = COMPLIANCE.ePDF13; break;
case 4: value = COMPLIANCE.ePDF14; break;
case 5: value = COMPLIANCE.ePDF15; break;
case 6: value = COMPLIANCE.ePDF16; break;
case 7: value = COMPLIANCE.ePDF17; break;
default: throw new IllegalArgumentException(String.format("Invalid version %s.", version.toString()));
}
break;
case 2:
switch (version.getMinor())
{
case 0: value = COMPLIANCE.ePDF20; break;
default: throw new IllegalArgumentException(String.format("Invalid version %s.", version.toString()));
}
break;
default:
throw new IllegalArgumentException(String.format("Invalid version %s.", version.toString()));
}
}
/**
* Create a new PDF/A conformance.
*/
public Conformance(PdfAVersion pdfa)
{
switch (pdfa.getPart())
{
case 1:
switch (pdfa.getLevel())
{
case B: value = COMPLIANCE.ePDFA1b; break;
case A: value = COMPLIANCE.ePDFA1a; break;
default: throw new IllegalArgumentException(String.format("Invalid PDF/A-1 level %s.", pdfa.getLevel().toString()));
}
break;
case 2:
switch (pdfa.getLevel())
{
case B: value = COMPLIANCE.ePDFA2b; break;
case U: value = COMPLIANCE.ePDFA2u; break;
case A: value = COMPLIANCE.ePDFA2a; break;
default: throw new IllegalArgumentException(String.format("Invalid PDF/A-2 level %s.", pdfa.getLevel().toString()));
}
break;
case 3:
switch (pdfa.getLevel())
{
case B: value = COMPLIANCE.ePDFA3b; break;
case U: value = COMPLIANCE.ePDFA3u; break;
case A: value = COMPLIANCE.ePDFA3a; break;
default: throw new IllegalArgumentException(String.format("Invalid PDF/A-3 level %s.", pdfa.getLevel().toString()));
}
break;
default:
throw new IllegalArgumentException(String.format("Invalid PDF/A part %d.", pdfa.getPart()));
}
}
/**
* The PDF version of this conformance
*/
public Version getVersion()
{
switch (value)
{
case COMPLIANCE.ePDF10: return new Version(1,0);
case COMPLIANCE.ePDF11: return new Version(1,1);
case COMPLIANCE.ePDF12: return new Version(1,2);
case COMPLIANCE.ePDF13: return new Version(1,3);
case COMPLIANCE.ePDFA1b:
case COMPLIANCE.ePDFA1a:
case COMPLIANCE.ePDF14: return new Version(1,4);
case COMPLIANCE.ePDF15: return new Version(1,5);
case COMPLIANCE.ePDF16: return new Version(1,6);
case COMPLIANCE.ePDFA2b:
case COMPLIANCE.ePDFA2u:
case COMPLIANCE.ePDFA2a:
case COMPLIANCE.ePDFA3b:
case COMPLIANCE.ePDFA3u:
case COMPLIANCE.ePDFA3a:
case COMPLIANCE.ePDF17: return new Version(1,7);
case COMPLIANCE.ePDF20: return new Version(2,0);
}
throw new IllegalArgumentException(String.format("Invalid conformance %d.", value));
}
/**
* The the PDF/A version attributes of this conformance
*
* @return null
for non PDF/A conformances. The PDF/A version otherwise.
*/
public PdfAVersion getPdfA()
{
switch (value)
{
case COMPLIANCE.ePDFA1b: return new PdfAVersion(1, PdfAVersion.Level.B);
case COMPLIANCE.ePDFA1a: return new PdfAVersion(1, PdfAVersion.Level.A);
case COMPLIANCE.ePDFA2b: return new PdfAVersion(2, PdfAVersion.Level.B);
case COMPLIANCE.ePDFA2u: return new PdfAVersion(2, PdfAVersion.Level.U);
case COMPLIANCE.ePDFA2a: return new PdfAVersion(2, PdfAVersion.Level.A);
case COMPLIANCE.ePDFA3b: return new PdfAVersion(3, PdfAVersion.Level.B);
case COMPLIANCE.ePDFA3u: return new PdfAVersion(3, PdfAVersion.Level.U);
case COMPLIANCE.ePDFA3a: return new PdfAVersion(3, PdfAVersion.Level.A);
}
return null;
}
/**
* Check if this conformance conforms to another.
*/
public boolean conformsTo(Conformance other)
{
PdfAVersion a1 = getPdfA();
PdfAVersion a2 = other.getPdfA();
if (a1 != null)
{
if (a2 != null)
return a1.part == a2.part &&
a1.level.getValue() >= a2.level.getValue();
else
return getVersion().equals(other.getVersion());
}
else if (a2 != null)
return false;
else
return getVersion().equals(other.getVersion());
}
private static Map toString;
private static Map conformances;
static
{
Map toStringMap = new ConcurrentHashMap()
{{
put(COMPLIANCE.ePDFA2a, "PDF/A-2a");
put(COMPLIANCE.ePDFA2b, "PDF/A-2b");
put(COMPLIANCE.ePDFA2u, "PDF/A-2u");
put(COMPLIANCE.ePDFA3a, "PDF/A-3a");
put(COMPLIANCE.ePDFA3b, "PDF/A-3b");
put(COMPLIANCE.ePDFA3u, "PDF/A-3u");
put(COMPLIANCE.ePDFA1a, "PDF/A-1a");
put(COMPLIANCE.ePDFA1b, "PDF/A-1b");
put(COMPLIANCE.ePDF14, "PDF 1.4");
put(COMPLIANCE.ePDF15, "PDF 1.5");
put(COMPLIANCE.ePDF16, "PDF 1.6");
put(COMPLIANCE.ePDF17, "PDF 1.7");
put(COMPLIANCE.ePDF20, "PDF 2.0");
put(COMPLIANCE.ePDF10, "PDF 1.0");
put(COMPLIANCE.ePDF11, "PDF 1.1");
put(COMPLIANCE.ePDF12, "PDF 1.2");
put(COMPLIANCE.ePDF13, "PDF 1.3");
}};
toString = Collections.unmodifiableMap(toStringMap);
Map conformancesMap = new ConcurrentHashMap();
for (Entry entry : toString.entrySet())
{
conformancesMap.put(entry.getValue(), entry.getKey());
}
conformances = Collections.unmodifiableMap(conformancesMap);
}
@Override
public String toString()
{
String result = toString.get(value);
if (result == null)
throw new IllegalArgumentException(String.format("Invalid conformance %d.", value));
return result;
}
public static Conformance parse(String conformance)
{
Integer result = conformances.get(conformance);
if (result == null)
throw new IllegalArgumentException(String.format("Invalid conformance %s.", conformance));
return new Conformance(result);
}
@Override
public boolean equals(Object other)
{
if (other != null && other instanceof Conformance)
{
return ((Conformance) other).value == value;
}
return false;
}
@Override
public int hashCode()
{
return value;
}
}