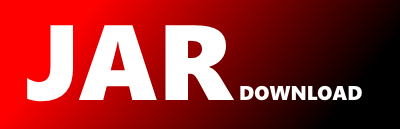
com.pdftools.sign.SignatureConfiguration Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: SignatureConfiguration.java
*
* Description: PDFTOOLS SignatureConfiguration Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.sign;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* Configuration for signature creation
*
* This configuration controls the signature creation in {@link Signer#sign } and {@link Signer#certify }.
*
* Use a {@link com.pdftools.crypto.providers.Provider pdftools.crypto.providers.Provider} to create a signature configuration.
*
* Note that this object can be re-used to sign multiple documents for mass-signing applications.
*
*/
public class SignatureConfiguration extends NativeObject
{
protected SignatureConfiguration(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static SignatureConfiguration createDynamicObject(long handle)
{
int type = getType(handle);
switch (type)
{
case 0:
return new SignatureConfiguration(handle);
case 1:
return com.pdftools.crypto.providers.globalsigndss.SignatureConfiguration.createDynamicObject(handle);
case 2:
return com.pdftools.crypto.providers.swisscomsigsrv.SignatureConfiguration.createDynamicObject(handle);
case 3:
return com.pdftools.crypto.providers.pkcs11.SignatureConfiguration.createDynamicObject(handle);
case 4:
return com.pdftools.crypto.providers.builtin.SignatureConfiguration.createDynamicObject(handle);
default:
return null;
}
}
/**
* The name of the existing signature field (Getter)
*
* The {@link com.pdftools.pdf.SignatureField#getFieldName pdftools.pdf.SignatureField.getFieldName} of an existing, unsigned signature field to sign.
* Note that when an existing signature field is signed, the appearance's position is defined by the existing field.
* Therefore, make sure the {@link SignatureConfiguration#getAppearance } fits into the {@link com.pdftools.pdf.SignatureField#getBoundingBox pdftools.pdf.SignatureField.getBoundingBox}.
*
* If {@code null} a new signature field is created using a unique field name.
*
* Default: {@code null}
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public String getFieldName()
{
String retVal = getFieldNameNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The name of the existing signature field (Setter)
*
* The {@link com.pdftools.pdf.SignatureField#getFieldName pdftools.pdf.SignatureField.getFieldName} of an existing, unsigned signature field to sign.
* Note that when an existing signature field is signed, the appearance's position is defined by the existing field.
* Therefore, make sure the {@link SignatureConfiguration#getAppearance } fits into the {@link com.pdftools.pdf.SignatureField#getBoundingBox pdftools.pdf.SignatureField.getBoundingBox}.
*
* If {@code null} a new signature field is created using a unique field name.
*
* Default: {@code null}
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public void setFieldName(String value)
{
boolean retVal = setFieldNameNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The visual appearance of the signature (Getter)
*
* The visual appearance or {@code null} to create a signature without a visual appearance.
*
* Default: {@code null}
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public com.pdftools.sign.Appearance getAppearance()
{
long retHandle = getAppearanceNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
return null;
}
return com.pdftools.sign.Appearance.createDynamicObject(retHandle);
}
/**
* The visual appearance of the signature (Setter)
*
* The visual appearance or {@code null} to create a signature without a visual appearance.
*
* Default: {@code null}
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public void setAppearance(com.pdftools.sign.Appearance value)
{
boolean retVal = setAppearanceNative(getHandle(), getHandle(value), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The name of the signing certificate (Getter)
*
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public String getName()
{
String retVal = getNameNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The location of signing (Getter)
* The CPU host name or physical location of the signing.
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public String getLocation()
{
String retVal = getLocationNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The location of signing (Setter)
* The CPU host name or physical location of the signing.
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public void setLocation(String value)
{
boolean retVal = setLocationNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The reason for signing (Getter)
*
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public String getReason()
{
String retVal = getReasonNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The reason for signing (Setter)
*
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public void setReason(String value)
{
boolean retVal = setReasonNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The contact information of the signer (Getter)
* Information provided by the signer to enable a recipient to contact
* the signer to verify the signature.
* For example, a phone number.
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public String getContactInfo()
{
String retVal = getContactInfoNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The contact information of the signer (Setter)
* Information provided by the signer to enable a recipient to contact
* the signer to verify the signature.
* For example, a phone number.
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public void setContactInfo(String value)
{
boolean retVal = setContactInfoNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
private static native int getType(long handle);
private native String getFieldNameNative(long handle);
private native boolean setFieldNameNative(long handle, String value);
private native long getAppearanceNative(long handle);
private native boolean setAppearanceNative(long handle, long value, com.pdftools.sign.Appearance valueObj);
private native String getNameNative(long handle);
private native String getLocationNative(long handle);
private native boolean setLocationNative(long handle, String value);
private native String getReasonNative(long handle);
private native boolean setReasonNative(long handle, String value);
private native String getContactInfoNative(long handle);
private native boolean setContactInfoNative(long handle, String value);
}