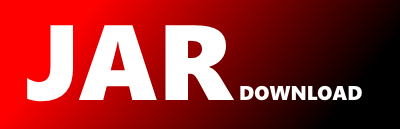
com.pdftools.pdf2image.Converter Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: Converter.java
*
* Description: PDFTOOLS Converter Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.pdf2image;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The class to convert a PDF document to a rasterized image
*/
public class Converter extends NativeObject
{
protected Converter(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Converter createDynamicObject(long handle)
{
return new Converter(handle);
}
/**
*
*/
public Converter()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* Convert all pages of a PDF document to a rasterized image
* @param inDoc
* The input PDF document
* @param outStream
* The stream to which the rasterized image is written.
* @param profile
*
* The profile defines how the PDF pages are rendered and what type of output image is used.
* Note that the profile's image options must support multi-page images (TIFF).
* For other profiles, the method {@link Converter#convertPage } should be used.
*
* For details, see {@link com.pdftools.pdf2image.profiles.Profile profiles.Profile}.
*
* @return
*
* The output image document.
* The object can be used as input for further processing.
*
* Note that, this object must be disposed before the output stream
* object (method argument {@link com.pdftools.pdf2image.Converter#convertDocument outStream}).
*
*
* @throws com.pdftools.LicenseException The license is invalid.
* @throws java.io.IOException Writing to the output image failed.
* @throws com.pdftools.UnsupportedFeatureException The input PDF is a PDF collection (Portfolio) that has no cover pages.
* @throws com.pdftools.UnsupportedFeatureException The input PDF contains unrendered XFA form fields.
* See {@link com.pdftools.pdf.Document#getXfa pdftools.pdf.Document.getXfa} for more information on how to detect and handle XFA documents.
* @throws com.pdftools.GenericException An unexpected failure occurred.
* @throws IllegalArgumentException The {@link com.pdftools.pdf2image.Converter#convertDocument profile} does not support multi-page output.
* @throws com.pdftools.ProcessingException The processing has failed.
* @throws IllegalStateException Internal error has occured.
* @throws IllegalArgumentException if {@code inDoc} is {@code null}
* @throws IllegalArgumentException if {@code outStream} is {@code null}
* @throws IllegalArgumentException if {@code profile} is {@code null}
*/
public com.pdftools.image.MultiPageDocument convertDocument(com.pdftools.pdf.Document inDoc, com.pdftools.sys.Stream outStream, com.pdftools.pdf2image.profiles.Profile profile)
throws
java.io.IOException,
com.pdftools.GenericException,
com.pdftools.LicenseException,
com.pdftools.UnsupportedFeatureException,
com.pdftools.ProcessingException
{
if (inDoc == null)
throw new IllegalArgumentException("Argument 'inDoc' must not be null.", new NullPointerException("'inDoc'"));
if (outStream == null)
throw new IllegalArgumentException("Argument 'outStream' must not be null.", new NullPointerException("'outStream'"));
if (profile == null)
throw new IllegalArgumentException("Argument 'profile' must not be null.", new NullPointerException("'profile'"));
long retHandle = convertDocumentNative(getHandle(), getHandle(inDoc), inDoc, outStream, getHandle(profile), profile);
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
case 3: throw new IllegalArgumentException(getLastErrorMessage());
case 4: throw new java.io.IOException(getLastErrorMessage());
case 10: throw new com.pdftools.GenericException(getLastErrorMessage());
case 12: throw new com.pdftools.LicenseException(getLastErrorMessage());
case 19: throw new com.pdftools.UnsupportedFeatureException(getLastErrorMessage());
case 21: throw new com.pdftools.ProcessingException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return com.pdftools.image.MultiPageDocument.createDynamicObject(retHandle);
}
/**
* Convert a single page of a PDF document to a rasterized image
* @param inDoc
* The input PDF document
* @param outStream
* The stream to which the rasterized image is written.
* @param profile
*
* The profile defines how the PDF page is rendered and what type of output image is used.
*
* For details, see {@link com.pdftools.pdf2image.profiles.Profile profiles.Profile}.
*
* @param pageNumber
* The PDF page number to be converted.
* The number must be in the range of {@code 1} (first page) to {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount} (last page).
* @return
*
* The image object allowing to open and read the
* output image and treat it as a new input for further processing.
*
* Note that, this object must be disposed before the output stream
* object (method argument {@link com.pdftools.pdf2image.Converter#convertPage outStream}).
*
*
* @throws com.pdftools.LicenseException The license is invalid.
* @throws IllegalArgumentException The {@link com.pdftools.pdf2image.Converter#convertPage pageNumber} is not in the allowed range.
* @throws java.io.IOException Writing to the output image failed.
* @throws com.pdftools.UnsupportedFeatureException The input PDF is a collection that has no cover pages.
* @throws com.pdftools.UnsupportedFeatureException The input PDF contains unrendered XFA form fields.
* See {@link com.pdftools.pdf.Document#getXfa pdftools.pdf.Document.getXfa} for more information on how to detect and handle XFA documents.
* @throws com.pdftools.GenericException An unexpected failure occurred.
* @throws com.pdftools.ProcessingException The processing has failed.
* @throws IllegalArgumentException if {@code inDoc} is {@code null}
* @throws IllegalArgumentException if {@code outStream} is {@code null}
* @throws IllegalArgumentException if {@code profile} is {@code null}
*/
public com.pdftools.image.Document convertPage(com.pdftools.pdf.Document inDoc, com.pdftools.sys.Stream outStream, com.pdftools.pdf2image.profiles.Profile profile, int pageNumber)
throws
java.io.IOException,
com.pdftools.GenericException,
com.pdftools.LicenseException,
com.pdftools.UnsupportedFeatureException,
com.pdftools.ProcessingException
{
if (inDoc == null)
throw new IllegalArgumentException("Argument 'inDoc' must not be null.", new NullPointerException("'inDoc'"));
if (outStream == null)
throw new IllegalArgumentException("Argument 'outStream' must not be null.", new NullPointerException("'outStream'"));
if (profile == null)
throw new IllegalArgumentException("Argument 'profile' must not be null.", new NullPointerException("'profile'"));
long retHandle = convertPageNative(getHandle(), getHandle(inDoc), inDoc, outStream, getHandle(profile), profile, pageNumber);
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
case 4: throw new java.io.IOException(getLastErrorMessage());
case 10: throw new com.pdftools.GenericException(getLastErrorMessage());
case 12: throw new com.pdftools.LicenseException(getLastErrorMessage());
case 19: throw new com.pdftools.UnsupportedFeatureException(getLastErrorMessage());
case 21: throw new com.pdftools.ProcessingException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return com.pdftools.image.Document.createDynamicObject(retHandle);
}
private static native long newNative();
private native long convertDocumentNative(long handle, long inDoc, com.pdftools.pdf.Document inDocObj, com.pdftools.sys.Stream outStream, long profile, com.pdftools.pdf2image.profiles.Profile profileObj);
private native long convertPageNative(long handle, long inDoc, com.pdftools.pdf.Document inDocObj, com.pdftools.sys.Stream outStream, long profile, com.pdftools.pdf2image.profiles.Profile profileObj, int pageNumber);
}