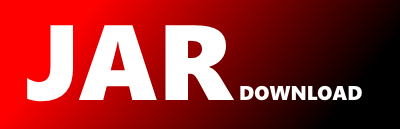
com.pdftools.sign.MapCommon Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdftools-sdk Show documentation
Show all versions of pdftools-sdk Show documentation
The Pdftools SDK is a comprehensive development library that lets developers integrate advanced PDF functionalities into in-house applications.
The newest version!
/****************************************************************************
*
* File: MapCommon.java
*
* Description: PDFTOOLS internal classes of map implementations.
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.sign;
import java.util.AbstractCollection;
import java.util.AbstractSet;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
/**
* @hidden
* In Java, the file name should be always the same as a class name.
* This dummy class is to fulfill that requirement because some tools, e.g. javadoc, cannot process the file otherwise.
*/
class MapCommon
{}
class KeySet extends AbstractSet
{
public KeySet(Map map)
{
this.map = map;
}
@Override
public boolean remove(Object o)
{
return map.remove(o) != null;
}
@Override
public void clear()
{
map.clear();
}
@Override
public boolean contains(Object object)
{
return map.containsKey(object);
}
@Override
public Iterator iterator()
{
return new KeyIterator(map.entrySet().iterator());
}
@Override
public int size()
{
return map.size();
}
private Map map;
}
class ValueCollection extends AbstractCollection
{
public ValueCollection(Map map)
{
this.map = map;
}
@Override
public Iterator iterator()
{
return new ValueIterator(map.entrySet().iterator());
}
@Override
public int size()
{
return map.size();
}
private Map map;
}
abstract class ProxyIterator implements Iterator
{
public ProxyIterator(Iterator it)
{
this.it = it;
}
@Override
public boolean hasNext() {
return it.hasNext();
}
@Override
public void remove()
{
it.remove();
}
Iterator
it;
}
class KeyIterator extends ProxyIterator>
{
public KeyIterator(Iterator> it)
{
super(it);
}
@Override
public K next()
{
return it.next().getKey();
}
}
class ValueIterator extends ProxyIterator>
{
public ValueIterator(Iterator> it)
{
super(it);
}
@Override
public V next()
{
return it.next().getValue();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy