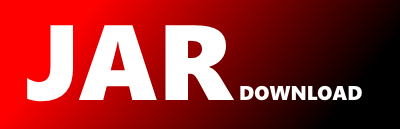
com.pdftools.pdfa.validation.AnalysisOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
The newest version!
/****************************************************************************
*
* File: AnalysisOptions.java
*
* Description: PDFTOOLS AnalysisOptions Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.pdfa.validation;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The PDF/A analysis options
* Options for the analysis of documents using the validator's method {@link Validator#analyze } in preparation for the document's conversion to PDF/A.
*/
public class AnalysisOptions extends NativeObject
{
protected AnalysisOptions(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static AnalysisOptions createDynamicObject(long handle)
{
return new AnalysisOptions(handle);
}
/**
*
*/
public AnalysisOptions()
{
this(newHelper());
}
private static long newHelper()
{
long handle = newNative();
if (handle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return handle;
}
/**
* The PDF/A conformance to validate (Getter)
*
* It is recommended to use:
*
* -
* The input document's claimed conformance {@link com.pdftools.pdf.Document#getConformance pdftools.pdf.Document.getConformance}, if it is an acceptable conversion conformance.
* No conversion is needed, if the analysis result's property {@link AnalysisResult#getIsConversionRecommended } is {@code false}.
*
* -
* PDF/A-2b for the conversion to PDF/A-2. This is the recommended value for all other input documents.
*
* -
* PDF/A-3b for the conversion to PDF/A-3
* -
* PDF/A-1b for the conversion to PDF/A-1
*
*
* Default: "PDF/A-2b"
*
*/
public com.pdftools.pdf.Conformance getConformance()
{
int retVal = getConformanceNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return new com.pdftools.pdf.Conformance(retVal);
}
/**
* The PDF/A conformance to validate (Setter)
*
* It is recommended to use:
*
* -
* The input document's claimed conformance {@link com.pdftools.pdf.Document#getConformance pdftools.pdf.Document.getConformance}, if it is an acceptable conversion conformance.
* No conversion is needed, if the analysis result's property {@link AnalysisResult#getIsConversionRecommended } is {@code false}.
*
* -
* PDF/A-2b for the conversion to PDF/A-2. This is the recommended value for all other input documents.
*
* -
* PDF/A-3b for the conversion to PDF/A-3
* -
* PDF/A-1b for the conversion to PDF/A-1
*
*
* Default: "PDF/A-2b"
*
* @throws IllegalArgumentException if {@code value} is {@code null}
*/
public void setConformance(com.pdftools.pdf.Conformance value)
{
if (value == null)
throw new IllegalArgumentException("Argument 'value' must not be null.", new NullPointerException("'value'"));
boolean retVal = setConformanceNative(getHandle(), value.getValue());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Whether to enable additional, strict validation checks (Getter)
*
* Whether to check for potential issues that are corner cases of the PDF/A ISO Standard in which a conversion is strongly advised.
* Also see the documentation of {@link AnalysisResult#getIsConversionRecommended }.
*
* Default: {@code true}
*/
public boolean getStrictMode()
{
boolean retVal = getStrictModeNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether to enable additional, strict validation checks (Setter)
*
* Whether to check for potential issues that are corner cases of the PDF/A ISO Standard in which a conversion is strongly advised.
* Also see the documentation of {@link AnalysisResult#getIsConversionRecommended }.
*
* Default: {@code true}
*/
public void setStrictMode(boolean value)
{
boolean retVal = setStrictModeNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
private static native long newNative();
private native int getConformanceNative(long handle);
private native boolean setConformanceNative(long handle, int value);
private native boolean getStrictModeNative(long handle);
private native boolean setStrictModeNative(long handle, boolean value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy