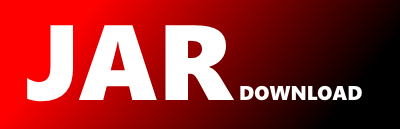
com.pdftools.pdf.OutputOptions Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: OutputOptions.java
*
* Description: PDFTOOLS OutputOptions Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.pdf;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The parameters for document-level features of output PDFs
* Output options are used in many operations that create PDF documents.
*/
public class OutputOptions extends NativeObject
{
protected OutputOptions(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static OutputOptions createDynamicObject(long handle)
{
int type = getType(handle);
switch (type)
{
case 0:
return new OutputOptions(handle);
case 1:
return com.pdftools.sign.OutputOptions.createDynamicObject(handle);
default:
return null;
}
}
/**
*
*/
public OutputOptions()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* The parameters to encrypt output PDFs (Getter)
*
* If {@code null}, no encryption is used.
*
* Encryption is not allowed by the PDF/A ISO standards.
* For that reason, it is recommended to use {@code null} when processing PDF/A documents.
* Otherwise, most operations will remove PDF/A conformance from the output document.
* More details can be found in the documentation of the operation.
*
* Default: {@code null}, no encryption is used.
*
*/
public com.pdftools.pdf.Encryption getEncryption()
{
long retHandle = getEncryptionNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
return null;
}
return com.pdftools.pdf.Encryption.createDynamicObject(retHandle);
}
/**
* The parameters to encrypt output PDFs (Setter)
*
* If {@code null}, no encryption is used.
*
* Encryption is not allowed by the PDF/A ISO standards.
* For that reason, it is recommended to use {@code null} when processing PDF/A documents.
* Otherwise, most operations will remove PDF/A conformance from the output document.
* More details can be found in the documentation of the operation.
*
* Default: {@code null}, no encryption is used.
*
*/
public void setEncryption(com.pdftools.pdf.Encryption value)
{
boolean retVal = setEncryptionNative(getHandle(), getHandle(value), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Default: {@code null}, metadata are copied to the output document.
*/
public com.pdftools.pdf.MetadataSettings getMetadataSettings()
{
long retHandle = getMetadataSettingsNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
return null;
}
return com.pdftools.pdf.MetadataSettings.createDynamicObject(retHandle);
}
/**
* Default: {@code null}, metadata are copied to the output document.
*/
public void setMetadataSettings(com.pdftools.pdf.MetadataSettings value)
{
boolean retVal = setMetadataSettingsNative(getHandle(), getHandle(value), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
private static native int getType(long handle);
private static native long newNative();
private native long getEncryptionNative(long handle);
private native boolean setEncryptionNative(long handle, long value, com.pdftools.pdf.Encryption valueObj);
private native long getMetadataSettingsNative(long handle);
private native boolean setMetadataSettingsNative(long handle, long value, com.pdftools.pdf.MetadataSettings valueObj);
}