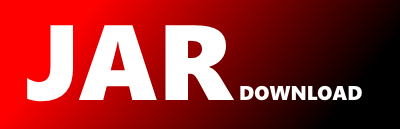
com.pdftools.pdf.Document Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
/****************************************************************************
*
* File: Document.java
*
* Description: PDFTOOLS Document Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.pdf;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The PDF document
* PDF documents are either opened using {@link Document#open } or the result of an operation, e.g. of PDF optimization (see {@link com.pdftools.optimization.Optimizer#optimizeDocument pdftools.optimization.Optimizer.optimizeDocument}).
*/
public class Document extends NativeObject implements AutoCloseable
{
protected Document(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Document createDynamicObject(long handle)
{
int type = getType(handle);
switch (type)
{
case 0:
return new Document(handle);
case 1:
return com.pdftools.sign.PreparedDocument.createDynamicObject(handle);
default:
return null;
}
}
/**
* Open a PDF document.
* Documents opened with this method are read-only and cannot be modified.
* @param stream
* The stream from which the PDF is read.
* @return
* The newly created document instance
*
* @throws com.pdftools.LicenseException The license check has failed.
* @throws com.pdftools.PasswordException The document is encrypted and the {@code password} is invalid.
* @throws com.pdftools.CorruptException The document is corrupt or not a PDF.
* @throws com.pdftools.UnsupportedFeatureException The document is an unencrypted wrapper document.
* @throws com.pdftools.GenericException A generic error occurred.
* @throws IllegalArgumentException if {@code stream} is {@code null}
*/
public static com.pdftools.pdf.Document open(com.pdftools.sys.Stream stream)
throws
com.pdftools.GenericException,
com.pdftools.LicenseException,
com.pdftools.CorruptException,
com.pdftools.PasswordException,
com.pdftools.UnsupportedFeatureException
{
if (stream == null)
throw new IllegalArgumentException("Argument 'stream' must not be null.", new NullPointerException("'stream'"));
return open(stream, null);
}
/**
* Open a PDF document.
* Documents opened with this method are read-only and cannot be modified.
* @param stream
* The stream from which the PDF is read.
* @param password
* The password to open the PDF document.
* If {@code null} or empty, no password is used.
* @return
* The newly created document instance
*
* @throws com.pdftools.LicenseException The license check has failed.
* @throws com.pdftools.PasswordException The document is encrypted and the {@code password} is invalid.
* @throws com.pdftools.CorruptException The document is corrupt or not a PDF.
* @throws com.pdftools.UnsupportedFeatureException The document is an unencrypted wrapper document.
* @throws com.pdftools.GenericException A generic error occurred.
* @throws IllegalArgumentException if {@code stream} is {@code null}
*/
public static com.pdftools.pdf.Document open(com.pdftools.sys.Stream stream, String password)
throws
com.pdftools.GenericException,
com.pdftools.LicenseException,
com.pdftools.CorruptException,
com.pdftools.PasswordException,
com.pdftools.UnsupportedFeatureException
{
if (stream == null)
throw new IllegalArgumentException("Argument 'stream' must not be null.", new NullPointerException("'stream'"));
long retHandle = openNative(stream, password);
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 10: throw new com.pdftools.GenericException(getLastErrorMessage());
case 12: throw new com.pdftools.LicenseException(getLastErrorMessage());
case 16: throw new com.pdftools.CorruptException(getLastErrorMessage());
case 17: throw new com.pdftools.PasswordException(getLastErrorMessage());
case 19: throw new com.pdftools.UnsupportedFeatureException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return com.pdftools.pdf.Document.createDynamicObject(retHandle);
}
/**
* The claimed conformance of the document (Getter)
*
* This method only returns the claimed conformance level,
* the document is not validated.
*
* This property can return {@code null} if the document's conformance is unknown.
*
*/
public com.pdftools.pdf.Conformance getConformance()
{
Integer retVal = getConformanceNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
return null;
}
return new com.pdftools.pdf.Conformance(retVal);
}
/**
* The number of pages in the document (Getter)
* If the document is a collection (also known as PDF Portfolio), then this property is {@code 0}.
*/
public int getPageCount()
{
int retVal = getPageCountNative(getHandle());
if (retVal == -1)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The access permissions applicable for this document (Getter)
*
* This property is {@code null}, if the document is not encrypted.
*
* Note that these permissions might be different from the "Document Restrictions Summary" displayed in Adobe Acrobat.
* This is because Acrobat's restrictions are also affected by other factors.
* For example, "Document Assembly" is generally only allowed in Acrobat Pro and not the Acrobat Reader.
*
*/
public EnumSet getPermissions()
{
Integer retVal = getPermissionsNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
return null;
}
return getEnumSet(retVal, com.pdftools.pdf.Permission.class);
}
/**
* Whether the document is linearized (Getter)
*
*/
public boolean getIsLinearized()
{
boolean retVal = getIsLinearizedNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
*
*/
public boolean getIsSigned()
{
boolean retVal = getIsSignedNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
*
*/
public com.pdftools.pdf.SignatureFieldList getSignatureFields()
{
long retHandle = getSignatureFieldsNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.pdf.SignatureFieldList.createDynamicObject(retHandle);
}
/**
* Whether the document is an XML Forms Architecture (XFA) or a PDF document (Getter)
* While XFA documents may seem like regular PDF documents they are not and cannot be processed by many components (error {@link com.pdftools.UnsupportedFeatureException }).
* An XFA form is included as a resource in a mere shell PDF.
* The PDF pages' content is generated dynamically from the XFA data, which is a complex, non-standardized process.
* For this reason, XFA is forbidden by the ISO Standards ISO 19'005-2 (PDF/A-2) and ISO 32'000-2 (PDF 2.0) and newer.
* It is recommended to convert XFA documents to PDF using an Adobe product, e.g. by using the "Print to PDF" function of Adobe Acrobat Reader.
*/
public com.pdftools.pdf.XfaType getXfa()
{
int retVal = getXfaNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return com.pdftools.pdf.XfaType.fromValue(retVal);
}
/**
* The metadata of the document. (Getter)
*
*/
public com.pdftools.pdf.Metadata getMetadata()
{
long retHandle = getMetadataNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.pdf.Metadata.createDynamicObject(retHandle);
}
private static native int getType(long handle);
private static native long openNative(com.pdftools.sys.Stream stream, String password);
private native Integer getConformanceNative(long handle);
private native int getPageCountNative(long handle);
private native Integer getPermissionsNative(long handle);
private native boolean getIsLinearizedNative(long handle);
private native boolean getIsSignedNative(long handle);
private native long getSignatureFieldsNative(long handle);
private native int getXfaNative(long handle);
private native long getMetadataNative(long handle);
/**
* Close the object.
* Release all resources associated with the object.
* @throws com.pdftools.PdfToolsException only explicitly stated in a superclass
*/
public void close()
throws com.pdftools.PdfToolsException,
java.io.IOException
{
try
{
if (!close(getHandle()))
throwLastError();
}
finally
{
setHandle(0);
}
}
private native boolean close(long hObject);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy