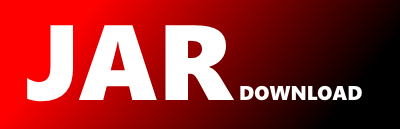
com.pdftools.pdf.MetadataSettings Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: MetadataSettings.java
*
* Description: PDFTOOLS MetadataSettings Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.pdf;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
*
* It allows you to set and update individual metadata properties.
* Any metadata properties that have been explicitly set are included in the output document.
*/
public class MetadataSettings extends NativeObject
{
protected MetadataSettings(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static MetadataSettings createDynamicObject(long handle)
{
return new MetadataSettings(handle);
}
/**
*
*/
public MetadataSettings()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* The title of the document or resource. (Getter)
* This property corresponds to the "dc:title" entry
* in the XMP metadata and to the "Title" entry in
* the document information dictionary.
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public String getTitle()
{
String retVal = getTitleNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The title of the document or resource. (Setter)
* This property corresponds to the "dc:title" entry
* in the XMP metadata and to the "Title" entry in
* the document information dictionary.
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public void setTitle(String value)
{
boolean retVal = setTitleNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The name of the person who created the document or resource. (Getter)
* This property corresponds to the "dc:creator" entry
* in the XMP metadata and to the "Author" entry in
* the document information dictionary.
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public String getAuthor()
{
String retVal = getAuthorNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The name of the person who created the document or resource. (Setter)
* This property corresponds to the "dc:creator" entry
* in the XMP metadata and to the "Author" entry in
* the document information dictionary.
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public void setAuthor(String value)
{
boolean retVal = setAuthorNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The subject of the document or resource. (Getter)
* This property corresponds to the "dc:description" entry
* in the XMP metadata and to the "Subject" entry in
* the document information dictionary.
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public String getSubject()
{
String retVal = getSubjectNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The subject of the document or resource. (Setter)
* This property corresponds to the "dc:description" entry
* in the XMP metadata and to the "Subject" entry in
* the document information dictionary.
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public void setSubject(String value)
{
boolean retVal = setSubjectNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* Keywords associated with the document or resource. (Getter)
*
* Keywords can be separated by:
*
* -
* carriage return / line feed
* -
* comma
* -
* semicolon
* -
* tab
* -
* double space
*
*
* This property corresponds to the "pdf:Keywords" entry
* in the XMP metadata and to the "Keywords" entry in
* the document information dictionary.
*
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public String getKeywords()
{
String retVal = getKeywordsNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Keywords associated with the document or resource. (Setter)
*
* Keywords can be separated by:
*
* -
* carriage return / line feed
* -
* comma
* -
* semicolon
* -
* tab
* -
* double space
*
*
* This property corresponds to the "pdf:Keywords" entry
* in the XMP metadata and to the "Keywords" entry in
* the document information dictionary.
*
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public void setKeywords(String value)
{
boolean retVal = setKeywordsNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The original application that created the document. (Getter)
*
* The name of the first known tool used to create the document or resource.
*
* This property corresponds to the "xmp:CreatorTool" entry
* in the XMP metadata and to the "Creator" entry in
* the document information dictionary.
*
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public String getCreator()
{
String retVal = getCreatorNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The original application that created the document. (Setter)
*
* The name of the first known tool used to create the document or resource.
*
* This property corresponds to the "xmp:CreatorTool" entry
* in the XMP metadata and to the "Creator" entry in
* the document information dictionary.
*
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public void setCreator(String value)
{
boolean retVal = setCreatorNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The application that created the PDF (Getter)
*
* If the document has been converted to PDF from another format,
* the name of the PDF processor that converted the document to PDF.
*
* This property corresponds to the "pdf:Producer" entry
* in the XMP metadata and to the "Producer" entry in
* the document information dictionary.
*
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public String getProducer()
{
String retVal = getProducerNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The application that created the PDF (Setter)
*
* If the document has been converted to PDF from another format,
* the name of the PDF processor that converted the document to PDF.
*
* This property corresponds to the "pdf:Producer" entry
* in the XMP metadata and to the "Producer" entry in
* the document information dictionary.
*
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public void setProducer(String value)
{
boolean retVal = setProducerNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The date and time the document or resource was originally created. (Getter)
* This property corresponds to the "xmp:CreateDate" entry
* in the XMP metadata and to the "CreationDate" entry in
* the document information dictionary.
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public OffsetDateTime getCreationDate()
{
OffsetDateTime retVal = getCreationDateNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The date and time the document or resource was originally created. (Setter)
* This property corresponds to the "xmp:CreateDate" entry
* in the XMP metadata and to the "CreationDate" entry in
* the document information dictionary.
*
* @throws IllegalStateException If the metadata settings have already been closed
* @throws IllegalArgumentException The date is invalid.
*/
public void setCreationDate(OffsetDateTime value)
{
boolean retVal = setCreationDateNative(getHandle(), value == null, value != null ? value.getYear() : 0, value != null ? value.getMonthValue() : 0, value != null ? value.getDayOfMonth() : 0, value != null ? value.getHour() : 0, value != null ? value.getMinute() : 0, value != null ? value.getSecond() : 0, value != null ? value.getOffset().getTotalSeconds() : 0);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The date and time the document or resource was most recently modified. (Getter)
* This property corresponds to the "xmp:ModifyDate" entry
* in the XMP metadata and to the "ModDate" entry in
* the document information dictionary.
*
* @throws IllegalStateException If the metadata settings have already been closed
*/
public OffsetDateTime getModificationDate()
{
OffsetDateTime retVal = getModificationDateNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The date and time the document or resource was most recently modified. (Setter)
* This property corresponds to the "xmp:ModifyDate" entry
* in the XMP metadata and to the "ModDate" entry in
* the document information dictionary.
*
* @throws IllegalStateException If the metadata settings have already been closed
* @throws IllegalArgumentException The date is invalid.
*/
public void setModificationDate(OffsetDateTime value)
{
boolean retVal = setModificationDateNative(getHandle(), value == null, value != null ? value.getYear() : 0, value != null ? value.getMonthValue() : 0, value != null ? value.getDayOfMonth() : 0, value != null ? value.getHour() : 0, value != null ? value.getMinute() : 0, value != null ? value.getSecond() : 0, value != null ? value.getOffset().getTotalSeconds() : 0);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
private static native long newNative();
private native String getTitleNative(long handle);
private native boolean setTitleNative(long handle, String value);
private native String getAuthorNative(long handle);
private native boolean setAuthorNative(long handle, String value);
private native String getSubjectNative(long handle);
private native boolean setSubjectNative(long handle, String value);
private native String getKeywordsNative(long handle);
private native boolean setKeywordsNative(long handle, String value);
private native String getCreatorNative(long handle);
private native boolean setCreatorNative(long handle, String value);
private native String getProducerNative(long handle);
private native boolean setProducerNative(long handle, String value);
private native OffsetDateTime getCreationDateNative(long handle);
private native boolean setCreationDateNative(long handle, boolean isNull, int year, int month, int day, int hour, int minute, int second, int tzOffset);
private native OffsetDateTime getModificationDateNative(long handle);
private native boolean setModificationDateNative(long handle, boolean isNull, int year, int month, int day, int hour, int minute, int second, int tzOffset);
}