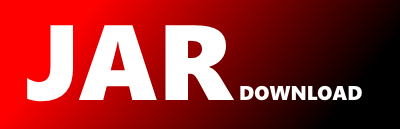
com.pdftools.sign.Appearance Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: Appearance.java
*
* Description: PDFTOOLS Appearance Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.sign;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* The visual appearance of signatures
*
* A signature may have a visual appearance on a page of the document.
* The visual appearance is optional and has no effect on the validity of the signature.
* Because of this and because a visual appearance may cover important content of the page,
* it is recommended to create invisible signatures by default.
*
* Typically, a visual appearance is created for forms with a dedicated area reserved for the appearance.
* Other transaction documents, e.g. invoices, correspondence, or bank statements, are usually signed without a visual appearance.
*
* The appearance can be positioned on a page using {@link Appearance#getPageNumber }, {@link Appearance#getTop }, {@link Appearance#getRight },
* {@link Appearance#getBottom }, and {@link Appearance#getLeft }.
* It is recommended to set either {@link Appearance#getTop } or {@link Appearance#getBottom } and {@link Appearance#getRight } or {@link Appearance#getLeft }.
* If all are {@code null}, the default is to position the appearance in the lower right corner with {@code 12 pt}
* ({@code 1/6 inch} or {@code 4.2 mm}) distance to the bottom and right edge of the page,
* i.e. {@code Bottom = 12} and {@code Right = 12}.
*
*/
public class Appearance extends NativeObject
{
protected Appearance(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static Appearance createDynamicObject(long handle)
{
return new Appearance(handle);
}
/**
* Create an appearance with the content loaded from a JSON file
* The format of the JSON file is described in the user manual.
* @param stream
* The JSON file defining the content
* @return
*
* @throws com.pdftools.CorruptException The file is not a valid JSON file.
* @throws com.pdftools.NotFoundException An image or font referenced in the JSON was not found.
* @throws com.pdftools.GenericException The JSON file is not a valid appearance content specification.
* @throws com.pdftools.ProcessingException Could not process content of the JSON.
* @throws IllegalArgumentException if {@code stream} is {@code null}
*/
public static com.pdftools.sign.Appearance createFromJson(com.pdftools.sys.Stream stream)
throws
com.pdftools.NotFoundException,
com.pdftools.GenericException,
com.pdftools.CorruptException,
com.pdftools.ProcessingException
{
if (stream == null)
throw new IllegalArgumentException("Argument 'stream' must not be null.", new NullPointerException("'stream'"));
long retHandle = createFromJsonNative(stream);
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 5: throw new com.pdftools.NotFoundException(getLastErrorMessage());
case 10: throw new com.pdftools.GenericException(getLastErrorMessage());
case 16: throw new com.pdftools.CorruptException(getLastErrorMessage());
case 21: throw new com.pdftools.ProcessingException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return com.pdftools.sign.Appearance.createDynamicObject(retHandle);
}
/**
* Create an appearance with the content loaded from an XML file
* The format of the XML file is described in the user manual.
* @param stream
* The XML file defining the content
* @return
*
* @throws com.pdftools.CorruptException The file is not a valid XML file.
* @throws com.pdftools.NotFoundException An image or font referenced in the XML was not found.
* @throws com.pdftools.GenericException The XML file is not a valid appearance content specification.
* @throws com.pdftools.ProcessingException Could not process content of the XML.
* @throws IllegalArgumentException if {@code stream} is {@code null}
*/
public static com.pdftools.sign.Appearance createFromXml(com.pdftools.sys.Stream stream)
throws
com.pdftools.NotFoundException,
com.pdftools.GenericException,
com.pdftools.CorruptException,
com.pdftools.ProcessingException
{
if (stream == null)
throw new IllegalArgumentException("Argument 'stream' must not be null.", new NullPointerException("'stream'"));
long retHandle = createFromXmlNative(stream);
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 5: throw new com.pdftools.NotFoundException(getLastErrorMessage());
case 10: throw new com.pdftools.GenericException(getLastErrorMessage());
case 16: throw new com.pdftools.CorruptException(getLastErrorMessage());
case 21: throw new com.pdftools.ProcessingException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return com.pdftools.sign.Appearance.createDynamicObject(retHandle);
}
/**
* Create the bounding box for an unsigned signature field
* Unsigned signature fields can define a rectangle on a page.
* When the field is signed, the signer creates a visual appearance within that rectangle.
* @param size
* The size of the rectangle
* @return
* @throws IllegalArgumentException if {@code size} is {@code null}
*/
public static com.pdftools.sign.Appearance createFieldBoundingBox(com.pdftools.geometry.units.Size size)
{
if (size == null)
throw new IllegalArgumentException("Argument 'size' must not be null.", new NullPointerException("'size'"));
long retHandle = createFieldBoundingBoxNative(size.getWidthValue(), size.getHeightValue());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.sign.Appearance.createDynamicObject(retHandle);
}
/**
* The number of the page where the appearance is positioned (Getter)
*
* Page number must be in the range from {@code 1} to {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount}.
*
* If {@code null}, the appearance is positioned on the last page.
*
* Default: {@code null}
*/
public Integer getPageNumber()
{
Integer retVal = getPageNumberNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The number of the page where the appearance is positioned (Setter)
*
* Page number must be in the range from {@code 1} to {@link com.pdftools.pdf.Document#getPageCount pdftools.pdf.Document.getPageCount}.
*
* If {@code null}, the appearance is positioned on the last page.
*
* Default: {@code null}
*/
public void setPageNumber(Integer value)
{
boolean retVal = setPageNumberNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
}
/**
* Distance to top of page (Getter)
*
* This property specifies the distance between appearance's top edge and the top of the page.
*
* If {@code null}, the distance to the top is unspecified.
*
* Default: {@code null}
*/
public com.pdftools.geometry.units.Length getTop()
{
Double retVal = getTopNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
return null;
}
return new com.pdftools.geometry.units.Length(retVal);
}
/**
* Distance to top of page (Setter)
*
* This property specifies the distance between appearance's top edge and the top of the page.
*
* If {@code null}, the distance to the top is unspecified.
*
* Default: {@code null}
*
* @throws IllegalArgumentException If the given value is negative
*/
public void setTop(com.pdftools.geometry.units.Length value)
{
boolean retVal = setTopNative(getHandle(), value != null ? value.getValue() : null);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* Distance to right of page (Getter)
*
* This property specifies the distance between appearance's right edge and the right of the page.
*
* If {@code null}, the distance to the right is unspecified.
*
* Default: {@code null}
*/
public com.pdftools.geometry.units.Length getRight()
{
Double retVal = getRightNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
return null;
}
return new com.pdftools.geometry.units.Length(retVal);
}
/**
* Distance to right of page (Setter)
*
* This property specifies the distance between appearance's right edge and the right of the page.
*
* If {@code null}, the distance to the right is unspecified.
*
* Default: {@code null}
*
* @throws IllegalArgumentException If the given value is negative
*/
public void setRight(com.pdftools.geometry.units.Length value)
{
boolean retVal = setRightNative(getHandle(), value != null ? value.getValue() : null);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* Distance to bottom of page (Getter)
*
* This property specifies the distance between appearance's bottom edge and the bottom of the page.
*
* If {@code null}, the distance to the bottom is unspecified.
*
* Default: {@code null}
*/
public com.pdftools.geometry.units.Length getBottom()
{
Double retVal = getBottomNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
return null;
}
return new com.pdftools.geometry.units.Length(retVal);
}
/**
* Distance to bottom of page (Setter)
*
* This property specifies the distance between appearance's bottom edge and the bottom of the page.
*
* If {@code null}, the distance to the bottom is unspecified.
*
* Default: {@code null}
*
* @throws IllegalArgumentException If the given value is negative
*/
public void setBottom(com.pdftools.geometry.units.Length value)
{
boolean retVal = setBottomNative(getHandle(), value != null ? value.getValue() : null);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* Distance to left of page (Getter)
*
* This property specifies the distance between appearance's left edge and the left of the page.
*
* If {@code null}, the distance to the left is unspecified.
*
* Default: {@code null}
*/
public com.pdftools.geometry.units.Length getLeft()
{
Double retVal = getLeftNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
default: throwLastRuntimeException();
}
return null;
}
return new com.pdftools.geometry.units.Length(retVal);
}
/**
* Distance to left of page (Setter)
*
* This property specifies the distance between appearance's left edge and the left of the page.
*
* If {@code null}, the distance to the left is unspecified.
*
* Default: {@code null}
*
* @throws IllegalArgumentException If the given value is negative
*/
public void setLeft(com.pdftools.geometry.units.Length value)
{
boolean retVal = setLeftNative(getHandle(), value != null ? value.getValue() : null);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 3: throw new IllegalArgumentException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* Maps the name of a custom text variable to its value.
* These variables can parametrize the content of the text element in the appearance configuration XML and Json files.
* They are used by setting "[custom:‹key›]".
*/
public com.pdftools.sign.CustomTextVariableMap getCustomTextVariables()
{
long retHandle = getCustomTextVariablesNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
default: throwLastRuntimeException();
}
}
return com.pdftools.sign.CustomTextVariableMap.createDynamicObject(retHandle);
}
private static native long createFromJsonNative(com.pdftools.sys.Stream stream);
private static native long createFromXmlNative(com.pdftools.sys.Stream stream);
private static native long createFieldBoundingBoxNative(double sizeWidth, double sizeHeight);
private native Integer getPageNumberNative(long handle);
private native boolean setPageNumberNative(long handle, Integer value);
private native Double getTopNative(long handle);
private native boolean setTopNative(long handle, Double value);
private native Double getRightNative(long handle);
private native boolean setRightNative(long handle, Double value);
private native Double getBottomNative(long handle);
private native boolean setBottomNative(long handle, Double value);
private native Double getLeftNative(long handle);
private native boolean setLeftNative(long handle, Double value);
private native long getCustomTextVariablesNative(long handle);
}