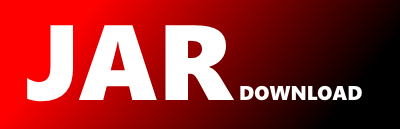
com.pdftools.sign.TimestampConfiguration Maven / Gradle / Ivy
Show all versions of pdftools-sdk Show documentation
/****************************************************************************
*
* File: TimestampConfiguration.java
*
* Description: PDFTOOLS TimestampConfiguration Class
*
* Author: PDF Tools AG
*
* Copyright: Copyright (C) 2023 - 2024 PDF Tools AG, Switzerland
* All rights reserved.
*
* Notice: By downloading and using this artifact, you accept PDF Tools AG's
* [license agreement](https://www.pdf-tools.com/license-agreement/),
* [privacy policy](https://www.pdf-tools.com/privacy-policy/),
* and allow PDF Tools AG to track your usage data.
*
***************************************************************************/
package com.pdftools.sign;
import com.pdftools.sys.*;
import com.pdftools.internal.*;
import java.util.EnumSet;
import java.time.OffsetDateTime;
/**
* Configuration for adding time-stamps
*
* This configuration controls the creation of time-stamps in {@link Signer#addTimestamp }.
*
* Use a {@link com.pdftools.crypto.providers.Provider pdftools.crypto.providers.Provider} to create a time-stamp configuration.
*
* Note that this object can be re-used to add time-stamps to multiple documents for mass-signing applications.
*
*/
public abstract class TimestampConfiguration extends NativeObject
{
protected TimestampConfiguration(long handle)
{
super(handle);
}
/**
* @hidden
*/
public static TimestampConfiguration createDynamicObject(long handle)
{
int type = getType(handle);
switch (type)
{
case 1:
return com.pdftools.crypto.providers.globalsigndss.TimestampConfiguration.createDynamicObject(handle);
case 2:
return com.pdftools.crypto.providers.swisscomsigsrv.TimestampConfiguration.createDynamicObject(handle);
case 3:
return com.pdftools.crypto.providers.pkcs11.TimestampConfiguration.createDynamicObject(handle);
case 4:
return com.pdftools.crypto.providers.builtin.TimestampConfiguration.createDynamicObject(handle);
default:
return null;
}
}
/**
* The name of the existing signature field (Getter)
*
* The {@link com.pdftools.pdf.SignatureField#getFieldName pdftools.pdf.SignatureField.getFieldName} of an existing, unsigned signature field to time-stamp.
* Note that when an existing signature field is used, the appearance's position is defined by the existing field.
* Therefore, make sure the {@link TimestampConfiguration#getAppearance } fits into the {@link com.pdftools.pdf.SignatureField#getBoundingBox pdftools.pdf.SignatureField.getBoundingBox}.
*
* If {@code null}, a new signature field is created using a unique field name.
*
* Default: {@code null}
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public String getFieldName()
{
String retVal = getFieldNameNative(getHandle());
if (retVal == null)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
return retVal;
}
/**
* The name of the existing signature field (Setter)
*
* The {@link com.pdftools.pdf.SignatureField#getFieldName pdftools.pdf.SignatureField.getFieldName} of an existing, unsigned signature field to time-stamp.
* Note that when an existing signature field is used, the appearance's position is defined by the existing field.
* Therefore, make sure the {@link TimestampConfiguration#getAppearance } fits into the {@link com.pdftools.pdf.SignatureField#getBoundingBox pdftools.pdf.SignatureField.getBoundingBox}.
*
* If {@code null}, a new signature field is created using a unique field name.
*
* Default: {@code null}
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public void setFieldName(String value)
{
boolean retVal = setFieldNameNative(getHandle(), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
/**
* The visual appearance of the time-stamp (Getter)
*
* The visual appearance or {@code null} to create a time-stamp without a visual appearance.
*
* For time-stamps, not all text variables are available,
* most notably the {@code [signature:name]}.
*
* Default: {@code null}
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public com.pdftools.sign.Appearance getAppearance()
{
long retHandle = getAppearanceNative(getHandle());
if (retHandle == 0)
{
switch (getLastErrorCode())
{
case 0: break;
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
return null;
}
return com.pdftools.sign.Appearance.createDynamicObject(retHandle);
}
/**
* The visual appearance of the time-stamp (Setter)
*
* The visual appearance or {@code null} to create a time-stamp without a visual appearance.
*
* For time-stamps, not all text variables are available,
* most notably the {@code [signature:name]}.
*
* Default: {@code null}
*
* @throws IllegalStateException If the creating provider has already been closed
*/
public void setAppearance(com.pdftools.sign.Appearance value)
{
boolean retVal = setAppearanceNative(getHandle(), getHandle(value), value);
if (!retVal)
{
switch (getLastErrorCode())
{
case 0: throw new RuntimeException("An unexpected error occurred");
case 2: throw new IllegalStateException(getLastErrorMessage());
default: throwLastRuntimeException();
}
}
}
private static native int getType(long handle);
private native String getFieldNameNative(long handle);
private native boolean setFieldNameNative(long handle, String value);
private native long getAppearanceNative(long handle);
private native boolean setAppearanceNative(long handle, long value, com.pdftools.sign.Appearance valueObj);
}