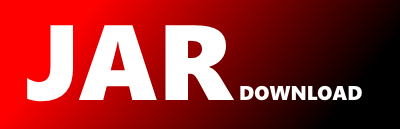
com.pdftools.pdf.Encryption Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: Encryption.java
*
* Description: PDFTOOLS Encryption Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.pdf;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The parameters to encrypt PDF documents
*
* PDF document can be encrypted to protect content from unauthorized access.
* The encryption process applies encryption to all streams (e.g. images) and strings, but not to other items in the PDF document.
* This means the structure of the PDF document is accessible, but the content of its pages is encrypted.
*
* The standard security handler allows access permissions and up to two passwords to be specified for a document:
* A user password (see {@link Encryption#getUserPassword }) and an owner password (see {@link Encryption#getOwnerPassword }).
*
* The following list shows the four possible combinations of passwords and how an application processing such a PDF document behaves:
*
*
* -
*
* No user password, no owner password (no encryption):
*
* Everyone can read, i.e. no password required to open the document.
* Everyone can change security settings.
*
* -
*
* No user password, owner password:
*
* Everyone can read, i.e. no password required to open the document.
* Access permissions are restricted (unless the owner password is provided).
* Owner password required to change security settings.
*
* -
*
* User password, no owner password:
*
* User password required to read.
* All access permissions are granted.
*
* -
*
* User password, owner password:
*
* User or owner password required to read.
* Access permissions are restricted (unless the owner password is provided).
* Owner password required to change security settings.
*
*
*
* Since encryption is not allowed by the PDF/A ISO standards, PDF/A documents must not be encrypted.
*
*/
public class Encryption extends NativeObject
{
protected Encryption(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Encryption createDynamicObject(long handle)
{
return new Encryption(handle);
}
/**
*
* @param userPassword
* Set the user password of the output document (see {@link Encryption#getUserPassword }).
* If {@code null} or empty, no user password is set.
* @param ownerPassword
* Set the owner password and permissions of the output document (see {@link Encryption#getOwnerPassword }).
* If {@code null} or empty, no owner password is set.
* @param permissions
* The permissions to be set on the PDF document.
* If no owner password is set, the permissions must not be restricted, i.e. the {@code permissions} must be {@code All}.
* @throws IllegalArgumentException if {@code permissions} is {@code null}
*/
public Encryption(String userPassword, String ownerPassword, EnumSet permissions)
{
this(newHelper(userPassword, ownerPassword, permissions));
}
private static long newHelper(String userPassword, String ownerPassword, EnumSet permissions)
{
if (permissions == null)
throw new IllegalArgumentException("Argument 'permissions' must not be null.", new NullPointerException("'permissions'"));
long handle = newNative(userPassword, ownerPassword, getFlags(permissions));
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* Set the owner password and access permissions.
* Only the given permissions are granted when opening the document.
* To the owner of the document, all permissions are granted.
* For this, the document must be opened by specifying the owner password (see {@link Encryption#getOwnerPassword }).
* @param ownerPassword
* The owner password to be set on the PDF document (see {@link Encryption#getOwnerPassword }).
* @param permissions
* The permissions to be set on the PDF document.
*
* @throws IllegalArgumentException If restricted {@link com.pdftools.pdf.Encryption#setPermissions permissions} (i.e. not {@code All}) are specified without {@link com.pdftools.pdf.Encryption#setPermissions ownerPassword}.
* @throws IllegalArgumentException if {@code ownerPassword} is {@code null}
* @throws IllegalArgumentException if {@code permissions} is {@code null}
*/
public void setPermissions(String ownerPassword, EnumSet permissions)
{
if (ownerPassword == null)
throw new IllegalArgumentException("Argument 'ownerPassword' must not be null.", new NullPointerException("'ownerPassword'"));
if (permissions == null)
throw new IllegalArgumentException("Argument 'permissions' must not be null.", new NullPointerException("'permissions'"));
boolean retVal = setPermissionsNative(getHandle(), ownerPassword, getFlags(permissions));
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The user password (Getter)
*
* This password protects the document against unauthorized opening and reading.
*
* If a PDF document is protected by a user password, it cannot be opened without a password.
* The user or, if set, owner password must be provided to open and read the document.
*
* If a document is not protected by a user password, it can be opened by without a password, even if an owner password is set.
*
* If the password contains characters that are not in the Windows ANSI encoding (Windows Code Page 1252),
* the output document's compliance level is automatically upgraded to PDF version 1.7.
* This is because older PDF versions do not support Unicode passwords.
*
*/
public String getUserPassword()
{
String retVal = getUserPasswordNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The user password (Setter)
*
* This password protects the document against unauthorized opening and reading.
*
* If a PDF document is protected by a user password, it cannot be opened without a password.
* The user or, if set, owner password must be provided to open and read the document.
*
* If a document is not protected by a user password, it can be opened by without a password, even if an owner password is set.
*
* If the password contains characters that are not in the Windows ANSI encoding (Windows Code Page 1252),
* the output document's compliance level is automatically upgraded to PDF version 1.7.
* This is because older PDF versions do not support Unicode passwords.
*
*/
public void setUserPassword(String value)
{
boolean retVal = setUserPasswordNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* The owner password (Getter)
*
* This password is sometimes also referred to as the author’s password.
* This password grants full access to the document.
* Not only can the document be opened and read, it also allows the document's security settings, access permissions, and passwords to be changed.
*
* If the password contains characters that are not in the Windows ANSI encoding (Windows Code Page 1252),
* the output document's compliance level is automatically upgraded to PDF version 1.7.
* This is because older PDF versions do not support Unicode passwords.
*
*/
public String getOwnerPassword()
{
String retVal = getOwnerPasswordNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The access permissions granted when opening the document (Getter)
*
* The operations granted in a PDF document are controlled using permission flags.
* In order to set permission flags, the PDF document must be encrypted and have an owner password.
*
* The restricted permissions apply whenever the document is opened with a password other than the owner password.
* The owner password is required to initially set or later change the permission flags.
*
* When opening an encrypted document, the access permissions for the document can be read using {@link Document#getPermissions }.
* Note that the permissions might be different from the "Document Restrictions Summary" displayed in Adobe Acrobat.
*
*/
public EnumSet getPermissions()
{
int retVal = getPermissionsNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return getEnumSet(retVal, com.pdftools.pdf.Permission.class);
}
private static native long newNative(String userPassword, String ownerPassword, int permissions);
private native boolean setPermissionsNative(long handle, String ownerPassword, int permissions);
private native String getUserPasswordNative(long handle);
private native boolean setUserPasswordNative(long handle, String value);
private native String getOwnerPasswordNative(long handle);
private native int getPermissionsNative(long handle);
}