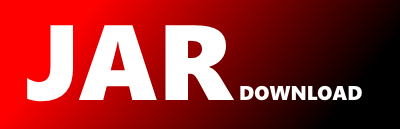
com.pdftools.pdfa.conversion.ConversionOptions Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: ConversionOptions.java
*
* Description: PDFTOOLS ConversionOptions Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.pdfa.conversion;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The PDF/A conversion options
* The options for the conversion of documents using the converter's method {@link Converter#convert }
*/
public class ConversionOptions extends NativeObject
{
protected ConversionOptions(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static ConversionOptions createDynamicObject(long handle)
{
return new ConversionOptions(handle);
}
/**
*
*/
public ConversionOptions()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* The minimal target conformance (Getter)
*
* If a conformance is set, it is used as the minimal target conformance.
* The PDF/A version of the conformance must match the PDF/A version of the analysisOptions of {@link com.pdftools.pdfa.validation.Validator#analyze pdftools.pdfa.validation.Validator.analyze}.
* If the conformance level cannot be achieved, the conversion will abort with the error {@link com.pdftools.ConformanceException }.
* If a higher conformance level can be achieved, it is used automatically.
*
* If {@code null} is used, the optimal conformance determined in the analysis
* (i.e. {@link com.pdftools.pdfa.validation.AnalysisResult#getRecommendedConformance pdftools.pdfa.validation.AnalysisResult.getRecommendedConformance}) is used.
* It is highly recommended to use {@code null}.
*
* Default value: {@code null}
*/
public com.pdftools.pdf.Conformance getConformance()
{
Integer retVal = getConformanceNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
return null;
}
return new com.pdftools.pdf.Conformance(retVal);
}
/**
* The minimal target conformance (Setter)
*
* If a conformance is set, it is used as the minimal target conformance.
* The PDF/A version of the conformance must match the PDF/A version of the analysisOptions of {@link com.pdftools.pdfa.validation.Validator#analyze pdftools.pdfa.validation.Validator.analyze}.
* If the conformance level cannot be achieved, the conversion will abort with the error {@link com.pdftools.ConformanceException }.
* If a higher conformance level can be achieved, it is used automatically.
*
* If {@code null} is used, the optimal conformance determined in the analysis
* (i.e. {@link com.pdftools.pdfa.validation.AnalysisResult#getRecommendedConformance pdftools.pdfa.validation.AnalysisResult.getRecommendedConformance}) is used.
* It is highly recommended to use {@code null}.
*
* Default value: {@code null}
*/
public void setConformance(com.pdftools.pdf.Conformance value)
{
boolean retVal = setConformanceNative(getHandle(), value == null ? 0 : value.getValue(), value == null);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Whether to copy metadata (Getter)
* Copy document information dictionary and XMP metadata.
* Default: {@code true}.
*/
public boolean getCopyMetadata()
{
boolean retVal = getCopyMetadataNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether to copy metadata (Setter)
* Copy document information dictionary and XMP metadata.
* Default: {@code true}.
*/
public void setCopyMetadata(boolean value)
{
boolean retVal = setCopyMetadataNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Image quality of recompressed images (Getter)
*
* The image quality for images that use a prohibited lossy compression type and must be recompressed.
* Supported values are {@code 0.01} to {@code 1.0}.
* A higher value means better visual quality at the cost of a larger file size.
* Recommended values range from {@code 0.7} to {@code 0.9}.
*
* Example:
* JPX (JPEG2000) is not allowed in PDF/A-1. If a PDF contains a JPX compressed image, its compression type must be altered.
* Thus the image is converted to an image with JPEG compression using the image quality defined by this property.
* Default value: {@code 0.8}
*/
public double getImageQuality()
{
double retVal = getImageQualityNative(getHandle());
if (retVal == -1.0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Image quality of recompressed images (Setter)
*
* The image quality for images that use a prohibited lossy compression type and must be recompressed.
* Supported values are {@code 0.01} to {@code 1.0}.
* A higher value means better visual quality at the cost of a larger file size.
* Recommended values range from {@code 0.7} to {@code 0.9}.
*
* Example:
* JPX (JPEG2000) is not allowed in PDF/A-1. If a PDF contains a JPX compressed image, its compression type must be altered.
* Thus the image is converted to an image with JPEG compression using the image quality defined by this property.
* Default value: {@code 0.8}
*
* @throws IllegalArgumentException The given value is smaller than {@code 0.1} or greater than {@code 1}.
*/
public void setImageQuality(double value)
{
boolean retVal = setImageQualityNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
private static native long newNative();
private native Integer getConformanceNative(long handle);
private native boolean setConformanceNative(long handle, int value, boolean isNull);
private native boolean getCopyMetadataNative(long handle);
private native boolean setCopyMetadataNative(long handle, boolean value);
private native double getImageQualityNative(long handle);
private native boolean setImageQualityNative(long handle, double value);
}