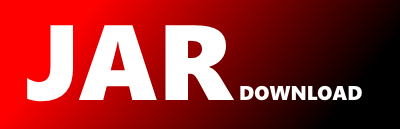
com.pdftools.pdf.Revision Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
/****************************************************************************
*
* File: Revision.java
*
* Description: PDFTOOLS Revision Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.pdf;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The document revision
* An incremental update to a PDF creates a new revision.
* A revision is defined by the update itself and all updates that came before, including the initial document.
* An update can introduce changes to the document (visible or invisible), it can sign the current revision, or it can do both.
* But an update can only ever hold one valid signature.
*/
public class Revision extends NativeObject
{
protected Revision(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Revision createDynamicObject(long handle)
{
return new Revision(handle);
}
/**
* Write the contents of the document revision to a stream
* @param stream
* The stream to which the revision is written.
*
* @throws java.io.IOException Unable to write to the stream.
* @throws IllegalArgumentException if {@code stream} is {@code null}
*/
public void write(com.pdftools.sys.Stream stream)
throws
java.io.IOException
{
if (stream == null)
throw new IllegalArgumentException("Argument 'stream' must not be null.", new NullPointerException("'stream'"));
boolean retVal = writeNative(getHandle(), stream);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 4: throw new java.io.IOException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* Whether this is the latest document revision (Getter)
*
*/
public boolean getIsLatest()
{
boolean retVal = getIsLatestNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether the revision contains a non-signing update. (Getter)
* Returns {@code true} if any update leading up to this revision does not contain a signature.
* Returns {@code false} if every update that leads up to this revision contains a signature.
*/
public boolean getHasNonSigningUpdates()
{
boolean retVal = getHasNonSigningUpdatesNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
private native boolean writeNative(long handle, com.pdftools.sys.Stream stream);
private native boolean getIsLatestNative(long handle);
private native boolean getHasNonSigningUpdatesNative(long handle);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy