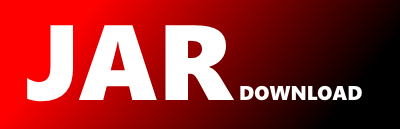
com.pdftools.pdfa.validation.AnalysisResult Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: AnalysisResult.java
*
* Description: PDFTOOLS AnalysisResult Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.pdfa.validation;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The PDF/A analysis result
*
* Result of the validator's method {@link Validator#analyze } which is required for the conversion to PDF/A with {@link com.pdftools.pdfa.conversion.Converter#convert pdftools.pdfa.conversion.Converter.convert}.
*
* Note that {@link AnalysisResult } objects remain valid as long as their {@link com.pdftools.pdf.Document pdftools.pdf.Document} has not been closed and the analysis result has not been used in {@link com.pdftools.pdfa.conversion.Converter#convert pdftools.pdfa.conversion.Converter.convert}.
*
*/
public class AnalysisResult extends NativeObject
{
protected AnalysisResult(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static AnalysisResult createDynamicObject(long handle)
{
return new AnalysisResult(handle);
}
/**
* The conformance used for analysis (Getter)
*
* The PDF/A level might differ from the {@link AnalysisOptions#getConformance }.
* If the claimed PDF/A level of the input document is higher than {@link AnalysisOptions#getConformance }, the higher level is used for {@link AnalysisResult#getConformance }.
*
* For example, if {@link AnalysisOptions#getConformance } is PDF/A-2b, but the document's claimed conformance is PDF/A-2u, the analysis checks if the document actually conforms to its claimed conformance PDF/A-2u.
* Because otherwise a conversion is required.
*
*/
public com.pdftools.pdf.Conformance getConformance()
{
int retVal = getConformanceNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return new com.pdftools.pdf.Conformance(retVal);
}
/**
* The recommended conversion conformance (Getter)
* The optimal PDF/A conformance for the conversion (i.e. the {@link com.pdftools.pdfa.conversion.ConversionOptions#getConformance pdftools.pdfa.conversion.ConversionOptions.getConformance}).
* The recommended conformance level might be higher than the analysis conformance, if the document actually contains all data required for the higher level.
* It might also be lower, if the document is missing some required data.
*/
public com.pdftools.pdf.Conformance getRecommendedConformance()
{
int retVal = getRecommendedConformanceNative(getHandle());
if (retVal == 0)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return new com.pdftools.pdf.Conformance(retVal);
}
/**
* Whether the document should be converted to PDF/A (Getter)
*
* A conversion is generally recommended in the following cases:
*
* -
* If {@link AnalysisResult#getIsConforming } is {@code false}, i.e. if the document does not conform to the {@link AnalysisResult#getConformance }.
*
* -
* If the document is conforming, but other issues are found for which a conversion is highly recommended.
* For example, if certain corner cases of the specification are detected.
*
*
*
* Note that in certain processes it might also be beneficial to convert a document if its conformance does not match the {@link AnalysisResult#getRecommendedConformance }.
* This will actually upgrade the PDF/A level of the input document.
*
*/
public boolean getIsConversionRecommended()
{
boolean retVal = getIsConversionRecommendedNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether the document is conforming (Getter)
* Whether the document conforms to the {@link AnalysisResult#getConformance }.
* Note that even if this property returns {@code true} a conversion might still be recommended as indicated by {@link AnalysisResult#getIsConversionRecommended }.
*/
public boolean getIsConforming()
{
boolean retVal = getIsConformingNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether the document is digitally signed (Getter)
*
*/
public boolean getIsSigned()
{
boolean retVal = getIsSignedNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* Whether the document contains embedded files (Getter)
*
*/
public boolean getHasEmbeddedFiles()
{
boolean retVal = getHasEmbeddedFilesNative(getHandle());
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The number of fonts used in the document (Getter)
*
*/
public int getFontCount()
{
int retVal = getFontCountNative(getHandle());
if (retVal == -1)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
private native int getConformanceNative(long handle);
private native int getRecommendedConformanceNative(long handle);
private native boolean getIsConversionRecommendedNative(long handle);
private native boolean getIsConformingNative(long handle);
private native boolean getIsSignedNative(long handle);
private native boolean getHasEmbeddedFilesNative(long handle);
private native int getFontCountNative(long handle);
}