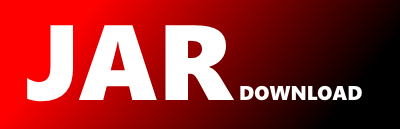
com.pdftools.pdf2image.RenderPageAtResolution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
The newest version!
/****************************************************************************
*
* File: RenderPageAtResolution.java
*
* Description: PDFTOOLS RenderPageAtResolution Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.pdf2image;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The image section mapping to render entire pages at a specific resolution
*
* The entire PDF page is rendered into an image of the same size and the specified resolution.
*
* For example, this mapping is suitable to create images of entire PDF pages.
*
*/
public class RenderPageAtResolution extends com.pdftools.pdf2image.ImageSectionMapping
{
protected RenderPageAtResolution(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static RenderPageAtResolution createDynamicObject(long handle)
{
return new RenderPageAtResolution(handle);
}
/**
*
* @param resolution
* The resolution of the output image.
*
* @throws IllegalArgumentException The resolution is smaller than 0.0 or greater than 10000.0.
* @throws IllegalArgumentException if {@code resolution} is {@code null}
*/
public RenderPageAtResolution(com.pdftools.geometry.units.Resolution resolution)
{
this(newHelper(resolution));
}
private static long newHelper(com.pdftools.geometry.units.Resolution resolution)
{
if (resolution == null)
throw new IllegalArgumentException("Argument 'resolution' must not be null.", new NullPointerException("'resolution'"));
long handle = newNative(resolution.getXDpi(), resolution.getYDpi());
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* The resolution of the output image (Getter)
* Valid values are 0.0, 10000.0 or in between.
*/
public com.pdftools.geometry.units.Resolution getResolution()
{
com.pdftools.geometry.units.Resolution retVal = getResolutionNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The resolution of the output image (Setter)
* Valid values are 0.0, 10000.0 or in between.
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setResolution(com.pdftools.geometry.units.Resolution value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setResolutionNative(getHandle(), value.getXDpi(), value.getYDpi());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
private static native long newNative(double resolutionXDpi, double resolutionYDpi);
private native com.pdftools.geometry.units.Resolution getResolutionNative(long handle);
private native boolean setResolutionNative(long handle, double valueXDpi, double valueYDpi);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy