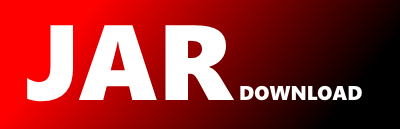
com.pdftools.pdfa.validation.ErrorCategory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
The newest version!
/****************************************************************************
*
* File: ErrorCategory.java
*
* Description: ErrorCategory Enumeration
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.pdfa.validation;
/**
* The validation error category
*/
public enum ErrorCategory
{
/**
*
* The file format (header, trailer, objects, xref, streams) is corrupted.
*/
FORMAT(0x00000001),
/**
*
* The document doesn't conform to the PDF reference or PDF/A Specification (missing required entries, wrong value types, etc.).
*/
PDF(0x00000002),
/**
*
* The file is encrypted.
*/
ENCRYPTION(0x00000004),
/**
*
* The document contains device-specific color spaces.
*/
COLOR(0x00000008),
/**
*
* The document contains illegal rendering hints (unknown intents, interpolation, transfer and halftone functions).
*/
RENDERING(0x00000010),
/**
*
* The document contains alternate information (images).
*/
ALTERNATE(0x00000020),
/**
*
* The document contains embedded PostScript code.
*/
POST_SCRIPT(0x00000040),
/**
*
* The document contains references to external content (reference XObjects, OPI).
*/
EXTERNAL(0x00000080),
/**
*
* The document contains fonts without embedded font programs or encoding information (CMAPs)
*/
FONT(0x00000100),
/**
*
* The document contains fonts without appropriate character to Unicode mapping information (ToUnicode maps)
*/
UNICODE(0x00000200),
/**
*
* The document contains transparency.
*/
TRANSPARENCY(0x00000400),
/**
*
* The document contains unknown annotation types.
*/
UNSUPPORTED_ANNOTATION(0x00000800),
/**
*
* The document contains multimedia annotations (sound, movies).
*/
MULTIMEDIA(0x00001000),
/**
*
* The document contains hidden, invisible, non-viewable or non-printable annotations.
*/
PRINT(0x00002000),
/**
*
* The document contains annotations or form fields with ambiguous or without appropriate appearances.
*/
APPEARANCE(0x00004000),
/**
*
* The document contains actions types other than for navigation (launch, JavaScript, ResetForm, etc.)
*/
ACTION(0x00008000),
/**
*
* The document's meta data is either missing or inconsistent or corrupt.
*/
METADATA(0x00010000),
/**
*
* The document doesn't provide appropriate logical structure information.
*/
STRUCTURE(0x00020000),
/**
*
* The document contains optional content (layers).
*/
OPTIONAL_CONTENT(0x00040000),
/**
*
* The document contains embedded files.
*/
EMBEDDED_FILE(0x00080000),
/**
*
* The document contains signatures.
*/
SIGNATURE(0x00100000),
/**
*
* Violations of custom corporate directives.
*/
CUSTOM(0x40000000);
ErrorCategory(int value)
{
this.value = value;
}
/**
* @hidden
*/
public static ErrorCategory fromValue(int value)
{
switch (value)
{
case 0x00000001: return FORMAT;
case 0x00000002: return PDF;
case 0x00000004: return ENCRYPTION;
case 0x00000008: return COLOR;
case 0x00000010: return RENDERING;
case 0x00000020: return ALTERNATE;
case 0x00000040: return POST_SCRIPT;
case 0x00000080: return EXTERNAL;
case 0x00000100: return FONT;
case 0x00000200: return UNICODE;
case 0x00000400: return TRANSPARENCY;
case 0x00000800: return UNSUPPORTED_ANNOTATION;
case 0x00001000: return MULTIMEDIA;
case 0x00002000: return PRINT;
case 0x00004000: return APPEARANCE;
case 0x00008000: return ACTION;
case 0x00010000: return METADATA;
case 0x00020000: return STRUCTURE;
case 0x00040000: return OPTIONAL_CONTENT;
case 0x00080000: return EMBEDDED_FILE;
case 0x00100000: return SIGNATURE;
case 0x40000000: return CUSTOM;
}
throw new IllegalArgumentException("Unknown value for ErrorCategory: " + value);
}
/**
* @hidden
*/
public int getValue()
{
return value;
}
private int value;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy